Python で XML 出力をきれいに印刷する
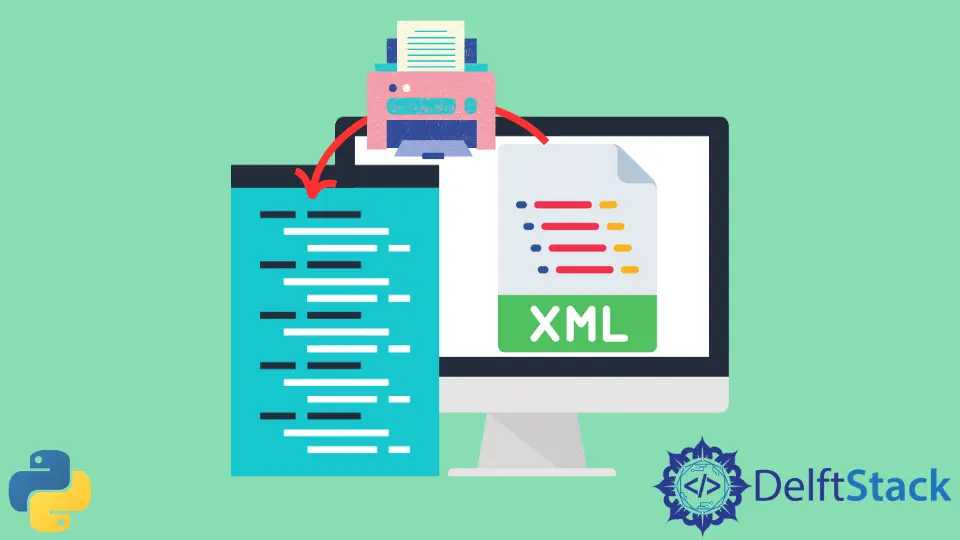
テキストファイル、HTML ファイル、XML ファイルなどを読み取る場合、ファイルのコンテンツの構造が不十分で、一貫性のないインデントが含まれています。この不一致により、出力を理解することが困難になります。この問題は、そのようなファイルの出力を美化することで解決できます。美化には、一貫性のないインデントの修正、ランダムなスペースの削除などが含まれます。
この記事では、XML ファイルの出力をより美しくする方法を学びます。以下の Python コードは、ここを考慮しています。同じファイルを使用したい場合は、XML コンテンツを books.xml
という名前の新しいファイルにコピーするだけです。
Python で BeautifulSoap
ライブラリを使用して XML 出力をきれいにする
BeautifulSoup
は、HTML および XML ファイルを解析するための Python ベースのライブラリです。これは通常、Web サイトやドキュメントからデータをスクレイピングするために使用されます。このライブラリを使用して、XML ドキュメントの出力を美化することができます。
このライブラリがマシンにインストールされていない場合は、次の pip
コマンドのいずれかを使用してください。
pip install beautifulsoup4
pip3 install beautifulsoup4
このライブラリを使用するには、さらに 2つのモジュール html5lib
と lxml
をインストールする必要があります。html5lib
ライブラリは、HTML またはハイパーテキストマークアップ言語ドキュメントを解析するための Python ベースのライブラリです。また、lxml
ライブラリは、XML ファイルを操作するためのユーティリティのコレクションです。次の pip
コマンドを使用して、これらのライブラリをマシンにインストールします。
pip install html5lib
pip install lxml
使用法を理解するには、次の Python コードを参照してください。
from bs4 import BeautifulSoup
filename = "./books.xml"
bs = BeautifulSoup(open(filename), "xml")
xml_content = bs.prettify()
print(xml_content)
出力:
<?xml version="1.0" encoding="utf-8"?>
<catalog>
<book id="bk101">
<author>
Gambardella, Matthew
</author>
<title>
XML Developer's Guide
</title>
<genre>
Computer
</genre>
<price>
44.95
</price>
<publish_date>
2000-10-01
</publish_date>
<description>
An in-depth look at creating applications
with XML.
</description>
</book>
...
<book id="bk111">
<author>
O'Brien, Tim
</author>
<title>
MSXML3: A Comprehensive Guide
</title>
<genre>
Computer
</genre>
<price>
36.95
</price>
<publish_date>
2000-12-01
</publish_date>
<description>
The Microsoft MSXML3 parser is covered in
detail, with attention to XML DOM interfaces, XSLT processing,
SAX and more.
</description>
</book>
<book id="bk112">
<author>
Galos, Mike
</author>
<title>
Visual Studio 7: A Comprehensive Guide
</title>
<genre>
Computer
</genre>
<price>
49.95
</price>
<publish_date>
2001-04-16
</publish_date>
<description>
Microsoft Visual Studio 7 is explored in depth,
looking at how Visual Basic, Visual C++, C#, and ASP+ are
integrated into a comprehensive development
environment.
</description>
</book>
</catalog>
上記のコードは、books.xml
ファイルが現在の作業ディレクトリにあると見なします。または、filename
変数でファイルへのフルパスを設定することもできます。prettify()
関数は、XML ファイルの出力を美化します。理解を深めるために、出力がトリミングされていることに注意してください。
Python で lxml
ライブラリを使用して XML 出力をきれいにする
lxml
ライブラリを単独で使用して、XML ファイルの出力を美化することができます。同じことについては、次の Python コードを参照してください。
from lxml import etree
filename = "./books.xml"
f = etree.parse(filename)
content = etree.tostring(f, pretty_print=True, encoding=str)
print(content)
出力:
<?xml version="1.0" encoding="utf-8"?>
<catalog>
<book id="bk101">
<author>
Gambardella, Matthew
</author>
<title>
XML Developer's Guide
</title>
<genre>
Computer
</genre>
<price>
44.95
</price>
<publish_date>
2000-10-01
</publish_date>
<description>
An in-depth look at creating applications
with XML.
</description>
</book>
...
<book id="bk111">
<author>
O'Brien, Tim
</author>
<title>
MSXML3: A Comprehensive Guide
</title>
<genre>
Computer
</genre>
<price>
36.95
</price>
<publish_date>
2000-12-01
</publish_date>
<description>
The Microsoft MSXML3 parser is covered in
detail, with attention to XML DOM interfaces, XSLT processing,
SAX and more.
</description>
</book>
<book id="bk112">
<author>
Galos, Mike
</author>
<title>
Visual Studio 7: A Comprehensive Guide
</title>
<genre>
Computer
</genre>
<price>
49.95
</price>
<publish_date>
2001-04-16
</publish_date>
<description>
Microsoft Visual Studio 7 is explored in depth,
looking at how Visual Basic, Visual C++, C#, and ASP+ are
integrated into a comprehensive development
environment.
</description>
</book>
</catalog>
pretty_print = True
引数に注意してください。このフラグにより、出力がよりきれいになります。