AngularJs $watchcollection
- AngularJs Watchers and How It Works
-
Use
$watchCollection
to Watch Changes in a Collection in AngularJs
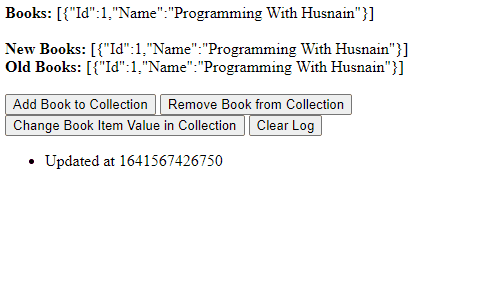
We will introduce AngularJs watchers and the types of watchers we can accomplish using AngularJs.
AngularJs Watchers and How It Works
Watchers are just keeping track of scope variables and changes in their values. Watchers monitor scope variables and the changes of the available values inside the scope variables.
To understand watchers in detail, let’s go through an example. Let’s create a controller-related markup with a div
associated with controller a
.
This div
will have two elements, one will be a textbox, and another one will be a div. As shown below, the textbox will have two-way binding to x
, and a div will have one-way binding to y
.
# angular
<div ng-controller="a">
<input ng-model="x">
<div>{{b}}</div>
</div>
Our controller a
will define three scope variables x
, y
, and z
. As shown below, we have assigned values as 1, 2, and 3 to these three variables.
# angular
app.controller("a", function(){
$scope.x = 1;
$scope.y = 2;
$scope.z = 3;
})
First, let’s discuss how the watcher works. Watch keeps track of a variable and its value. So we have a scope variable x
bound to the textbox using the ng-model
. That means there is a two-way binding accomplished.
Whenever we have data binding to a scope variable, a watch variable will automatically be created, which the AngularJs framework will handle. Whenever we have some data binding to scope variables, all those variables will have their respective Watchers automatically created by AngularJs.
In the example above, we have two elements with some data binding to two different scope variables and two different watchers created in the memory.
Some variables are not being watched because they are not bound with any element. From the above example, variable z
is not being watched, and no watcher is created automatically for variable z
.
AngularJs takes its own decision on whether to watch a particular variable or not based on the concepts of data binding.
AngularJs can execute our custom functions based on watchers or whenever a value of scope variable changes. If the value of x
is changed, I can create a custom function that can be executed.
Use $watchCollection
to Watch Changes in a Collection in AngularJs
AngularJs 1.1.4 introduced a new method to watch and observe changes in a collection, either as an array or an object.
The $watchCollection()
is used to push something to a collection or remove some elements from a collection. $watchCollection()
can return both new and previous collections.
Let’s create an example to understand how we can use $watchCollection()
.
We will add a book to a collection, remove a book from that collection, change the book from a collection to check how $watchCollection()
reacts to all of these functions.
# angular
var myApp = angular.module('myApp',[]);
myApp.controller('watchCollectionExample', function($scope) {
$scope.Books = [{
Id : 1,
Name: 'Programming With Husnain'
}];
$scope.AddItem = function() {
$scope.Books.push({
Id : $scope.Books.length + 1,
Name: 'Programming With Husnain ' + $scope.Books.length
});
}
$scope.RemoveItem = function() {
$scope.Books.pop(1);
}
$scope.ModifyItem = function() {
$scope.Books[0].Name = 'Coding Basics';
}
$scope.$watchCollection('Books', function(newCollection, oldCollection){
$scope.newValue = newCollection;
$scope.oldValue = oldCollection;
$scope.Itemslog.splice(0, 0, "Updated at " + (new Date()).getTime());
});
$scope.Itemslog = [];
$scope.LogClear = function(){
$scope.Itemslog.length = 0;
}
});
Now let’s create a template.
# angular
<div ng-controller="watchCollectionExample">
<b>Books:</b> {{Books}} <br/><br/>
<b>New Books:</b> {{newValue}} <br/>
<b>Old Books:</b> {{oldValue}}
<br/><br/>
<button ng-click="AddItem()">Add Book to Collection</button>
<button ng-click="RemoveItem()">Remove Book from Collection</button>
<button ng-click="ModifyItem()">Change Book Item Value in Collection</button>
<button ng-click="LogClear()">Clear Log</button>
<ul>
<li ng-repeat="book in Itemslog"> {{ book }} </li>
</ul>
</div>
Output:
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn