How to Bind Ng-Model to a List of Radio Buttons in Angular
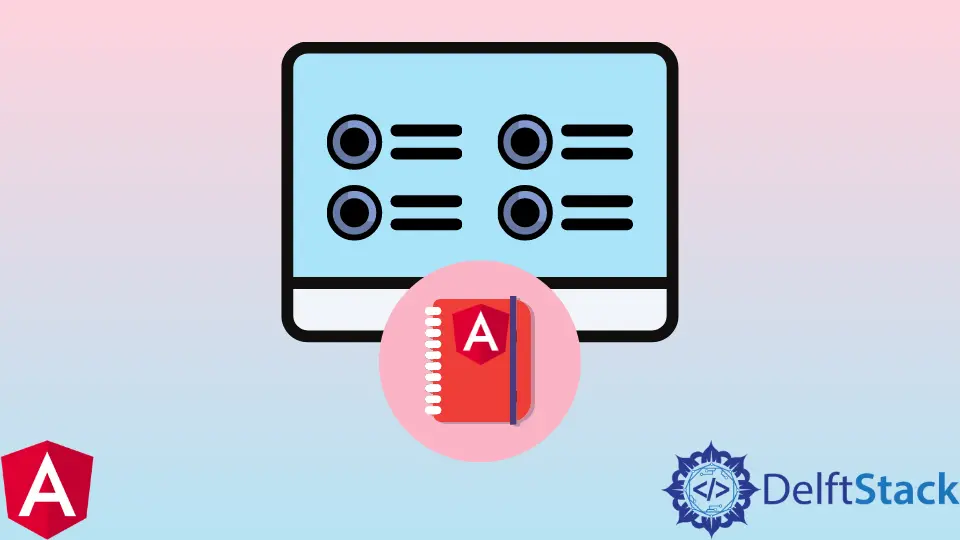
When a webpage user selects an item from multiple options, redisplaying the selected item under the list of options helps the user understand his choice. This enables the user to easily move on to the next thing to do on the webpage.
Angular features such as this are ideal when developing an e-commerce website. To get the website to display the selected item, we will look at using the ng-model
to perform this function.
The ng-model
can be bound to a list of radio buttons on a webpage, and we will learn two different methods to perform these functions.
Use ng-model
With the $scope
Function to Bind ng-model
to a List of Radio Buttons in Angular
This simple example will define the ng-model
in the index.html
. The HTML code will look like this.
HTML code:
<!DOCTYPE html>
<hmtl ng-app="testApp">
<head>
<script data-require="angularjs@1.5.5" data-semver="1.5.5" src="https://
code.angularjs.org/1.5.5/angular.js"></script>
<link rel="stylesheet" href="style.css" />
<script src="script.js"></script>
</head>
<body ng-controller="testController">
<form>
<div ng-repeat="option in occurrenceOptions track by $index">
<input type="radio" name="occurrences" ng-value="option"
ng-model="model.selectedOccurrence" />
<label>{{ option }}</label>
</div>
</form>
<div>The selected value is : {{ model.selectedOccurrence }}</div>
</body>
Then, in the script.js
file, we run the $scope
function inside the controller. The $scope
helps bind the controller to the HTML where we have carried out the codings for the ng-model
.
JavaScript code:
(function () {
var app = angular.module('testApp', []);
app.controller('testController', function($scope) {
$scope.occurrenceOptions = [];
$scope.occurrenceOptions.push('previous');
$scope.occurrenceOptions.push('current');
$scope.occurrenceOptions.push('next');
$scope.model = {};
$scope.model.selectedOccurrence = 'current';
});
}());
Click here to run the code provided in this example.
Use the ng-model
With the this
Function to Bind ng-model
to a List of Radio Buttons in Angular
The significant difference between this example and the previous is that we declare the this
function inside the controller, basically replacing the $scope
function.
We slightly change the ng-repeat
aspect of the index.html
, which will look like the code below.
HTML code:
<!DOCTYPE html>
<html ng-app="testApp">
<head>
<script data-require="angularjs@1.5.5" data-semver="1.5.5" src="https://
code.angularjs.org/1.5.5/angular.js"></script>
<link rel="stylesheet" href="style.css" />
<script src="script.js"></script>
</head>
<body ng-controller="testController as vm">
<form>
<div ng-repeat="option in vm.occurrenceOptions">
<input type="radio" name="occurrence" ng-value="option" ng-model="vm.
selectedOccurrence" />
<label>{{ option }}</label>
</div>
</form>
<div>The selected value is : {{ vm.selectedOccurrence }}</div>
</body>
Then, in the script.js
, we declare the this
function inside the controller.
JavaScript code:
(function () {
var app = angular.module('testApp', []);
app.controller('testController', function () {
var vm = this;
vm.occurrenceOptions = [];
vm.occurrenceOptions.push('previous');
vm.occurrenceOptions.push('current');
vm.occurrenceOptions.push('next');
vm.selectedOccurrence = 'current';
});
})();
Click here to run the code used in this example.
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn