Impresión bonita de la salida XML en Python
-
Haga que la salida XML sea bonita en Python usando la biblioteca
BeautifulSoap
-
Haga que la salida XML sea bonita en Python usando la biblioteca
lxml
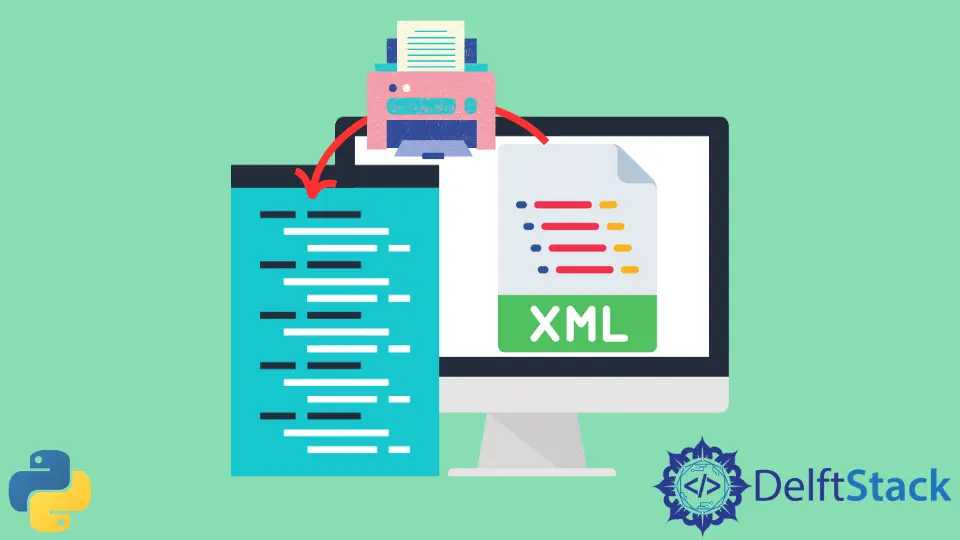
Al leer archivos de texto, archivos HTML, archivos XML, etc., el contenido de un archivo está mal estructurado y contiene sangría inconsistente. Esta disparidad dificulta la comprensión del resultado. Este problema se puede resolver embelleciendo la salida de dichos archivos. El embellecimiento incluye la reparación de sangrías inconsistentes, la eliminación de espacios aleatorios, etc.
En este artículo, aprenderemos cómo hacer que la salida de un archivo XML sea más bonita. Para que todos estemos en la misma página, los siguientes códigos de Python considerarán este archivo XML. Si desea utilizar el mismo archivo, todo lo que tiene que hacer es copiar el contenido XML en un nuevo archivo con el nombre de books.xml
.
Haga que la salida XML sea bonita en Python usando la biblioteca BeautifulSoap
BeautifulSoup
es una biblioteca basada en Python para analizar archivos HTML y XML. Generalmente se usa para extraer datos de sitios web y documentos. Podemos usar esta biblioteca para embellecer la salida de un documento XML.
En caso de que no tenga esta biblioteca instalada en su máquina, use cualquiera de los siguientes comandos pip
.
pip install beautifulsoup4
pip3 install beautifulsoup4
Tenemos que instalar dos módulos más para trabajar con esta librería: html5lib
y lxml
. La biblioteca html5lib
es una biblioteca basada en Python para analizar documentos HTML o Hypertext Markup Language. Y, la biblioteca lxml
es una colección de utilidades para trabajar con archivos XML. Use los siguientes comandos pip
para instalar estas bibliotecas en su máquina.
pip install html5lib
pip install lxml
Consulte el siguiente código de Python para comprender el uso.
from bs4 import BeautifulSoup
filename = "./books.xml"
bs = BeautifulSoup(open(filename), "xml")
xml_content = bs.prettify()
print(xml_content)
Producción :
<?xml version="1.0" encoding="utf-8"?>
<catalog>
<book id="bk101">
<author>
Gambardella, Matthew
</author>
<title>
XML Developer's Guide
</title>
<genre>
Computer
</genre>
<price>
44.95
</price>
<publish_date>
2000-10-01
</publish_date>
<description>
An in-depth look at creating applications
with XML.
</description>
</book>
...
<book id="bk111">
<author>
O'Brien, Tim
</author>
<title>
MSXML3: A Comprehensive Guide
</title>
<genre>
Computer
</genre>
<price>
36.95
</price>
<publish_date>
2000-12-01
</publish_date>
<description>
The Microsoft MSXML3 parser is covered in
detail, with attention to XML DOM interfaces, XSLT processing,
SAX and more.
</description>
</book>
<book id="bk112">
<author>
Galos, Mike
</author>
<title>
Visual Studio 7: A Comprehensive Guide
</title>
<genre>
Computer
</genre>
<price>
49.95
</price>
<publish_date>
2001-04-16
</publish_date>
<description>
Microsoft Visual Studio 7 is explored in depth,
looking at how Visual Basic, Visual C++, C#, and ASP+ are
integrated into a comprehensive development
environment.
</description>
</book>
</catalog>
El código anterior considera que el archivo books.xml
está en el directorio de trabajo actual. O bien, también podemos establecer la ruta completa al archivo en la variable filename
. La función prettify()
embellece la salida del archivo XML. Tenga en cuenta que la salida se ha recortado para fines de comprensión.
Haga que la salida XML sea bonita en Python usando la biblioteca lxml
Podemos usar la biblioteca lxml
sola para embellecer la salida de un archivo XML. Consulte el siguiente código de Python para lo mismo.
from lxml import etree
filename = "./books.xml"
f = etree.parse(filename)
content = etree.tostring(f, pretty_print=True, encoding=str)
print(content)
Producción :
<?xml version="1.0" encoding="utf-8"?>
<catalog>
<book id="bk101">
<author>
Gambardella, Matthew
</author>
<title>
XML Developer's Guide
</title>
<genre>
Computer
</genre>
<price>
44.95
</price>
<publish_date>
2000-10-01
</publish_date>
<description>
An in-depth look at creating applications
with XML.
</description>
</book>
...
<book id="bk111">
<author>
O'Brien, Tim
</author>
<title>
MSXML3: A Comprehensive Guide
</title>
<genre>
Computer
</genre>
<price>
36.95
</price>
<publish_date>
2000-12-01
</publish_date>
<description>
The Microsoft MSXML3 parser is covered in
detail, with attention to XML DOM interfaces, XSLT processing,
SAX and more.
</description>
</book>
<book id="bk112">
<author>
Galos, Mike
</author>
<title>
Visual Studio 7: A Comprehensive Guide
</title>
<genre>
Computer
</genre>
<price>
49.95
</price>
<publish_date>
2001-04-16
</publish_date>
<description>
Microsoft Visual Studio 7 is explored in depth,
looking at how Visual Basic, Visual C++, C#, and ASP+ are
integrated into a comprehensive development
environment.
</description>
</book>
</catalog>
Toma nota del argumento pretty_print = True
. Esta bandera hace que la salida sea más bonita.