在 Python 中漂亮地列印 XML 輸出
Vaibhav Vaibhav
2024年2月16日
Python
Python XML
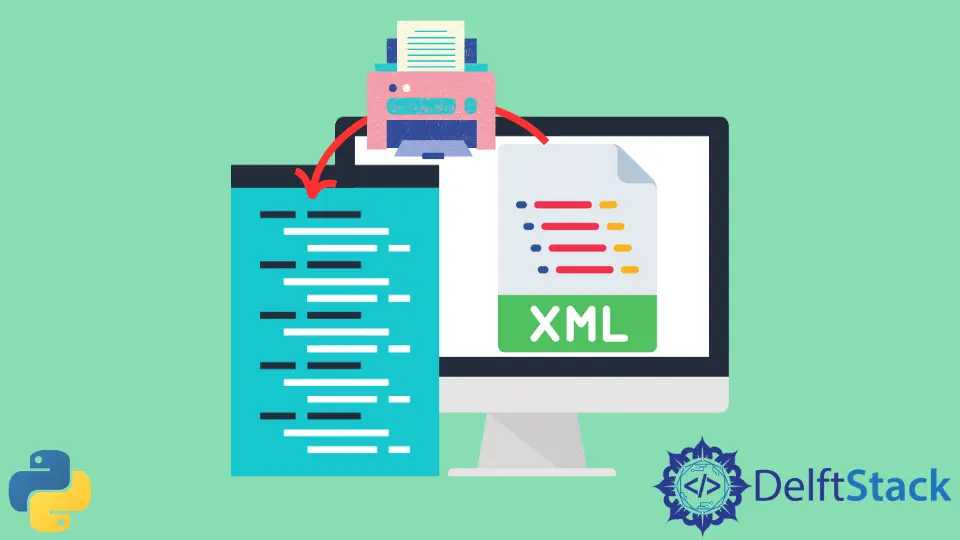
在讀取文字檔案、HTML 檔案、XML 檔案等時,檔案的內容結構很差,並且包含不一致的縮排。這種差異使得難以理解輸出。這個問題可以通過美化此類檔案的輸出來解決。美化包括修復不一致的縮排、去除隨機空格等。
在本文中,我們將學習如何使 XML 檔案輸出更漂亮。為了讓我們都在同一個頁面上,以下 Python 程式碼將考慮此 XML 檔案。如果你希望使用相同的檔案,你只需將 XML 內容複製到名為 books.xml
的新檔案中。
在 Python 中使用 BeautifulSoap
庫製作漂亮的 XML 輸出
BeautifulSoup
是一個基於 Python 的庫,用於解析 HTML 和 XML 檔案。它通常用於從網站和文件中抓取資料。我們可以使用這個庫來美化 XML 文件的輸出。
如果你的機器上沒有安裝此庫,請使用以下任一 pip
命令。
pip install beautifulsoup4
pip3 install beautifulsoup4
我們必須安裝另外兩個模組才能使用這個庫:html5lib
和 lxml
。html5lib
庫是一個基於 Python 的庫,用於解析 HTML 或超文字標記語言文件。而且,lxml
庫是處理 XML 檔案的實用程式的集合。使用以下 pip
命令在你的機器上安裝這些庫。
pip install html5lib
pip install lxml
請參考以下 Python 程式碼瞭解用法。
from bs4 import BeautifulSoup
filename = "./books.xml"
bs = BeautifulSoup(open(filename), "xml")
xml_content = bs.prettify()
print(xml_content)
輸出:
<?xml version="1.0" encoding="utf-8"?>
<catalog>
<book id="bk101">
<author>
Gambardella, Matthew
</author>
<title>
XML Developer's Guide
</title>
<genre>
Computer
</genre>
<price>
44.95
</price>
<publish_date>
2000-10-01
</publish_date>
<description>
An in-depth look at creating applications
with XML.
</description>
</book>
...
<book id="bk111">
<author>
O'Brien, Tim
</author>
<title>
MSXML3: A Comprehensive Guide
</title>
<genre>
Computer
</genre>
<price>
36.95
</price>
<publish_date>
2000-12-01
</publish_date>
<description>
The Microsoft MSXML3 parser is covered in
detail, with attention to XML DOM interfaces, XSLT processing,
SAX and more.
</description>
</book>
<book id="bk112">
<author>
Galos, Mike
</author>
<title>
Visual Studio 7: A Comprehensive Guide
</title>
<genre>
Computer
</genre>
<price>
49.95
</price>
<publish_date>
2001-04-16
</publish_date>
<description>
Microsoft Visual Studio 7 is explored in depth,
looking at how Visual Basic, Visual C++, C#, and ASP+ are
integrated into a comprehensive development
environment.
</description>
</book>
</catalog>
上面的程式碼認為 books.xml
檔案位於當前工作目錄中。或者,我們也可以在 filename
變數中設定檔案的完整路徑。prettify()
函式美化了 XML 檔案的輸出。請注意,為了便於理解,已對輸出進行了修剪。
在 Python 中使用 lxml
庫使 XML 輸出漂亮
我們可以單獨使用 lxml
庫來美化 XML 檔案的輸出。請參閱以下 Python 程式碼。
from lxml import etree
filename = "./books.xml"
f = etree.parse(filename)
content = etree.tostring(f, pretty_print=True, encoding=str)
print(content)
輸出:
<?xml version="1.0" encoding="utf-8"?>
<catalog>
<book id="bk101">
<author>
Gambardella, Matthew
</author>
<title>
XML Developer's Guide
</title>
<genre>
Computer
</genre>
<price>
44.95
</price>
<publish_date>
2000-10-01
</publish_date>
<description>
An in-depth look at creating applications
with XML.
</description>
</book>
...
<book id="bk111">
<author>
O'Brien, Tim
</author>
<title>
MSXML3: A Comprehensive Guide
</title>
<genre>
Computer
</genre>
<price>
36.95
</price>
<publish_date>
2000-12-01
</publish_date>
<description>
The Microsoft MSXML3 parser is covered in
detail, with attention to XML DOM interfaces, XSLT processing,
SAX and more.
</description>
</book>
<book id="bk112">
<author>
Galos, Mike
</author>
<title>
Visual Studio 7: A Comprehensive Guide
</title>
<genre>
Computer
</genre>
<price>
49.95
</price>
<publish_date>
2001-04-16
</publish_date>
<description>
Microsoft Visual Studio 7 is explored in depth,
looking at how Visual Basic, Visual C++, C#, and ASP+ are
integrated into a comprehensive development
environment.
</description>
</book>
</catalog>
注意 pretty_print = True
引數。這個標誌使輸出更漂亮。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Vaibhav Vaibhav