在 Python 中建立 XML 解析器
-
在 Python 中使用
ElementTree
API 解析 XML 文件 -
在 Python 中使用
minidom
模組解析 XML 文件 -
在 Python 中使用
Beautiful Soup
庫解析 XML 文件 -
在 Python 中使用
xmltodict
庫解析 XML 文件 -
在 Python 中使用
lxml
庫解析 XML 文件 -
在 Python 中使用
untangle
模組解析 XML 文件 -
在 Python 中使用
declxml
庫解析 XML 文件
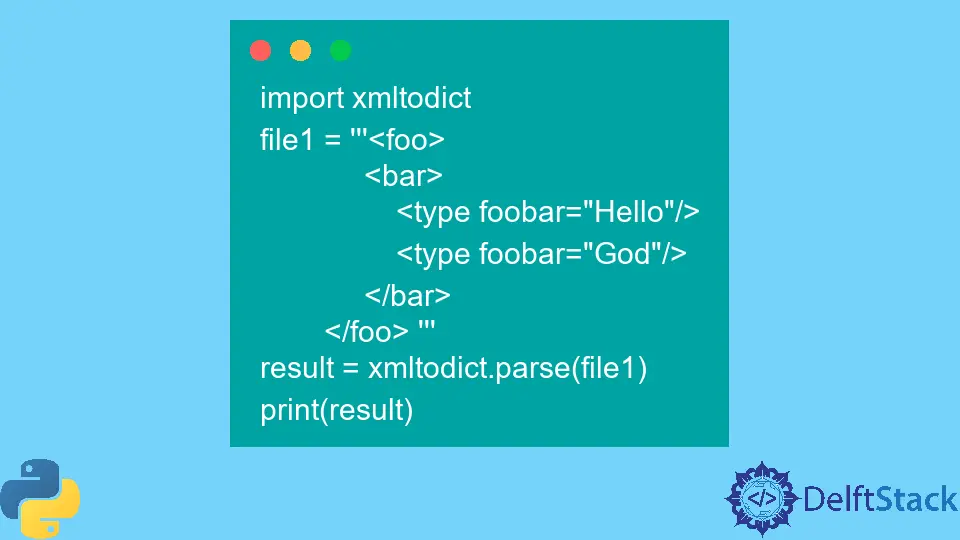
XML 是可擴充套件標記語言的縮寫,是一種用於儲存和傳輸資料的自描述語言。Python 為解析和修改 XML 文件提供了一種媒介。
本教程重點介紹並演示了在 Python 中解析 XML 文件的不同方法。
在 Python 中使用 ElementTree
API 解析 XML 文件
xml.etree.ElementTree
模組用於生成高效而簡單的 API 來解析 XML 文件並建立 XML 資料。
以下程式碼使用 xml.etree.ElementTree
模組在 Python 中解析 XML 文件。
# >= Python 3.3 code
import xml.etree.ElementTree as ET
file1 = """<foo>
<bar>
<type foobar="Hello"/>
<type foobar="God"/>
</bar>
</foo>"""
tree = ET.fromstring(file1)
x = tree.findall("bar/type")
for item in x:
print(item.get("foobar"))
輸出:
Hello
God
在這裡,我們將 XML 資料作為三引號內的字串傳遞。我們還可以在 ElementTree
模組的 parse()
函式的幫助下匯入一個實際的 XML 文件。
cElementTree
模組是 ElementTree
API 的 C 實現,唯一的區別是優化了 cElementTree
。話雖如此,它的解析速度比 ElementTree
模組快 15-20 倍,並且使用的記憶體量非常低。
但是,在 Python 3.3 及更高版本中,cElementTree
模組已被棄用,而 ElementTree
模組使用更快的實現。
在 Python 中使用 minidom
模組解析 XML 文件
xml.dom.minidom
可以定義為文件物件模型 (DOM) 介面的基本實現。所有 DOM 應用程式通常都從解析 XML 物件開始。因此,該方法是 Python 中解析 XML 文件最快的方法。
以下程式碼使用 minidom
模組中的 parse()
函式來解析 Python 中的 XML 文件。
XML 檔案 (sample1.xml):
<data>
<strings>
<string name="Hello"></string>
<string name="God"></string>
</strings>
</data>
Python 程式碼:
from xml.dom import minidom
xmldoc = minidom.parse("sample1.xml")
stringlist = xmldoc.getElementsByTagName("string")
print(len(stringlist))
print(stringlist[0].attributes["name"].value)
for x in stringlist:
print(x.attributes["name"].value)
輸出:
2
Hello
God
該模組還允許將 XML 作為字串傳遞,類似於 ElementTree
API。然而,它使用 parseString()
函式來實現這一點。
據說 xml.etree.ElementTree
和 xml.dom.minidom
模組對於惡意構造的資料都是不安全的。
在 Python 中使用 Beautiful Soup
庫解析 XML 文件
Beautiful Soup
庫是為網頁抓取專案和從 XML
和 HTML
檔案中提取資料而設計的。美麗的湯
速度非常快,可以解析它遇到的任何東西。
該庫甚至為程式執行樹遍歷過程並解析 XML 文件。此外,Beautiful Soup
還用於美化給定的原始碼。
Beautiful Soup
庫需要手動安裝,然後匯入到此方法的 Python 程式碼中。這個庫可以使用 pip
命令安裝。Beautiful Soup 4
庫是最新版本,適用於 Python 2.7 及更高版本。
以下程式碼使用 Beautiful Soup
庫在 Python 中解析 XML 文件。
from bs4 import BeautifulSoup
file1 = """<foo>
<bar>
<type foobar="Hello"/>
<type foobar="God"/>
</bar>
</foo>"""
a = BeautifulSoup(file1)
print(a.foo.bar.type["foobar"])
print(a.foo.bar.findAll("type"))
輸出:
u'Hello'
[<type foobar="Hello"></type>, <type foobar="God"></type>]
Beautiful Soup
比任何其他用於解析的工具都要快,但有時可能很難理解和實現這種方法。
在 Python 中使用 xmltodict
庫解析 XML 文件
xmltodict
庫有助於使處理 XML 檔案的過程類似於 JSON。它也可以用於我們想要解析 XML 檔案的情況。在這種情況下,可以通過將 XML 檔案解析為 Ordered Dictionary
來使用 xmltodict
模組。
xmltodict
庫需要手動安裝,然後匯入到包含 XML 檔案的 Python 程式碼中。xmltodict
的安裝非常基本,可以使用標準的 pip
命令完成。
以下程式碼使用 xmltodict
庫來解析 Python 中的 XML 文件。
import xmltodict
file1 = """<foo>
<bar>
<type foobar="Hello"/>
<type foobar="God"/>
</bar>
</foo> """
result = xmltodict.parse(file1)
print(result)
輸出:
OrderedDict([(u'foo', OrderedDict([(u'bar', OrderedDict([(u'type', [OrderedDict([(u'@foobar', u'Hello')]), OrderedDict([(u'@foobar', u'God')])])]))]))])
在 Python 中使用 lxml
庫解析 XML 文件
lxml
庫能夠在 Python 中提供一個簡單但非常強大的 API,用於解析 XML 和 HTML 檔案。它結合了 ElementTree
API 和 libxml2/libxslt
。
簡單來說,lxml
庫進一步擴充套件了舊的 ElementTree
庫,以提供對 XML Schema、XPath 和 XSLT 等新事物的支援。
在這裡,我們將使用 lxml.objectify
庫。以下程式碼使用 lxml
庫在 Python 中解析 XML 文件。
from collections import defaultdict
from lxml import objectify
file1 = """<foo>
<bar>
<type foobar="1"/>
<type foobar="2"/>
</bar>
</foo>"""
c = defaultdict(int)
root = objectify.fromstring(file1)
for item in root.bar.type:
c[item.attrib.get("foobar")] += 1
print(dict(c))
輸出:
{'1': 1, '2': 1}
在這裡,在這個程式中,c
變數用於儲存字典中每個可用專案的計數。
在 Python 中使用 untangle
模組解析 XML 文件
untangle
模組是一個易於實現的模組,專注於將 XML 轉換為 Python 物件。它也可以使用 pip
命令輕鬆安裝。此模組適用於 Python 2.7 及更高版本。
以下程式碼使用 untangle
模組在 Python 中解析 XML 文件。
XML 檔案 (sample1.xml):
<foo>
<bar>
<type foobar="Hello"/>
</bar>
</foo>
Python 程式碼:
import untangle
x = untangle.parse("/path_to_xml_file/sample1.xml")
print(x.foo.bar.type["foobar"])
輸出:
Hello
在 Python 中使用 declxml
庫解析 XML 文件
declxml
庫是 Declarative XML Processing 的縮寫,用於提供一個簡單的 API 來序列化和解析 XML 文件。該庫旨在減少程式設計師的工作量,並取代在使用其他流行的 API(例如 minidom
或 ElementTree
)時,需要處理大而長的解析邏輯程式碼塊。
declxml
模組可以通過使用 pip
或 pipenv
命令輕鬆安裝在系統中。以下程式碼使用 declxml
庫來解析 Python 中的 XML 文件。
import declxml as xml
xml_string = """
<foo>
<bar>
<type foobar="1"/>
<type foobar="3"/>
<type foobar="5"/>
</bar>
</foo>
"""
processor = xml.dictionary(
"foo", [xml.dictionary("bar", [xml.array(xml.integer("type", attribute="foobar"))])]
)
xml.parse_from_string(processor, xml_string)
輸出:
{'bar': {'foobar': [1, 3, 5]}}
在這種方法中,我們使用處理器來宣告性地表徵給定 XML 文件的結構以及 XML 和 Python 資料結構之間的對映。
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn