Python 字典索引
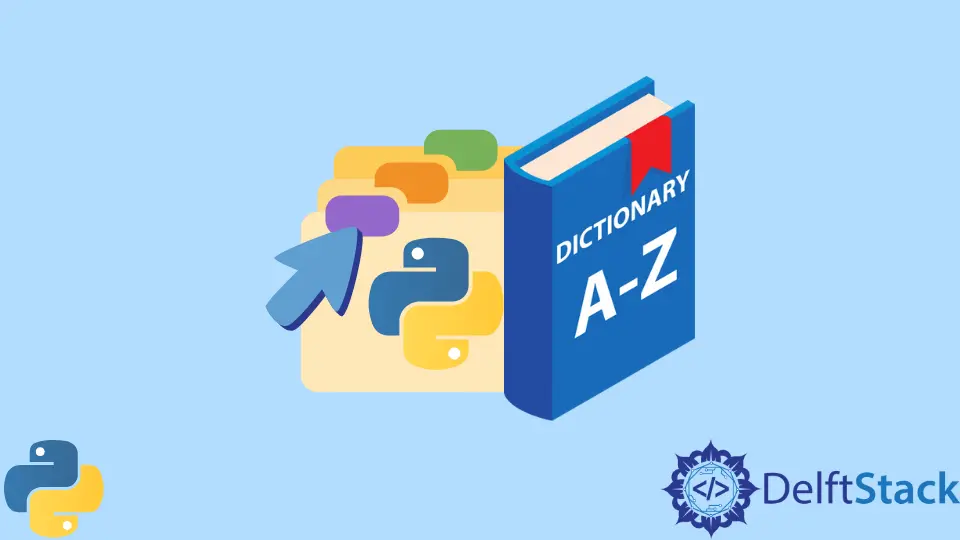
字典用於在 Python 中儲存鍵值對。通常,我們無法使用其他集合(例如列表或陣列)的元素索引來訪問字典。
在 Python 3.7 之前,字典是無序的。每個鍵值在字典中的分配順序都是隨機的。在這種情況下,我們可以使用 collections
模組中的 OrderedDict()
方法。它保留了鍵值對新增到字典的順序。
在 Python 3.7 及更高版本中,預設情況下使字典保持順序。
我們可以使用保留順序的字典中的索引來訪問鍵,值和鍵-值對。
使用索引從字典訪問鍵
我們將使用 keys()
方法,該方法返回鍵的集合。在將其轉換為列表之後,我們可以使用索引來訪問此集合中的所需鍵。
請記住,將 list()
函式與 keys()
,values()
和 items()
函式一起使用。這是因為它們不返回傳統列表,並且不允許使用索引訪問元素。
以下說明了這一點。
d = {}
d["a"] = 0
d["b"] = 1
d["c"] = 2
keys = list(d.keys())
print(keys[1])
輸出:
b
在 Python 3.7 以下工作時,請記住使用 OrderedDict()
方法建立所需字典並保持其順序。例如,
from collections import OrderedDict
d1 = OrderedDict()
d1["a"] = 0
d1["b"] = 1
d1["c"] = 2
keys = list(d1.keys())
print(keys[1])
輸出:
b
使用 Python 中的索引從字典訪問值
當我們想從字典中返回所有值的集合時,我們使用 values()
函式。
d = {}
d["a"] = 0
d["b"] = 1
d["c"] = 2
values = list(d.values())
print(values[1])
輸出:
1
使用 Python 中的索引從字典訪問鍵值對
items()
函式返回所有字典的鍵-值對的集合,每個元素儲存為一個元組。
索引可用於從列表中訪問這些對。
d = {}
d["a"] = 0
d["b"] = 1
d["c"] = 2
values = list(d.items())
print(values[1])
輸出:
('b', 1)
如果你的 Python 版本沒有保留字典的順序,請記住對所有方法都使用 OrderedDict()
函式。
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn