Component vs. Module in Angular
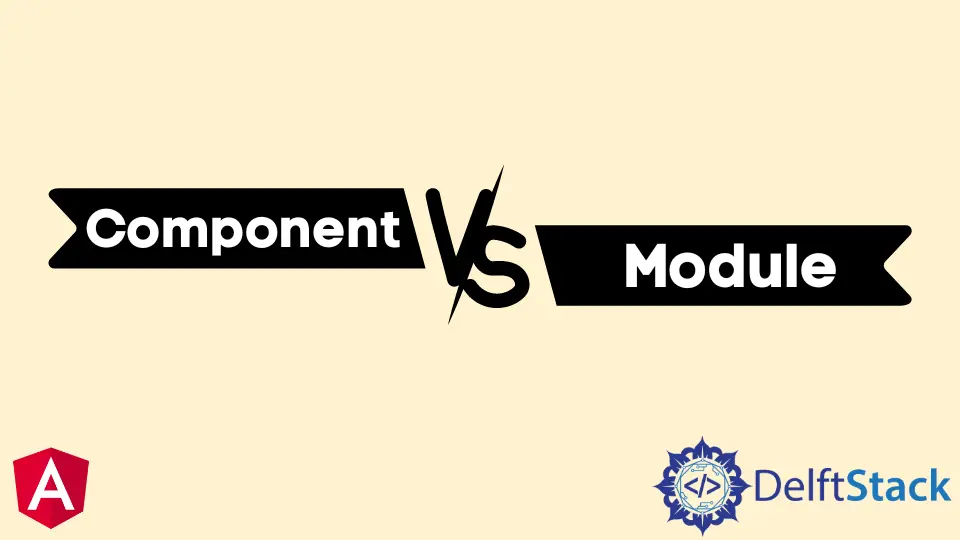
This article will introduce components and modules in Angular and discuss their differences.
Module in Angular
The module is a complete feature of our application.
For example, user authentication is a complete feature that includes user login, registration, and authentication (forget password and verify email). Thus, user authentication is a module of our application.
When we have a group of functionalities related to each other, just like user authentication, the module will contain one or more components, services, and pips.
For example, we have three components login
, register
, and forget a password
in user authentication. Our service will be an API call
in user authentication, and pips will be helpers.
So combining these different functionalities that are related to each other makes one module in our Angular application.
Now, let’s create a new application by using the following command.
# angular
ng new my-app
After creating our new application in Angular, we will go to our application directory using this command.
# angular
cd my-app
Now, let’s run our app to check if all dependencies are installed correctly.
# angular
ng serve --open
Then, we will open the app.module.ts
file.
# angular
import { BrowserModule } from "@angular/platform-browser";
import { NgModule } from "@angular/core";
import { AppComponent } from "./app.component";
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule {}
As you can see in the above code, we have imported NgModule and BrowserModule in our App module. We have also imported one component, AppComponent.
However, if we want to import more components in our modules, we can use declarations to register our components in our module. If we’re going to import another module, we can use imports
.
We can easily create new modules in our Angular application using the following command.
# angular
ng generate module user-auth
This command will create a new folder inside the App folder and create a new module, as shown below.
Output:
Components in Angular
The component is a piece of code created for a specific task.
For example, if we want users to register themselves, we can create a new component, register
, that will allow users to register on our application.
Components can be reused depending on their needs where they can be reused. For example, if we want to use header and footer in all of our web pages in Angular application, we can create header
and footer
and import these components wherever we want to use them.
A component consists of 4 files:
- CSS file used for writing styles for the component.
- HTML file used for writing frontend or view of our component.
- Specs file used for writing test cases.
- Component file in which we write logical part of our component containing functions, API calls, etc.
Now, let’s create a new application by using the following command.
# angular
ng new my-app
After creating our new application in Angular, we will go to our application directory using this command.
# angular
cd my-app
Now, let’s run our app to check if all dependencies are installed correctly.
# angular
ng serve --open
Then, we will open the app.component.ts
file.
# angular
import { Component } from "@angular/core";
@Component({
selector: "app-root",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"]
})
export class AppComponent {
title = "App Component";
}
As you can see in the above code, we have imported a component
method from @angular/core
. Now, we can define selector
, templateUrl
, and styleUrls
for our component using the component
we just imported.
The selector is a tag used to display our component’s content in our Angular application.
From the above code, if we create a tag <app-root></app-root>
in our index.html
file, the Angular application will detect the tag and replace it with our component’s content.
templateUrl
is used to define the template for our component. And styleUrls
will define the CSS file for our component in which styles are written.
We can easily create new components in our Angular application using the following command.
# angular
ng generate component login
This command will create 4 new files with a new selector for our component.
Output:
Conclusion
In this tutorial, we learned about modules and components in Angular, how to create new components, understand their methods, and what they are made up of.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedInRelated Article - Angular Component
- How to Delete Component in Angular
- How to Pass Data Between Components in Angular 2
- How to Create New Component in Angular