How to Set Cookie in Angular
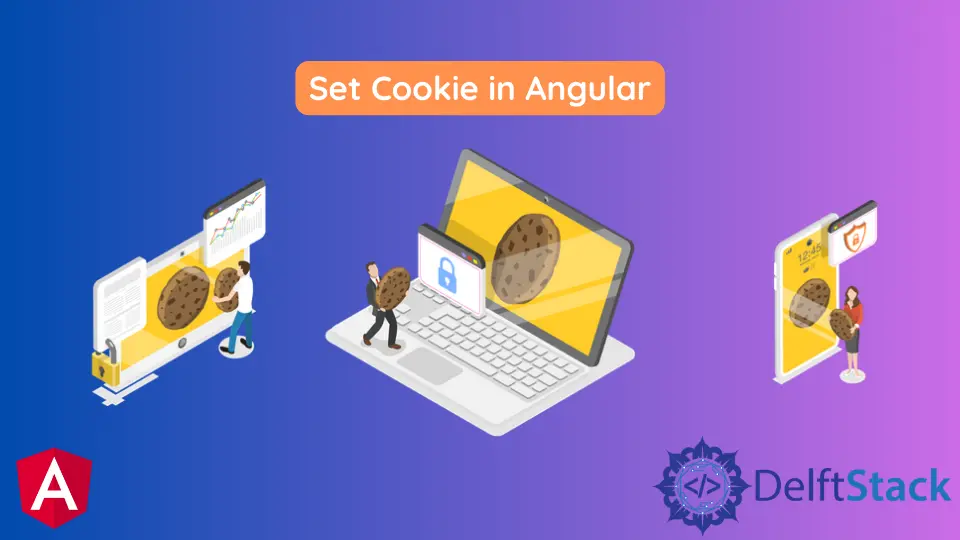
In this article, we will discuss cookies in Angular. We will introduce how to set up cookies in Angular with examples.
Cookies in Angular
Cookies are small packages of details typically stored by your browser, and websites tend to utilize cookies for numerous things. Cookies persist across different requests, and browser sessions should help us set them, and they can be an excellent technique for authentication in some web apps.
We can use cookies to store the important data in many situations, such as for authentication or saving user details when logged in. We can easily retrieve data from cookies whenever we want.
Generally, we can only store 20 cookies per web server and no more than 4KB
of data in each cookie, and they can last indefinitely should we prefer to determine the max-age attribute.
Let’s create a new application in Angular by using the following command.
ng new my-app
After creating our new application in Angular, we will go to our application directory using this command.
cd my-app
Let’s run our app to check if all dependencies are installed correctly.
ng serve --open
We need to set the cookies in Angular using a library ngx-cookie-service
.
Set Cookies in Angular
Angular provides a library ngx-cookie-service
that can be used to set cookies in our web application. We can easily install this library using the following command.
npm install ngx-cookie-service --save
This command will download and install the ngx-cookie-service
library in our project’s node_modules
folder, and it will also be included in the dependencies.
Once we’ve successfully added this library to our project, we can set up cookies. It’s suggested that we import the CookieService
within our module file and then add it to our provider’s array, as shown below.
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { CookieService } from 'ngx-cookie-service';
import { AppComponent } from './app.component';
import { HelloComponent } from './hello.component';
@NgModule({
imports: [BrowserModule, FormsModule],
declarations: [AppComponent, HelloComponent],
bootstrap: [AppComponent],
providers: [CookieService],
})
export class AppModule {}
Once we’ve added it as a provider, we can use it in one of our app.component.ts
, as shown below.
import { Component } from '@angular/core';
import { CookieService } from 'ngx-cookie-service';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
constructor(private cookieService: CookieService) {}
ngOnInit() {
this.cookieService.set('Test', 'Hasnain');
}
getCookie() {
const value: string = this.cookieService.get('Test');
console.log(value);
}
}
Output:
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn