SciPy scipy.integrate.quad メソッド
Bhuwan Bhatt
2023年1月30日
-
scipy.integrate.quad()
の構文: -
コード例:
scipy.integrate.quad()
積分を求めるメソッド -
scipy.integrate.quad()
を使用して正弦の積分を計算する
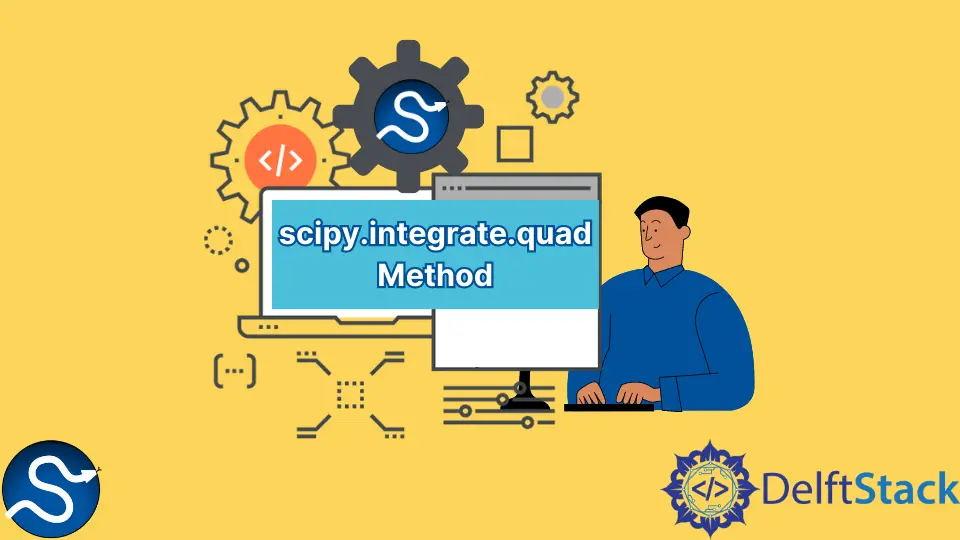
Python Scipy scipy.integrate.quad()
メソッドは、指定された下限と上限の間の特定の関数の統合を評価します。
scipy.integrate.quad()
の構文:
scipy.integrate.quad(func, a, b)
パラメーター
func |
これは、定積分が計算される関数です。 |
a |
下限。ここから機能の統合が始まります。浮動小数点値を取ります。 |
b |
上限。機能の統合はここで停止します。浮動小数点値を取ります。 |
戻り値
2つの値のタプルを返します。
- 積分の値。
- 実際の値と近似値の間の積分の誤差を推定します。
コード例:scipy.integrate.quad()
積分を求めるメソッド
import numpy as np
import scipy
from scipy.integrate import quad
def func(x):
return x
integral, error = scipy.integrate.quad(func, 0, 4)
print("The result of the integration of func from 0 to 4 is: " + str(integral))
print("The error value in the integration is:" + str(error))
出力:
The result of the integration of func from 0 to 4 is: 8.0
The error value in the integration is:8.881784197001252e-14
ここでは、func
という名前の関数が作成されます。これは、変更なしで入力値を返す単純な線形関数です。scipy.integrate.quad()
メソッドが呼び出されると、func
が下限 0
と上限 4
の間に統合され、メソッドからの出力として 2つの値のタプルが取得されます。最初の値は定積分の値を表し、2 番目の値は積分を推定する際の誤差を表します。
scipy.integrate.quad()
を使用して正弦の積分を計算する
import numpy as np
import scipy
from scipy.integrate import quad
lower_bound = 0
upper_bound = np.pi / 2
def func(x):
return np.cos(x)
value, err = quad(func, lower_bound, upper_bound)
print("Integral value of cosine function from 0 to pi/2 is:" + str(value))
print("Estimated error is: " + str(err))
出力:
Integral value of cosine function from 0 to pi/2 is: 0.9999999999999999
Estimated error is: 1.1102230246251564e-14
ここで、cos(x)
は制限 0
と np.pi/2
の間に統合されています。func
関数が作成され、cos(x)
が返されます。これは、間隔の上限と下限とともに quad
メソッドに渡され、最終的にそれぞれの積分値と絶対誤差を生成します。
他のいくつかのオプションのパラメーターを使用して、出力を調整できます。