The if...else Statement in Angular
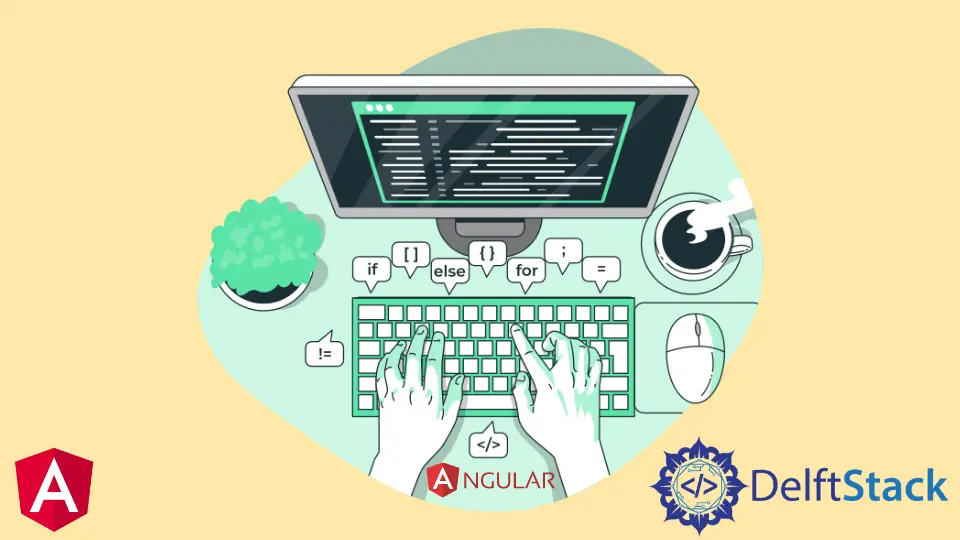
We will introduce how to use if
statements in angular applications and discuss examples.
Using the if
Statement in Angular
In programming, if
statements are logical blocks. These conditional statements tell the computer what to do when a particular condition is true or false.
In the modern era of web applications, if
statements make programmers’ lives easier to understand when to run a specific block of code based on the conditions.
There are different ways we can use if
statements in angular using *ng-if
or another simple method that we will discuss in an example.
Let’s create a new application by using the following command:
# angular
ng new my-app
After creating our new application in angular, we will go to our application directory using this command.
# angular
cd my-app
Now, let’s run our app to check if all dependencies are installed correctly.
# angular
ng serve --open
Then, in app.component.ts
, we will set a variable statement
as false
. We will use this variable to execute our code.
# angular
statement = false;
Now, in app.component.html
, we will create a template using the variable statement
that will display content saying the statement is true if we have set the variable to true
.
It will display the statement is false if we have set the variable to false
.
# angular
<hello name="{{ name }}"></hello>
<h2>{{ statement ? 'This statement is True' : 'This statement is false' }}</h2>
Let’s test our application to see if it works when changing the value statement
.
Output:
Change the value of the statement, set it to true
, and check how it works.
Output:
So, as you can see, when we change the value of the statement
variable, the code executes and shows the value we want to see using a simple method of statement.
Let’s imagine a block that we want to display inside a div where the if-else
statement is executed. We can use the *ng-if
statement and set the ids to the blocks we want to display when the condition is right or wrong.
We will set a new variable element
as 1. Our code in app.component.ts
will look like below.
# angular
import { Component, VERSION } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
statement = true;
element = 1;
}
Create a template in app.component.html
. We will have a div with a *ng-if
statement that will display a block trueBlock
, and if the value of element
is not 1, then it will display the block with an id of falseBlock
.
<div *ngIf="element == 1; then trueBlock; else falseBlock"></div>
<ng-template #trueBlock><button>Login</button></ng-template>
<ng-template #falseBlock><button>Logout</button></ng-template>
Let’s check how it works.
Output:
Try changing the value of the element
and check how it works.
# angular
element = 2;
Output:
As you can see in the above example, we can easily display the blocks of code using the *ng-if
statement and calling the block’s id.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn