Pandas DataFrame DataFrame.shift() Function
-
Syntax of
pandas.DataFrame.shift()
: -
Example Codes:
DataFrame.shift()
Function to Shift Along Row -
Example Codes:
DataFrame.shift()
Function to Shift Along Column -
Example Codes:
DataFrame.shift
Method Withfill_value
Parameter
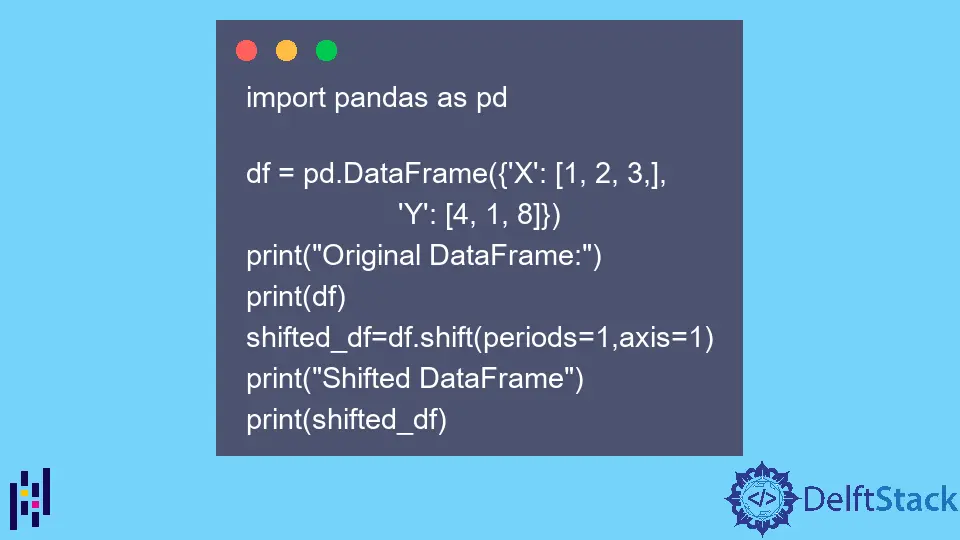
Pandas DataFrame.shift
method is used to shift the index of DataFrame
by a specified number of periods with an optional time frequency.
Syntax of pandas.DataFrame.shift()
:
DataFrame.shift(periods=1, freq=None, axis=0, fill_value=None)
Parameters
periods |
Integer. Decides number of periods to shift the index which can be negative or positive |
freq |
DateOffset , tseries.offsets , timedelta , or str . Optional parameter used to shift index values without realigning the data |
axis |
shift along the row (axis=0) or column (axis=1) |
fill_value |
scalar value to be used for newly introduced missing values. |
Return
It returns a DataFrame
object with shifted index values.
Example Codes: DataFrame.shift()
Function to Shift Along Row
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 3,],
'Y': [4, 1, 8]})
print("Original DataFrame:")
print(df)
shifted_df=df.shift(periods=1)
print("Shifted DataFrame")
print(shifted_df)
Output:
Original DataFrame:
X Y
0 1 4
1 2 1
2 3 8
Shifted DataFrame
X Y
0 NaN NaN
1 1.0 4.0
2 2.0 1.0
Here, we set the value of periods
to 1
, and this shifts the rows of DataFrame
from the top towards the bottom by 1
unit.
While shifting towards the bottom, the topmost rows become vacant and get filled by NaN
values by default.
If we want to shift rows from the bottom towards the top, we can set a negative value of the periods
parameter.
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 3,],
'Y': [4, 1, 8]})
print("Original DataFrame:")
print(df)
shifted_df=df.shift(periods=-2)
print("Shifted DataFrame")
print(shifted_df)
Output:
Original DataFrame:
X Y
0 1 4
1 2 1
2 3 8
Shifted DataFrame
X Y
0 3.0 8.0
1 NaN NaN
2 NaN NaN
It shifts rows from bottom to top by a period of 2
.
Example Codes: DataFrame.shift()
Function to Shift Along Column
If we want to shift the column axis, we set axis=1
in the shift()
method.
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 3,],
'Y': [4, 1, 8]})
print("Original DataFrame:")
print(df)
shifted_df=df.shift(periods=1,axis=1)
print("Shifted DataFrame")
print(shifted_df)
Output:
Original DataFrame:
X Y
0 1 4
1 2 1
2 3 8
Shifted DataFrame
X Y
0 NaN 1.0
1 NaN 2.0
2 NaN 3.0
Here, we set the value of periods
to 1
, and this shifts the columns of DataFrame
from the left towards the right by 1
unit.
If we want to shift the column axis from right towards the left, we set a negative value for the periods
parameter.
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 3,],
'Y': [4, 1, 8]})
print("Original DataFrame:")
print(df)
shifted_df=df.shift(periods=-1,axis=1)
print("Shifted DataFrame")
print(shifted_df)
Output:
Original DataFrame:
X Y
0 1 4
1 2 1
2 3 8
Shifted DataFrame
X Y
0 4.0 NaN
1 1.0 NaN
2 8.0 NaN
It shifts columns from right to left by the period of 1
.
Example Codes: DataFrame.shift
Method With fill_value
Parameter
In the previous examples, the missing values after shifting are filled with NaN
by default. We can also fill the missing values with other values rather than NaN
by using the fill_value
parameter.
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 3,],
'Y': [4, 1, 8]})
print("Original DataFrame:")
print(df)
shifted_df=df.shift(periods=-1,
axis=1,
fill_value=4)
print("Shifted DataFrame")
print(shifted_df)
Output:
Original DataFrame:
X Y
0 1 4
1 2 1
2 3 8
Shifted DataFrame
X Y
0 4 4
1 1 4
2 8 4
It fills all the missing values created by the shift()
method with 4
.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn