How to Display Image in AngularJS
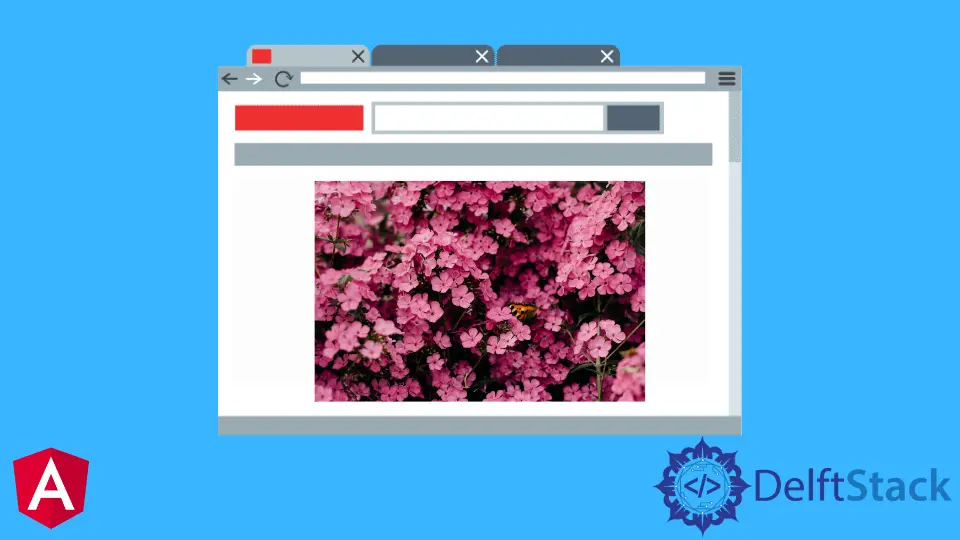
We will introduce how to add images in AngularJS with examples.
Display Image in AngularJS
Images are the most important part of any web application or website. We will use the ng-src
directive to add a single image.
We will discuss displaying an image inside the ng-repeat
directive in AngularJS. The ng-src
order is used along with image elements to show images from the model in AngularJS.
The image will be held in a folder on the server. And it will be shown by setting the path of the image to the ng-src
directive in AngularJS.
Let’s go through an example and display a single image using the ng-src
directive.
# AngularJS
<html>
<head>
<title></title>
</head>
<body>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.3.9/angular.min.js"></script>
<script type="text/javascript">
var app = angular.module('ngApp', [])
app.controller('ngController', function ($scope) {
});
</script>
<div ng-app="ngApp" ng-controller="ngController">
<img alt="" ng-src="https://www.delftstack.com/ezoimgfmt/d33wubrfki0l68.cloudfront.net/7748c5aa61aa13fd4c346e3cbfebe49f2dd4d580/2738b/assets/img/logo.png?ezimgfmt=rs:187x36/rscb5/ng:webp/ngcb5"/>
</div>
</body>
</html>
Output:
As you can see from the above example, it is quite simple to add an image inside the AngularJS application.
Display Multiple Images in AngularJS
Now, let’s go through another example and try to add multiple images from JSON.
The below HTML markup contains an HTML div
to which ng-app
and ng-controller
directives have been given. The HTML div
contains an HTML button and table consisting of image elements which will be settled from the JSON array using the ng-repeat
directive.
The button has been given the ng-click
directive. The general table function of the controller gets named when the button is clicked.
JSON objects are created and allocated to the consumer JSON array with the general table function.
The JSON object contains the consumerId
, Name
, and Photo
fields. The Photo
field supplies the URL of the image.
The ng-repeat
directive name implies the element based on the length of the collection.
In this system, it will repeat the tr
element. The tbody
element of the HTML has been appointed ng-repeat
directive to repeat through all the items of the consumer JSON array.
A tr
element is developed and appended into the HTML table for each JSON object in the consumer
JSON array. The Photo
field will be allocated to the ng-src
directive, which will read the image URL from the model and display the image, as shown below.
# AngularJS
<html>
<head>
<title>Consumers</title>
</head>
<style>
td{
border: 1px solid black;
}
</style>
<body>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.3.9/angular.min.js"></script>
<script type="text/javascript">
var app = angular.module('ngApp', [])
app.controller('ngController', function ($scope) {
$scope.IsVisible = false;
$scope.GetData = function () {
$scope.consumer= [
{ consumerId: 1, Name: "Google", Photo: "Images/1.jpg" },
{ consumerId: 2, Name: "Delft Stack", Photo: "Images/2.jpg" },
{ consumerId: 3, Name: "Yahoo", Photo: "Images/3.jpg" },
];
$scope.IsVisible = true;
};
});
</script>
<div ng-app="ngApp" ng-controller="ngController">
<input type="button" value="Get Data" ng-click="GetData()" />
<hr />
<table cellpadding="0" cellspacing="0" ng-show="IsVisible">
<tr>
<th>Company Id</th>
<th>Name</th>
<th>Logo</th>
</tr>
<tbody ng-repeat="m in consumer">
<tr>
<td>{{m.consumerId}}</td>
<td>{{m.Name}}</td>
<td><img alt="{{m.Name}}" ng-src="{{m.Photo}}"/></td>
</tr>
</tbody>
</table>
</div>
</body>
</html>
Output:
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn