使用 Python 將檔案從一個目錄移動到另一個目錄
-
在 Python 中使用
shutil.move()
函式移動檔案 -
在 Python 中使用
os.rename()
或os.replace()
函式移動檔案 -
在 Python 中使用
pathlib
模組移動檔案
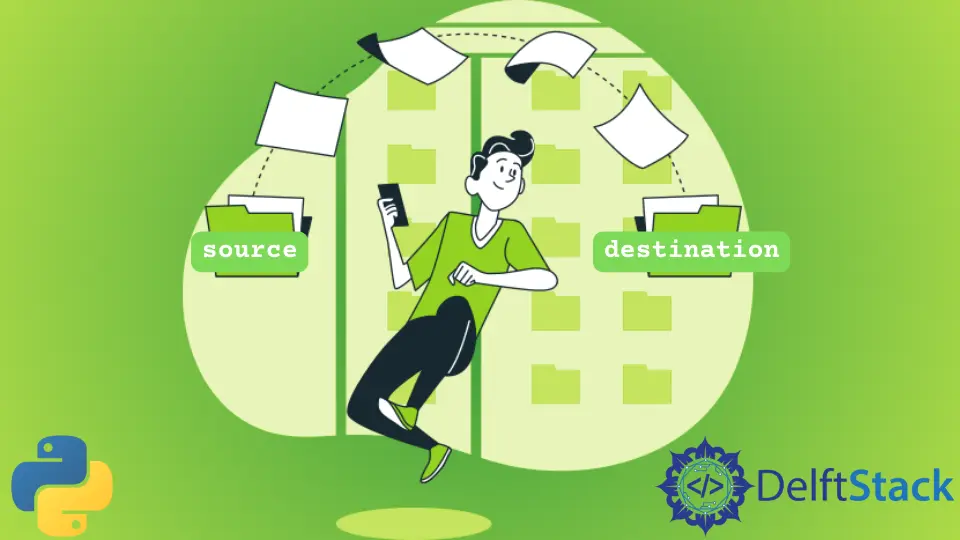
將檔案從一個目錄移動到另一個目錄可能聽起來沒什麼大不了的,但有時,它對操作檔案有很大幫助。
本教程將介紹一些在 Python 中將檔案從一個目錄移動到另一個目錄的方法。
在 Python 中使用 shutil.move()
函式移動檔案
shutil
模組是一個 Python 模組,可幫助對檔案或一組檔案進行高階操作。該模組在諸如從某處複製檔案或刪除檔案之類的操作中發揮作用。
為了在 shutil
模組的幫助下將檔案從一個目錄移動到另一個目錄,呼叫 shutil.move()
。
例子:
import shutil
import os
file_source = "Path/Of/Directory"
file_destination = "Path/Of/Directory"
get_files = os.listdir(file_source)
for g in get_files:
shutil.move(file_source + g, file_destination)
在這裡,listdir()
函式來自 os
模組,用於獲取目錄中存在的所有檔案的完整列表。我們使用 for
迴圈來移動檔案,並注意 shutil
模組的 move()
函式用於將檔案從一個目錄傳輸到另一個目錄。
在 Python 中使用 os.rename()
或 os.replace()
函式移動檔案
很多時候,使用者需要通過 Python 連線到主系統。在這種情況下,os
模組發揮作用。os
模組基本上充當使用者和計算機作業系統之間的中介,以便使用者可以正確連線作業系統。
該模組的功能之一是用於將檔案從一個位置移動到另一個位置的 rename()
模組。此函式通過重新命名這些檔案的目錄名稱來移動檔案。
該模組的另一個功能是 replace()
功能。此功能有助於重新命名檔案或當前目錄。目標必須是檔案而不是目錄。因此,如果目標是一個檔案,那麼它將被替換而不會出現任何錯誤。
總之,當檔案的最終目的地與其來源位於同一磁碟中時,將使用 rename()
函式。如果必須更改檔案的目的地,則必須使用 replace()
。
例子:
import os
file_source = "Path/Of/Directory"
file_destination = "Path/Of/Directory"
get_files = os.listdir(file_source)
for g in get_files:
os.replace(file_source + g, file_destination + g)
在這裡,我們也遵循相同的過程,首先定義初始目錄和最終目錄的路徑。然後我們使用 listdir()
函式來獲取當前目錄中所有檔案的列表。之後,我們使用 for
迴圈來覆蓋這些檔案的目的地。
在 Python 中使用 pathlib
模組移動檔案
Python 中的 pathlib
模組是一個標準模組,用於提供用於操作不同檔案和字典的物件。處理檔案的核心物件稱為路徑。
例子:
from pathlib import Path
import shutil
import os
file_source = "Path/Of/Directory"
file_destination = "Path/Of/Directory"
for file in Path(file_source).glob("randomfile.txt"):
shutil.move(os.path.join(file_source, file), file_destination)
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn相關文章 - Python File
- Python 如何得到資料夾下的所有檔案
- Python 如何查詢具有特定副檔名的檔案
- 如何在 Python 中從檔案讀取特定行
- 在 Python 中讀取文字檔案並列印其內容
- 在 Python 中逐行讀取 CSV
- 在 Python 中實現 touch 檔案