How to Implement Table Pagination Layout in AngularJS
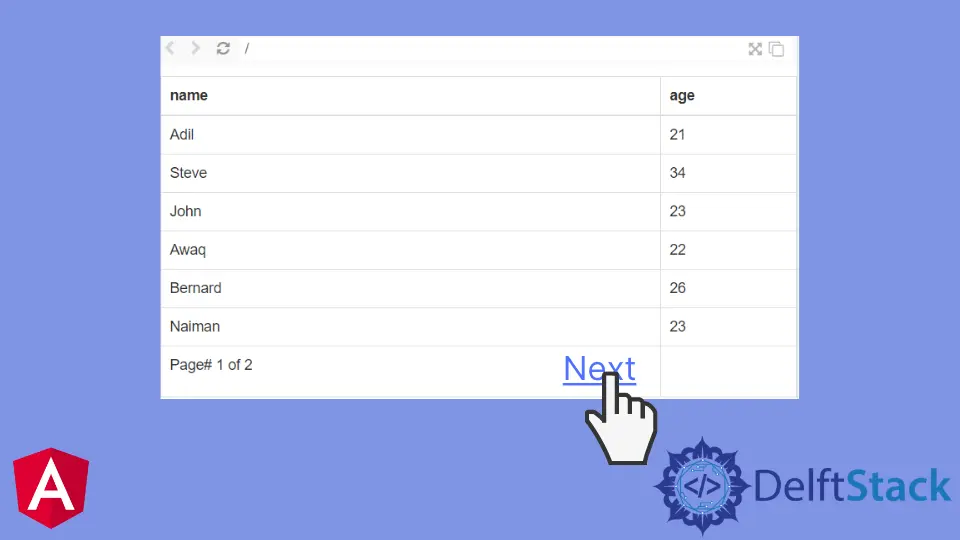
This article will introduce the table pagination layout in AngularJS and walk you through the steps to implement this in your data.
Table Pagination Layout in AngularJS
Table pagination is an essential feature in AngularJS necessary for any application that displays a list of data, such as an inventory or product catalog.
It allows users to scroll through large sets of data on one page rather than click through one page at a time, providing an easy-to-read layout perfect for mobile devices.
Table pagination lets you break down a long table into smaller, more manageable chunks by splitting the table into sections and paginating them for the readers to scan the information efficiently.
It provides a set of directives to implement and some utility functions and filters to ease the task of configuring the table.
Mainly, the pagination feature of the table is created using ng-repeat
to generate a list of data, loop through the data, and display it in the table. It also has the ng-click
directive that triggers an event when clicking on each row.
Steps to Implement Table Pagination Layout in AngularJS
AngularJS table pagination is an excellent way to paginate data in a horizontal or vertical table. The most common use case for AngularJS table pagination is to provide an efficient way to view and navigate large data tables.
We can use it for both static and dynamic data. And it also allows you to implement the sorting, filtering and searching functionality.
There are four steps to implement AngularJS table pagination:
- Load the data in JSON format and store it in a variable called
data
. - Create a controller and assign the variable to a scope variable called
data
. - Create an AngularJS factory that will generate pagination links, add them to the controller’s scope, and return them as an object of arrays of links with page numbers and page count, respectively. The factory will also define what type of pagination links you want to use (table, tree, list) and how many items are displayed on one page (page size).
- Add a pagination button at the bottom of each row inside your table.
Let’s discuss an example to understand table pagination in AngularJS better.
HTML Code:
<!DOCTYPE html>
<html>
<head>
<link data-require="bootstrap-css@*" data-semver="3.3.1" rel="stylesheet" href="//maxcdn.bootstrapcdn.com/bootstrap/3.3.1/css/bootstrap.min.css" />
<script data-require="angular.js@*" data-semver="1.4.3" src="https://code.angularjs.org/1.4.3/angular.js"></script>
<script src="services.js"></script>
<script src="main.js"></script>
</head>
<body>
<div ng-app="paginationApp">
<div style="margin-left:5px;margin-top:10px" ng-controller="mainCtrl">
<pgn-table conf="config"></pgn-table>
</div>
</div>
</body>
</html>
JavaScript Code:
var myApp = angular.module('paginationApp');
myApp.controller('mainCtrl', function($scope,demo) {
demo.then(function(data){
$scope.config.myData=data;
});
$scope.config = {
heads: ['name', 'age']
};
});
myApp.directive('pgnTable', ['$compile',
function($compile) {
return {
restrict: 'E',
templateUrl: 'Sample.html',
replace: true,
scope: {
conf: "="
},
controller: function($scope) {
$scope.currentPage=1;
$scope.numLimit=6;
$scope.start = 0;
$scope.$watch('conf.myData',function(newVal){
if(newVal){
$scope.pages=Math.ceil($scope.conf.myData.length/$scope.numLimit);
}
});
$scope.hideNext=function(){
if(($scope.start+ $scope.numLimit) < $scope.conf.myData.length){
return false;
}
else
return true;
};
$scope.hidePrev=function(){
if($scope.start===0){
return true;
}
else
return false;
};
$scope.nextPage=function(){
console.log("next pages");
$scope.currentPage++;
$scope.start=$scope.start+ $scope.numLimit;
console.log( $scope.start)
};
$scope.PrevPage=function(){
if($scope.currentPage>1){
$scope.currentPage--;
}
console.log("next pages");
$scope.start=$scope.start - $scope.numLimit;
console.log( $scope.start)
};
},
compile: function(elem) {
return function(ielem, $scope) {
$compile(ielem)($scope);
};
}
};
}
]);
After that, we use the following random information in tables.
{
"demo": [{
"name": "Adil",
"age": 21
}, {
"name": "Steve",
"age": 34
}, {
"name": "John",
"age": 23
}, {
"name": "Awaq",
"age": 22
}, {
"name": "Bernard",
"age": 26
}, {
"name": "Naiman",
"age": 23
}, {
"name": "Rotan",
"age": 45
}, {
"name": "David",
"age": 32
}, {
"name": "Jade",
"age": 32
}, {
"name": "Reven",
"age": 22
}, {
"name": "Philp",
"age": 28
}, {
"name": "Salt",
"age": 38
}]
}
Click here to check the live demonstration of the code above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook