ElementRef in Angular
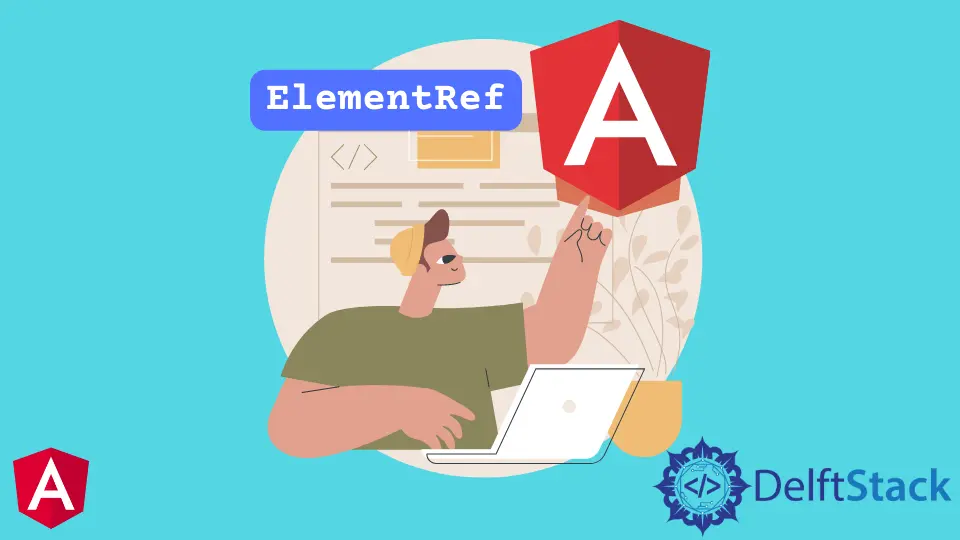
We will introduce what ElementRef
is and how to use it in Angular.
Use ElementRef
to Get DOM Element in Angular
ElemenRef
is a wrapper around a native DOM element object that contains the property nativeElement
. It holds the reference to the DOM element, and we can use it to manipulate the DOM. It is used with ViewChild
to get the HTML element from the component class.
Let’s go through an example to understand ElementRef
in detail and know how to use it in our application.
First, we need to import ViewChild
, ElementRef
, Component
, and AfterViewInit
from @angular/core
in the app.component.ts
file.
Once we have imported them, we will create an ElementRefDiv
using ViewChild
in our class. And we will create a ngAfterViewInit
function that will pass the value to our div.
So our code in app.component.ts
will look like below.
# angular
import { Component, ViewChild, ElementRef, AfterViewInit } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent implements AfterViewInit {
@ViewChild('ElementRefDiv') ElementView: ElementRef;
ngAfterViewInit() {
console.log(this.ElementView);
this.ElementView.nativeElement.innerHTML = 'Hello I am Using ElementRef To Display this!';
}
}
We need to create a template in app.component.html
with class ElementRefDiv
.
# angular
<div #ElementRefDiv></div>
Output:
So with these simple steps, we can manipulate any DOM element using its ElementRef
and ViewChild
.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn