Providers in AngularJS
- Providers in AngularJS
-
Difference Between
Provider
andFactory
In AngularJS - Difference Between Provider and Service In AngularJS
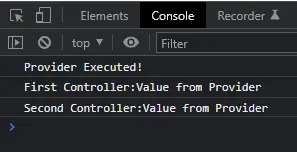
We will introduce providers in AngularJS and the differences between provider
, factory
, and service
in AngularJS.
Providers in AngularJS
Providers are a type of service. The provider()
function allows us to create a configurable service that contains the $get
method.
Let’s imagine creating a web application that uses APIs; if we need to set an API key to access the data from API in our application, we can set that in the module
config and pass input to the provider using the $provide
service.
Let’s create a module with a provider that will return a value when a controller calls the provider. First, we will add the AngularJS library and app.js
file using script
tags.
# AngularJS
<head>
<script src="https://code.angularjs.org/1.4.0-beta.4/angular.js"></script>
<script src="app.js"></script>
</head>
We will define AngularJS application using ng-app
and controller using ng-controller
.
# AngularJS
<body ng-app="myApp">
<div ng-controller="myController"></div>
</body>
In app.js
, we will create our module.
# AngularJS
var module = angular.module("myApp", []);
We will use a provider
; the providers do as their name implies, which is that they provide values, so in this case, we will provide a string value. The provider has one method defined: $get
.
The provider can have multiple methods, but Angular calls are $get
. So, we will assign a function to the $get
method that will return a string value.
# AngularJS
module.provider("myProvider", function(){
this.$get = function(){
return "Value from Provider";
};
});
We will inject the provider in our controller and console.log()
the value from the provider.
# AngularJS
module.controller("myController", function(myProvider){
console.log(myProvider);
});
Output:
As shown in the above example, we got the exact value from the provider in our console. So that worked, and we can use a provider to return a value and inject that into our method.
It’s important to note that this is true that all the providers are all the same and service and factory are also the same. But no matter how many times we inject the provider, the value will get executed just once.
We can test it by creating another controller, as shown below.
# AngularJS
module.controller("myController", function(myProvider){
console.log("First Controller:" + myProvider);
});
module.controller("myController2", function(myProvider){
console.log("Second Controller:" + myProvider);
});
We will use console.log()
inside our provider’s $get
method to log a value inside the provider. So whenever this provider is executed, it will log the same value.
In this way, we can check whether our provider is executed once or twice.
# AngularJS
module.provider("myProvider", function(){
this.$get = function(){
console.log("Provider Executed!")
return "Value from Provider";
};
});
Let’s add div
for our second controller in index.html
.
# AngularJS
<div ng-controller="myController"></div>
<div ng-controller="myController2"></div>
Output:
The above example shows that the provider is executed only once, but the provider’s value is injected in both controllers. We got two output messages from our controllers.
It is important to notice that Angular executes it when a provider gets injected, calls $get and store and remembers that value. Every subsequent time the provider gets injected, it returns the value it extracted the first time.
Difference Between Provider
and Factory
In AngularJS
Let’s create a factory
with the same functionality as the provider we created. Now we will copy our provider and change it to a factory
.
The factory doesn’t have a method $get
, so we will directly call the function in our factory and return a value. That is the only part that is different from the provider.
We will inject our factory into both controllers. Our code in app.js
will look like below.
# AngularJS
var module = angular.module("myApp", []);
module.factory("myFactory", function(){
console.log("Factory Executed!")
return "Value from Factory";
});
module.controller("myController", function(myFactory){
console.log("First Controller:" + myFactory);
});
module.controller("myController2", function(myFactory){
console.log("Second Controller:" + myFactory);
});
Output:
As shown in the above example, we get the same results. Both providers and the factory do the same thing, but a factory
can do it in a little less code.
Difference Between Provider and Service In AngularJS
Let’s create a service
with the same functionality as the provider we created. Now we will copy our provider and change it to a service.
Service doesn’t have a method $get
, so we will directly call the function in our service the same as factory and return a value. That is the only part that is different from the provider.
We will inject our service into both controllers. Our code in app.js
will look like below.
# AngularJS
var module = angular.module("myApp", []);
module.service("myService", function(){
console.log("Service Executed!");
this.getValue = function(){
return "Object from Service";
}
});
module.controller("myController", function(myService){
console.log("First Controller:" + myService.getValue());
});
module.controller("myController2", function(myService){
console.log("Second Controller:" + myService.getValue());
});
Output:
As shown in the above example, we get the same results. Both providers and the service do the same thing, but the service returns an object instead of a string.
The main point is when to use a provider
, factory
, and service
. If we want to return a value instead of an object, we can’t use a service.
We have to use a factory instead of a service, but we will use a factory to return an object instead of a value. The provider gives us access doing configuration time.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn