How to Copy in Angular
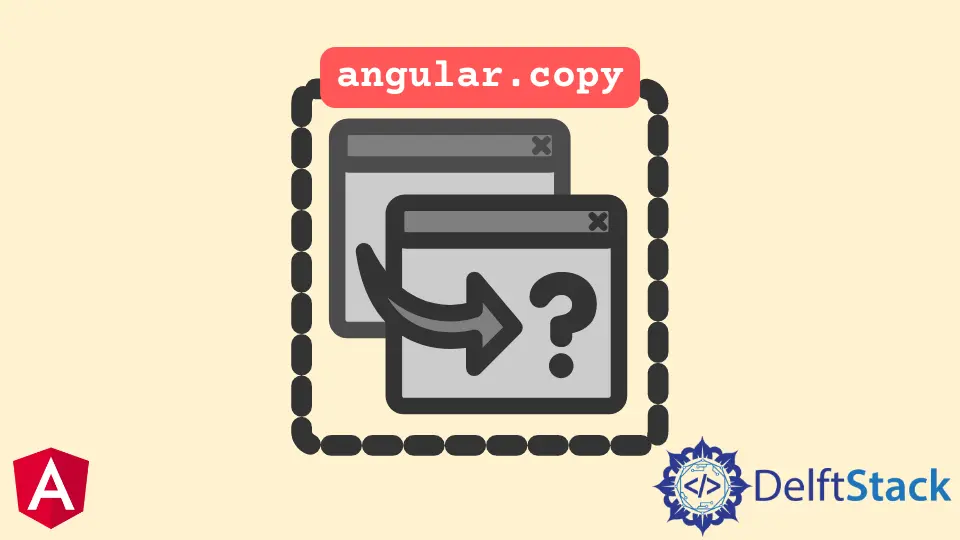
We will demonstrate when and how to use the angular.copy
in angular.
angular.copy
in Angular
angular.copy
is a powerful method for keeping the value of an object that we want to copy to another variable.
When we use angular.copy
, changing the property’s value or adding a new property will update all objects referencing the same object. angular.copy
is not intended to be an all-purpose copy function.
It has some limitations as well. If no destination is supplied to angular.copy
, a copy of the object or an array will be created.
If we provide the destination, all of its elements for array and properties for objects will be deleted, and all elements/properties from the source will be copied to it. If the provided source is not an object or an array including null
and undefined
, the source will be returned.
If the provided source
is identical to the provided destination, it will throw an exception. Some object types that are not handled correctly by angular.copy
like File, Map, ImageData, MediaStream, Set, WeakMap, getter, and setter.
angular.copy
deeply duplicates the variable. A deep copy of a variable is the one that doesn’t share the same memory address as the original variable.
For example, let’s create a form that will take users’ input and use angular.copy
. Our HTML
code will be like below.
# angular
<body ng-app="NgCopy">
<div ng-controller="NgController">
<form novalidate class="simple-form">
<label>Name: <input type="text" ng-model="user.name" /></label><br />
<button ng-click="ngreset()">RESET</button>
<button ng-click="ngupdate(user)">SAVE</button>
</form>
<pre>form = {{user | json}}</pre>
<pre>member = {{member | json}}</pre>
</div>
</body>
And our code in script.js
will look like below.
# angular
(function(angular) {
'use strict';
angular.
module('NgCopy', []).
controller('NgController', ['$scope', function($scope) {
$scope.member = {};
$scope.ngreset = function() {
$scope.user = angular.copy($scope.member);
};
$scope.ngupdate = function(user) {
angular.copy(user, $scope.member);
};
$scope.ngreset();
}]);
})(window.angular);
As seen in the above example, we used angular.copy
two times. In $scope.user = angular.copy($scope.member);
we have used it with one parameter, and in angular.copy(user, $scope.member);
we used angular.copy
with two parameters.
Output:
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn