How to Use Angular 2 Hover Event
-
the
mouseenter
andmouseleave
Applications in Angular -
the
mouseover
andmouseout
Applications in Angular -
Pass the
mouseenter
andmouseleave
as a Function in Angular
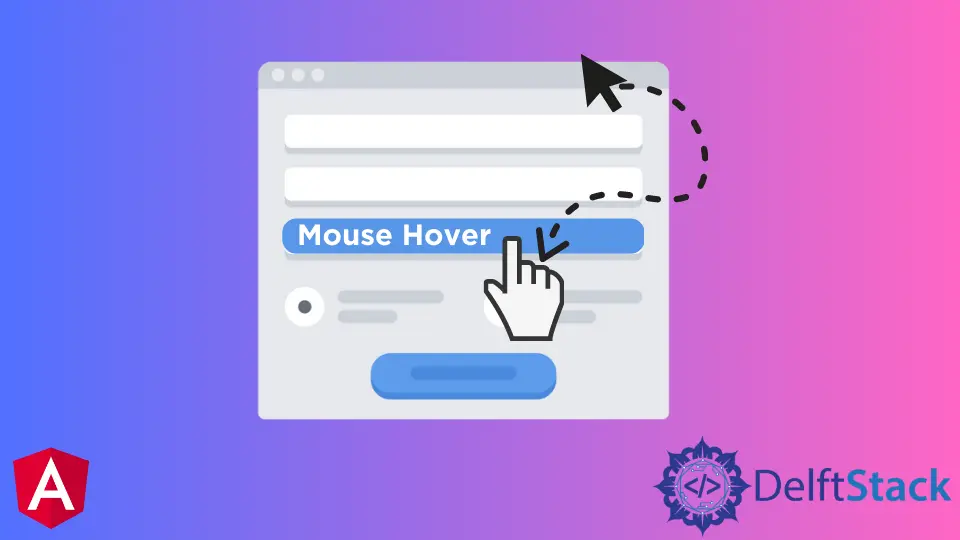
When you hover your mouse on certain elements on the screen, you get access to information without clicking. You can even perform some functions on the element you hover your mouse.
This article looks at simple solutions to carry out the hover event function mouse hover on elements.
We will apply the mouseenter
and mouseleave
functions to create a hover event. The second method will also use two functions, namely the mouseover
and mouseout
.
We will then apply a more advanced approach to carry out a hover event.
the mouseenter
and mouseleave
Applications in Angular
When dealing with functions that come in pairs, we need to pass entries for each function.
We use the mouseenter
function to declare what happens when we hover a mouse over the element. The mouseleave
function then determines what happens when moving the mouse away.
The HTML
input will look this:
<div style="text-align: center;">
<h2>Mouse Hover Event</h2>
</div>
<button
class="btn-primary btn"
(mouseenter)="showImage = true"
(mouseleave)="showImage = false"
>
Hover Over Me!
</button>
<br />
<img
*ngIf="showImage"
src="https://vangogh.teespring.com/v3/image/so-OI-9L5KAWsqHQuv6gQwymSQ8/480/560.jpg"
/>
Afterward, we will write the app.component.ts
like below:
import { Component, VERSION } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
name = 'Angular ' + VERSION.major;
title = 'mouse-hover';
showImage: boolean;
constructor() {
this.showImage = false;
}
}
the mouseover
and mouseout
Applications in Angular
The mouseover
and mouseout
attribute work in pairs like the method explained earlier, we only need to switch the functions and voila:
<div style="text-align: center;">
<h2>Mouse Hover Event</h2>
</div>
<button
class="btn-primary btn"
(mouseover)="showImage = true"
(mouseout)="showImage = false"
>
Hover Over Me!
</button>
<br />
<img
*ngIf="showImage"
src="https://vangogh.teespring.com/v3/image/so-OI-9L5KAWsqHQuv6gQwymSQ8/480/560.jpg"
/>
Pass the mouseenter
and mouseleave
as a Function in Angular
We will get a bit more creative and sophisticated with the following method, where we will pass the mouseenter
and mouseleave
events as a function.
The HTML
will be slightly tweaked:
<div style="text-align: center;">
<h2>Mouse Hover Event</h2>
</div>
<button
class="btn-primary btn"
(mouseover)="showImg(true)"
(mouseleave)="showImg(false)"
>
Hover Over Me!
</button>
<br />
<img
*ngIf="showImage"
src="https://vangogh.teespring.com/v3/image/so-OI-9L5KAWsqHQuv6gQwymSQ8/480/560.jpg"
/>
And the app.component.ts
as well:
import { Component, VERSION } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
name = 'Angular ' + VERSION.major;
title = 'mouse-hover';
showImage: boolean;
constructor() {
this.showImage = false;
}
showImg(hover: boolean) {
this.showImage = hover;
}
}
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn