How to Use forEach in Angular
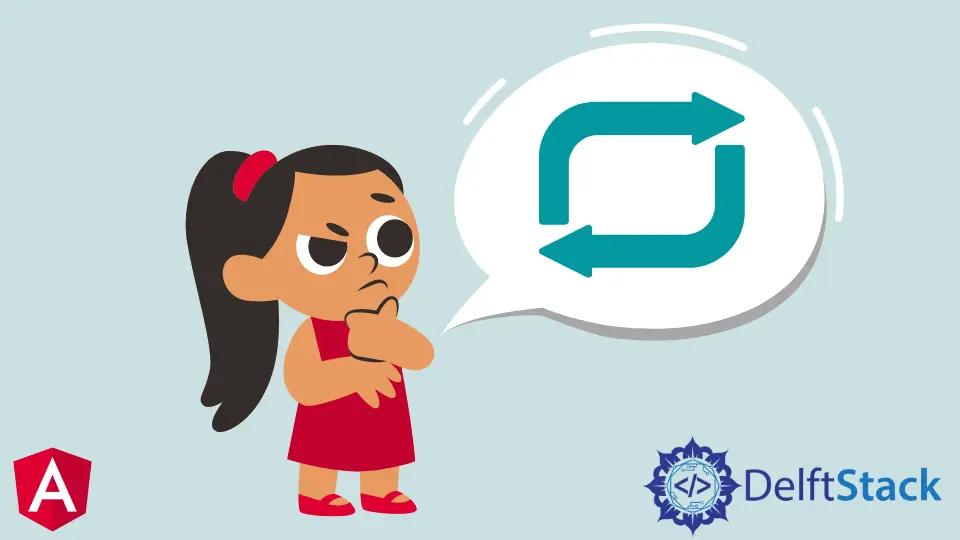
We will introduce the .forEach()
function in Angular with an example and use it to iterate through elements of an array.
Using .forEach()
in Angular
The .forEach()
is a function in Angular that calls a function for each element in an array.
It is not executed for empty arrays. It is used only in .ts
files and cannot be used in the template to display any information in template files in Angular.
The syntax of .forEach()
is very simple. Let’s start with an example by creating a new application using the following command.
# angular
ng new my-app
After creating our new application in Angular, we will go to our application directory using this command:
# angular
cd my-app
Now, let’s run our app to check if all dependencies are installed correctly.
# angular
ng serve --open
We will create an array of numbers from 1 to 10.
# Angular
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
Let’s use .forEach()
function to console.log
the array one by one. So, we will create another variable, num
.
# Angular
num = this.numbers.forEach(x => console.log(x));
Output:
As seen in the above example, we can use the .forEach()
to call a function on each element of an array. Let’s call a function on this array instead of just using console.log
.
In this function, we will take variable x
as a parameter that will be the elements of the array, and we will add 10
in them to return it.
# Angular
function(x){
x = x + 10;
console.log(x);
}
We will now use .forEach()
to call that function on each element of our array numbers
.
# Angular
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
num = this.numbers.forEach(x => this.function(x));
Output:
As seen in the above example, the function we passed to all elements of an array worked, and it added 10
into each element of an array. But we cannot use the .forEach()
function on template files to iterate through the elements of an array.
If we want to iterate through the elements of an array or a list on template files, we can use the *ngFor
loop.
Using the *ngFor
Loop in Angular
The *ngFor
is a for
loop in Angular that iterates through elements of an array, a list, or an object, and it can be used on template files to display a list of data on our web application or website.
We will go through an example in which we will create a list of widely used programming languages and display that list in a template file using the *ngFor
loop.
Let’s create a new application by using the following command.
# angular
ng new my-app
After creating our new application in Angular, we will go to our application directory using this command.
# angular
cd my-app
Let’s run our app to check if all dependencies are installed correctly.
# angular
ng serve --open
We will create a class file, language.ts
, in which we will create a constructor for a language. So code in language.ts
will look like below.
# angular
export class Language {
constructor(public name: string, public version: string) {}
}
In app.component.ts
, we will import Language
.
# angular
import { Language } from './language';
We will create a list of programming languages.
# angular
ProgrammingLanguages: Language[] = [
new Language('Angular', '13.1'),
new Language('React', '17'),
new Language('Vue', '3.2'),
new Language('Node', '17'),
];
In our template app.component.html
, we will display our list using the *ngFor
loop.
# angular
<main>
<h2>Widely Used Programming Languages</h2>
<section>
<div *ngFor="let language of ProgrammingLanguages">
<div class="list">
<h2>{{ language.name }}</h2>
<p>{{ language.version }}</p>
</div>
</div>
</section>
</main>
In app.component.css
, we will write some CSS to make our output look clean and easy to understand.
# angular
.list {
padding: 5px;
text-align: center;
background-color: white;
border: 1px solid black;
margin-bottom: 5px;
margin-left: 5px;
width: 45%;
float: left;
}
Output:
In this tutorial, we got to learn how we can use the .forEach()
function to iterate through elements of an array in typescript
files and how we can use the *ngFor
loop to iterate through elements of an array, object, or a list in template files and display data on the frontend of our web applications or websites.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn