Python 字典索引
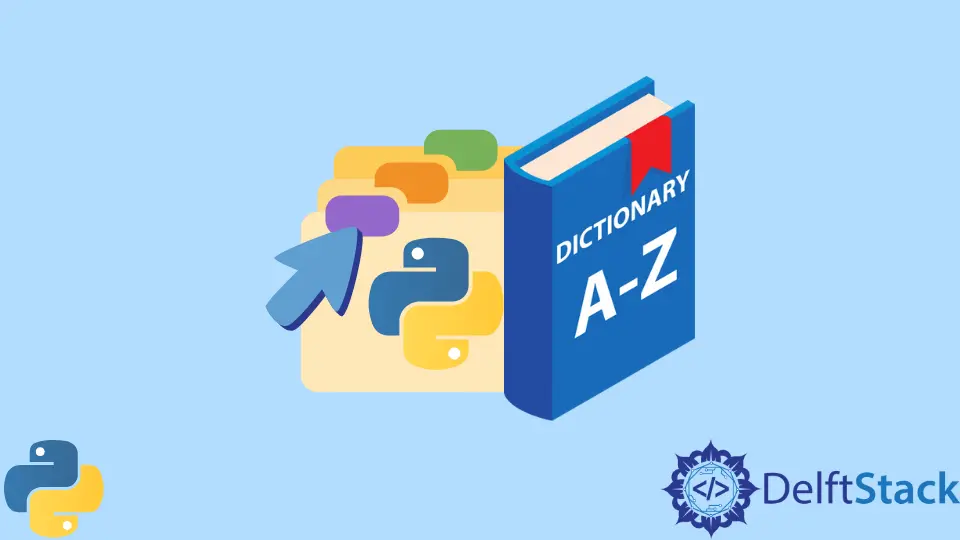
字典用于在 Python 中存储键值对。通常,我们无法使用其他集合(例如列表或数组)的元素索引来访问字典。
在 Python 3.7 之前,字典是无序的。每个键值在字典中的分配顺序都是随机的。在这种情况下,我们可以使用 collections
模块中的 OrderedDict()
方法。它保留了键值对添加到字典的顺序。
在 Python 3.7 及更高版本中,默认情况下使字典保持顺序。
我们可以使用保留顺序的字典中的索引来访问键,值和键-值对。
使用索引从字典访问键
我们将使用 keys()
方法,该方法返回键的集合。在将其转换为列表之后,我们可以使用索引来访问此集合中的所需键。
请记住,将 list()
函数与 keys()
,values()
和 items()
函数一起使用。这是因为它们不返回传统列表,并且不允许使用索引访问元素。
以下说明了这一点。
d = {}
d["a"] = 0
d["b"] = 1
d["c"] = 2
keys = list(d.keys())
print(keys[1])
输出:
b
在 Python 3.7 以下工作时,请记住使用 OrderedDict()
方法创建所需字典并保持其顺序。例如,
from collections import OrderedDict
d1 = OrderedDict()
d1["a"] = 0
d1["b"] = 1
d1["c"] = 2
keys = list(d1.keys())
print(keys[1])
输出:
b
使用 Python 中的索引从字典访问值
当我们想从字典中返回所有值的集合时,我们使用 values()
函数。
d = {}
d["a"] = 0
d["b"] = 1
d["c"] = 2
values = list(d.values())
print(values[1])
输出:
1
使用 Python 中的索引从字典访问键值对
items()
函数返回所有字典的键-值对的集合,每个元素存储为一个元组。
索引可用于从列表中访问这些对。
d = {}
d["a"] = 0
d["b"] = 1
d["c"] = 2
values = list(d.items())
print(values[1])
输出:
('b', 1)
如果你的 Python 版本没有保留字典的顺序,请记住对所有方法都使用 OrderedDict()
函数。
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn