How to Create a Simple Table in AngularJS
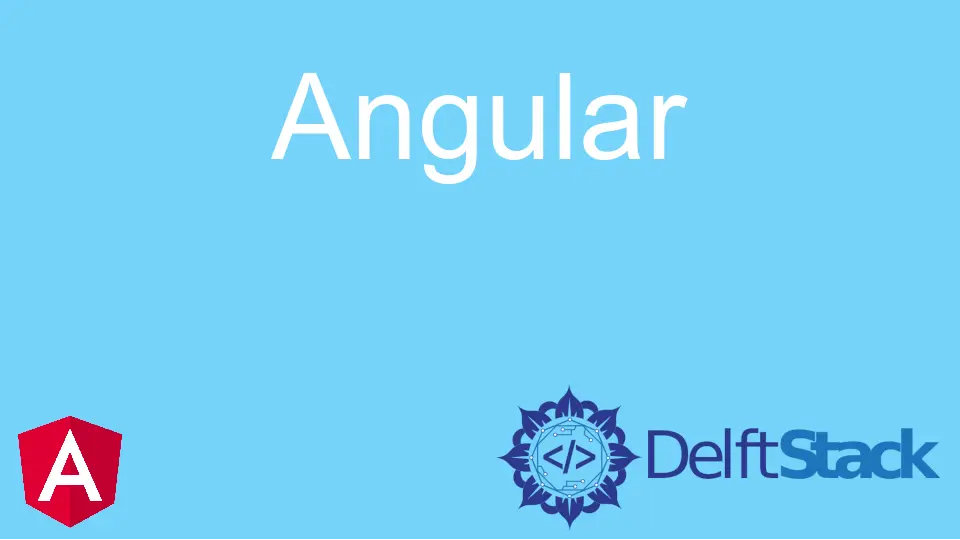
When we create web pages in an e-commerce website, we need to use a table to present large, complex information like the lists of products and their prices, list of staff, salaries, date of employment, etc.
Tables help us present these data in a well-structured and easy-to-digest way. It also allows us to manage the screen estate of our website better.
So we will create a simple table using the *ngFor
function, then pass it through a database. We will make use of the JSON Server Rest API
.
First, we will use VS Code editor, which allows us to use terminals from inside it to install dependencies. Then, we open the terminal and type in npm install -g json-server
and install the json server
to our system.
The next step is to create a new project.
After the project is created, we open the project folder and add a new file called the db.json
. This is where we store the data that the REST API
will access.
Now we navigate to the db.json
folder and input the data we want to have on the table.
{
"Users": [
{
"id": 1,
"firstName": "Nitin",
"lastName": "Rana",
"email": "nitin.rana@gmail.com",
"mobile": "2345678901",
"salary": "25000"
},
{
"id": 2,
"firstName": "Rajat",
"lastName": "Singh",
"email": "rajat.singh1@gmail.com",
"mobile": "5637189302",
"salary": "30000"
},
{
"id": 3,
"firstName": "Rahul",
"lastName": "Singh",
"email": "rahul.singh1@gmail.com",
"mobile": "5557189302",
"salary": "40000"
},
{
"id": 4,
"firstName": "Akhil",
"lastName": "Verma",
"email": "akhil.verma2@gmail.com",
"mobile": "5690889302",
"salary": "20000"
},
{
"id": 5,
"firstName": "Mohan",
"lastName": "Ram",
"email": "mohan.ram1@gmail.com",
"mobile": "7637189302",
"salary": "60000"
},
{
"id": 6,
"firstName": "Sohan",
"lastName": "Rana",
"email": "sohan.rana@gmail.com",
"mobile": "3425167890",
"salary": "25000"
},
{
"id": 7,
"firstName": "Rajjev",
"lastName": "Singh",
"email": "rajeev.singh1@gmail.com",
"mobile": "5637189302",
"salary": "30000"
},
{
"id": 8,
"firstName": "Mukul",
"lastName": "Singh",
"email": "mukul.singh1@gmail.com",
"mobile": "5557189302",
"salary": "40000"
},
{
"id": 9,
"firstName": "Vivek",
"lastName": "Verma",
"email": "vivek.verma2@gmail.com",
"mobile": "5690889302",
"salary": "20000"
},
{
"id": 10,
"firstName": "Shubham",
"lastName": "Singh",
"email": "shubham.singh@gmail.com",
"mobile": "7637189502",
"salary": "60000"
}
]
}
The next thing to do is navigate the app.component.html
to create the table using the *ngFor
function. But before that, we have to create a Rest service
that will access the data from db.json
.
Then we head to the terminal. In the project folder, we input ng generate service Rest
.
After installation, two files are created in the scr/app
folder, the rest.service.spec.ts
and the rest.service.ts
. We now need to navigate to the rest.service.ts
to do some coding:
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Users } from './Users';
@Injectable({
providedIn: 'root'
})
export class RestService {
constructor(private http : HttpClient) { }
url : string = "http://localhost:3000/Users";
getUsers()
{
return this.http.get<Users[]>(this.url);
}
}
This service helps monitor activities in the desired url where the database will be passing.
Then we head to the app.component.html
to create the table using the *ngFor
function:
<h1>Table using JSON Server API</h1>
<hr>
<table id="users">
<tr>
<th *ngFor="let col of columns">
{{col}}
</th>
</tr>
<tr *ngFor="let user of users">
<td *ngFor="let col of index">
{{user[col]}}
</td>
</tr>
</table>
The next thing that we need to do is head over to the app.module.ts
to do some more coding. Here we add the HttpClientModule
to the imports array.
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { AppComponent } from './app.component';
import { HttpClientModule } from '@angular/common/http';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
To make the table date in the db.json
readable by the HttpClient
, we must make it a string property. So we create a Users.ts
file, and we input these codes:
export class Users
{
id : string;
firstName : string;
lastName : string;
email : string;
mobile : string;
salary : string;
constructor(id, firstName, lastName, email, mobile, salary)
{
this.id = id;
this.firstName = firstName;
this.lastName = lastName;
this.email = email;
this.mobile = mobile;
this.salary = salary;
}
}
The only thing left to do at this point is to beautify the table using some CSS styling:
h1
{
text-align: center;
color: #4CAF50;
}
#users {
font-family: "Trebuchet MS", Arial, Helvetica, sans-serif;
border-collapse: collapse;
width: 100%;
}
#users td, #users th {
border: 1px solid #ddd;
padding: 8px;
}
#users tr:nth-child(even){background-color: #f2f2f2;}
#users tr:hover {background-color: #ddd;}
#users th {
padding-top: 12px;
padding-bottom: 12px;
text-align: left;
background-color: #4CAF50;
color: white;
}
Everything should work fine now, but we likely stumble into an error such as Parameter mobile
implicitly has an any
type.
We need to navigate to the ts.config.json
file and add noImplicitAny: false
to the list. Then we also add \\
to the noImplicitOverride: true,
to make the setting inactive.
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn