檢查 Pandas 中是否存在列
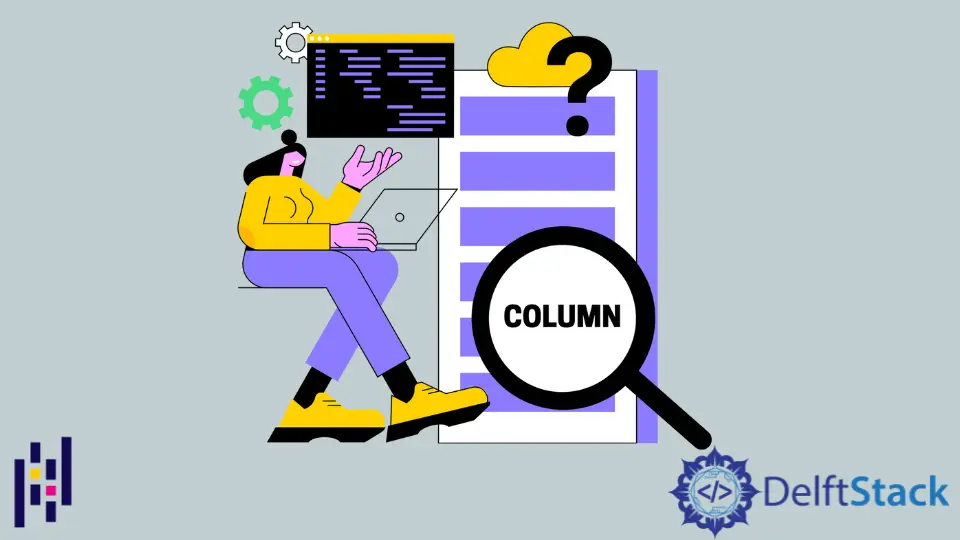
本教程演示了檢查 Python 中是否存在 Pandas Dataframe 中的列的方法。我們將在 Python 中使用可用於執行此操作的 IN
和 NOT IN
運算子。
使用 IN
運算子檢查 Pandas 中是否存在列
Dataframe 是一種儲存二維資料及其相應標籤的排列。我們可以使用 dataframe.column
屬性找到列標籤。
為了確保列是否存在,我們使用 IN
表示式。但是,在開始之前,我們需要在 Pandas 中形成一個虛擬 DataFrame 以使用上述技術。
在這裡,我們建立了一個學生表現的 DataFrame,列名稱為 Name
、Promoted
和 Marks
。
import pandas as pd
import numpy as np
# Creating dataframe
df = pd.DataFrame()
# Adding columns to the dataframe
df["Name"] = ["John", "Doe", "Bill"]
df["Promoted"] = [True, False, True]
df["Marks"] = [82, 38, 63]
# Getting the dataframe as an output
print(df)
程式碼給出以下輸出。
Name Promoted Marks
0 John True 82
1 Doe False 38
2 Bill True 63
DataFrame 準備好後,我們可以通過編寫下面給出的程式碼來檢查 DataFrame 是否包含專案或為空。為此,我們可以使用兩種方法。
我們可以使用 Pandas 中存在的 df.empty
函式,或者我們可以使用 len(df.index)
檢查 DataFrame 的長度。
我們在下面的示例中使用了 Pandas 屬性 df.empty
。
if df.empty:
print("DataFrame is empty!")
else:
print("Not empty!")
由於我們已將資料插入列中,因此輸出必須為 Not empty!
。
Not empty!
現在,讓我們繼續使用 IN
方法檢查 Pandas DataFrame 中的列是否存在。請參閱下面的程式碼以檢視此功能的執行情況。
if "Promoted" in df:
print("Yes, it does exist.")
else:
print("No, it does not exist.")
程式碼給出以下輸出。
Yes, it does exist.
為了更清楚起見,也可以將其寫成 if 'Promoted' in df.columns:
,而不是僅僅寫成 df
。
使用 NOT IN
運算子檢查 Pandas 中是否存在列
讓我們看看如何使用 NOT IN
屬性來執行相同的操作。它以相反的方式執行,並且由於屬性中新增了否定,輸出被反轉。
這是下面給出的 NOT IN
屬性的示例工作。
if "Promoted" not in df.columns:
print("Yes, it does not exist.")
else:
print("No, it does exist.")
程式碼給出以下輸出。
No, it does exist.
我們已經看到如何對 DataFrame 中的單個列執行此操作。Pandas 還使使用者能夠檢查 DataFrame 中的多個列。
這有助於快速完成任務並有助於同時對多個列進行分類。
下面是檢查 Pandas DataFrame 中多列的程式碼片段。
if set(["Name", "Promoted"]).issubset(df.columns):
print("Yes, all of them exist.")
else:
print("No")
程式碼給出以下輸出。
Yes, all of them exist.
set([])
也可以使用花括號來構造。
if not {"Name", "Promoted"}.issubset(df.columns):
print("Yes")
else:
print("No")
輸出將是:
No
這些是檢查資料中的一列或多列的可能方法。同樣,我們也可以對現成的資料而不是虛擬資料執行這些功能。
我們只需要通過 read_csv
方法使用 Python Pandas 模組匯入 CSV 檔案。如果使用 Google Colab,請從 google.colab
匯入 files
模組,以便在執行時從個人系統上傳資料檔案。