How to Use Angular CLI Webpack Configuration
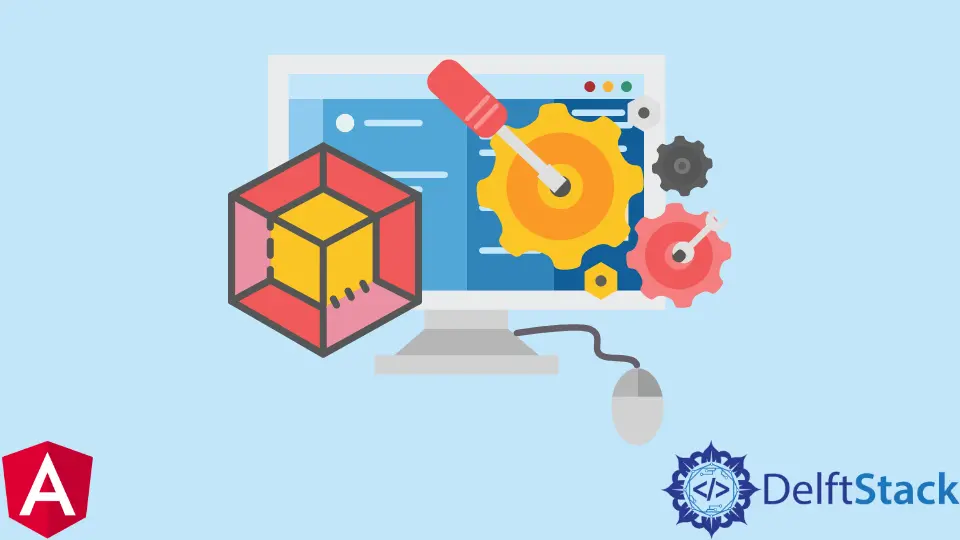
Angular CLI is a command-line interface that can generate Angular projects, add dependencies, and run development and production build. It provides all the tools required to transform an idea into a full-fledged application.
The Angular CLI provides commands for creating the project, adding and running tests, generating components, services, pipes, scaffolding modules, features, etc. It also provides a webpack configuration file.
The Webpack Module
Webpack is a module bundler that handles modules with dependencies and generates static assets representing those modules. We can use it in conjunction with other tools like Babel or TypeScript to support different types of JavaScript syntax.
Webpack isn’t just for assembling source files; it can also execute various extra activities due to its handling ability of many plugins. Webpack module loaders can interpret several file formats.
This module enables Angular TypeScript files, for example, to utilize the import line to import CSS files. Webpack is often concealed behind the Angular command-line tool.
However, while developing an Angular application, it may be required to modify the webpack settings. Older Angular versions allowed you to eject the webpack settings and directly alter them.
But access to the base configuration has been blocked in Angular 8. Still, we may extend the webpack configuration by adding new loaders or configuration variables.
The Angular CLI also includes a development server that compiles the application on each change and reloads it in the browser with every new change. This makes it an effective tool for building applications quickly without worrying about configuring webpack separately.
Steps to Get the Angular CLI Webpack Configuration
The following steps must be accomplished to use the Angular CLI with webpack:
- Install NodeJS and npm on your system.
- If you are using an older version of Node.js, install 4 or higher and upgrade your npm version to 5 or higher before installing Angular CLI. You can also use nvm for this purpose.
- Install Angular globally with
npm install -g @angular/CLI
. - Create an Angular project using
ng new PROJECTNAME
. - Add the following scripts in
package.json
:"ng": "ng", "start": "ng serve", "build": "ng build"
. - Add webpack as a dependency in
package.json
.
Example (App.component.ts
):
import { Component, Inject } from "@angular/core";
import { APP_VERSION } from '../webpack/app-version';
@Component({
selector: "app-root",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"]
})
export class AppComponent {
constructor(
@Inject(APP_VERSION) public appVersion: string
) {
}
}
Example (App.module.ts
):
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { AppComponent } from './app.component';
import { APP_VERSION } from '../webpack/app-version';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule
],
providers: [{
provide: APP_VERSION,
useValue: '12345678'// AppVersion
}],
bootstrap: [AppComponent]
})
export class AppModule { }
Click here to check the live demonstration of the code mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook