SciPy scipy.integrate.quad Method
-
Syntax of
scipy.integrate.quad()
: -
Example Codes :
scipy.integrate.quad()
Method to Find Integral -
Calculating Integral of Cosine Using
scipy.integrate.quad()
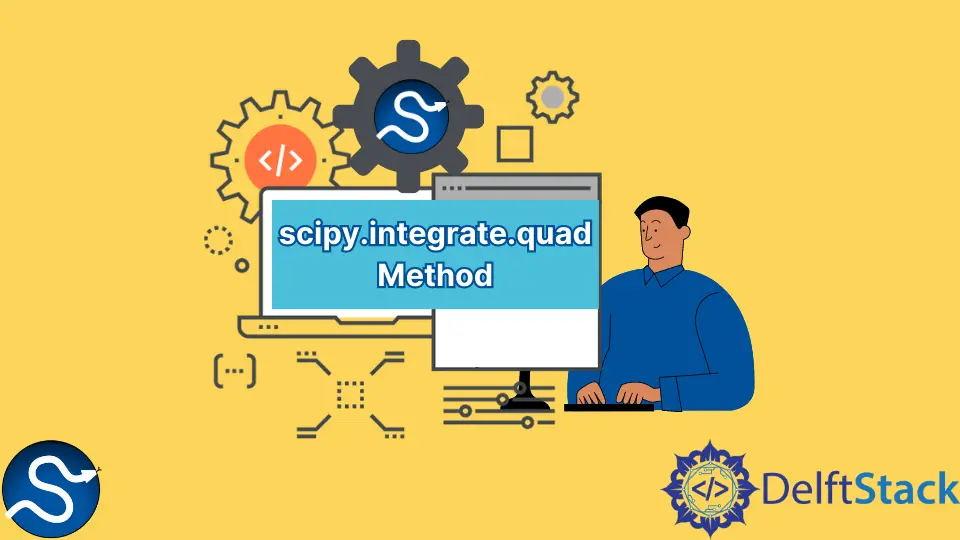
Python Scipy scipy.integrate.quad()
method evaluates the integration of a given function in between the provided lower and upper limits.
Syntax of scipy.integrate.quad()
:
scipy.integrate.quad(func, a, b)
Parameters
func |
It is the function whose definite integral is to be calculated. |
a |
Lower limit. Integration of function will start from here. It takes float value. |
b |
Upper limit. Integration of function will stop here. It takes a float value. |
Return
It returns a tuple of two values :
- Value of integral.
- Estimate error of the integral in between actual and approximate value.
Example Codes : scipy.integrate.quad()
Method to Find Integral
import numpy as np
import scipy
from scipy.integrate import quad
def func(x):
return x
integral, error = scipy.integrate.quad(func, 0, 4)
print("The result of the integration of func from 0 to 4 is: " + str(integral))
print("The error value in the integration is:" + str(error))
Output:
The result of the integration of func from 0 to 4 is: 8.0
The error value in the integration is:8.881784197001252e-14
Here, a function named func
is created, which is simply a linear function that returns the input value without any modification. When the scipy.integrate.quad()
method is called, func
is integrated between lower bound 0
and upper bound 4
, and we get a tuple of 2 values as an output from the method. The first value represents the value of the definite integral, while the second value represents the error while estimating the integral.
Calculating Integral of Cosine Using scipy.integrate.quad()
import numpy as np
import scipy
from scipy.integrate import quad
lower_bound = 0
upper_bound = np.pi / 2
def func(x):
return np.cos(x)
value, err = quad(func, lower_bound, upper_bound)
print("Integral value of cosine function from 0 to pi/2 is:" + str(value))
print("Estimated error is: " + str(err))
Output:
Integral value of cosine function from 0 to pi/2 is: 0.9999999999999999
Estimated error is: 1.1102230246251564e-14
Here, cos(x)
is integrated in between limits 0
and np.pi/2
. The func
function is created that returns cos(x)
, which is passed into the quad
method along with upper and lower interval limits, finally producing respective integral value and absolute error.
Several other optional parameters can be used to tune the output.