NumPy numpy.median Function
-
Syntax of
numpy.median()
: -
Example Codes:
numpy.median()
Method to Find Median of an Array -
Example Codes: Set
axis
Parameter innumpy.median()
Method to Find Median of an Array Along Particular Axis -
Example Codes: Set
out
Parameter innumpy.median()
Method -
Example Codes: Set
keepdims
Parameter innumpy.median()
Method
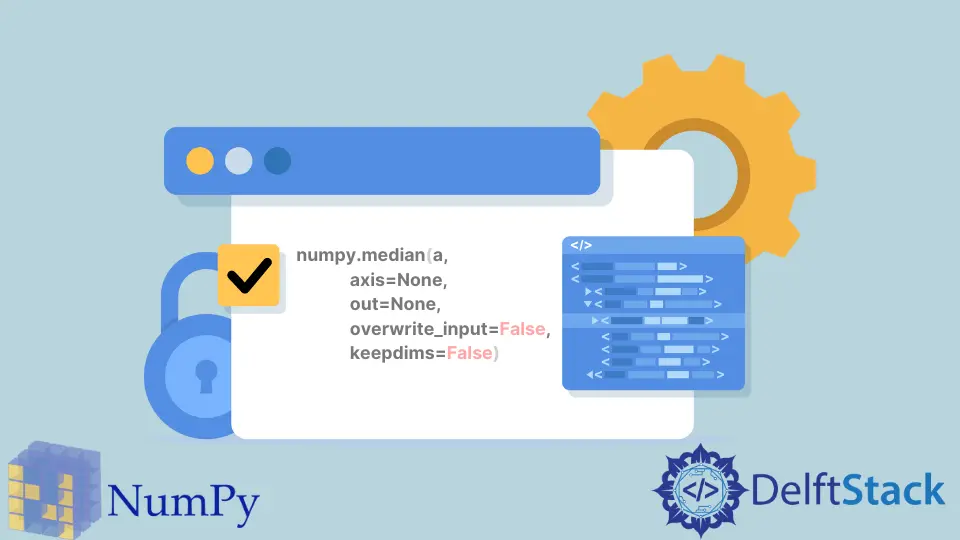
Python Numpynumpy.median()
calculates the median of the given NumPy array over the specified axis.
Syntax of numpy.median()
:
numpy.median(a, axis=None, out=None, overwrite_input=False, keepdims=False)
Parameters
a |
Array or Object, which could be converted into an array whose median is to be calculated. |
axis |
find median along the row (axis=0) or column (axis=1). By default, the median is calculated by flattening the array. |
out |
placeholder for the result of the np.median() method |
overwrite_input |
Boolean. The input array will be modified by the call to the median() method (overwrite_input=True ) |
keepdims |
Boolean. Make the dimensions of the output the same as the input (keepdims=True ). |
Return
Array with medians along the specified axis.
Example Codes: numpy.median()
Method to Find Median of an Array
import numpy as np
a=np.array([[2,3,4],
[5,6,7],
[8,9,10]])
median=np.median(a)
print(median)
Output:
6.0
It calculates the median of the array by flattening the array.
By flattening the array, we mean placing all the rows one after another to convert the given array to a 1-D array.
Example Codes: Set axis
Parameter in numpy.median()
Method to Find Median of an Array Along Particular Axis
Example Codes: numpy.median()
Method to Find Median of an Array Along Column Axis
To find the mean of the array along the column axis, we set axis=0
.
import numpy as np
a=np.array([[2,3],
[5,6],
[8,9]])
median=np.median(a,axis=0)
print(median)
Output:
[5. 6.]
It calculates the median for both the columns and finally returns an array with each column’s median.
Example Codes: numpy.median()
Method to Find Median of an Array Along Row Axis
To find the median of the array along the row axis, we set axis=1
.
import numpy as np
a=np.array([[2,3],
[5,6],
[8,9]])
median=np.median(a,axis=1)
print(median)
Output:
[2.5 5.5 8.5]
It calculates the median for all three rows and finally returns an array with each row’s median.
Example Codes: Set out
Parameter in numpy.median()
Method
import numpy as np
a = np.array([[2, 3], [5, 6], [8, 9]])
median = np.zeros(np.median(a, axis=1).shape)
print(f"median before calculation: {median}")
np.median(a, axis=1, out=median)
print(f"median after calculation: {median}")
Output:
[2.5 5.5 8.5]
It saves the result of the method in the median
variable.
We must ensure that the variable’s dimension to which output is to be assigned is of the same size as that of output.
Example Codes: Set keepdims
Parameter in numpy.median()
Method
import numpy as np
a = np.array([[2, 3], [5, 6], [8, 9]])
print(f"Dimension of Input Array: {median.ndim}")
median = np.median(a, axis=1)
print(f"Dimension of median with 'keepdims=False': {median.ndim}")
median = np.median(a, axis=1, keepdims=True)
print(f"Dimension of median with 'keepdims=True': {median.ndim}")
Output:
Dimension of Input Array: 2
Dimension of median with 'keepdims=False': 1
Dimension of median with 'keepdims=True': 2
Setting keepdims=True
preserves the number of dimensions in the output array.
Here, input array a
is 2-dimensional. If keepdims=False
(default value), the dimensions of median
might get altered but setting keepdims=True
preserves the number of dimensions in output of np.median()
method.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn