在 Pandas 的列中展平層次索引
Luqman Khan
2023年1月30日
Pandas
Pandas Column
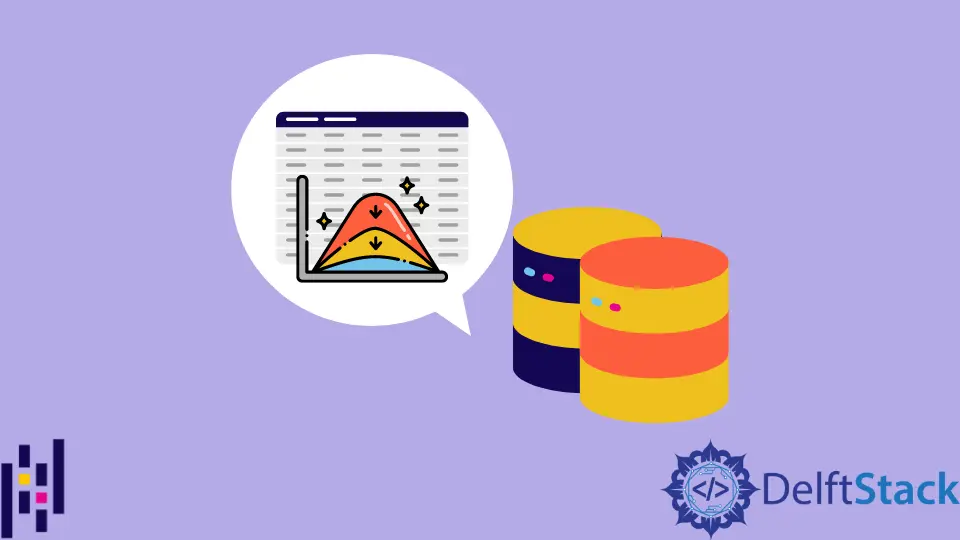
本文將討論如何在 Pandas Dataframe 列中展平分層索引。
Groupby 聚合函式通常用於建立分層索引。使用的聚合函式將在結果 DataFrame 的分層索引中可見。
我們將使用不同的函式來解釋如何在列中展平層次索引。
使用 rest_index()
在 Pandas 的列中展平層次索引
Pandas 中的 reset_index()
函式將 groupby 聚合函式建立的分層索引展平。
語法:
pandas.DataFrame.reset_index(level, drop, inplace)
其中,
level
:僅從索引中刪除指示的級別。drop
:索引重置為預設整數索引。inplace
:不復制,永久修改 DataFrame 物件。
我們使用 Pandas groupby()
函式按季度對巴士銷售資料進行分組,並使用 reset_index()
pandas 函式來展平分組 DataFrame 的分層索引列。
首先,匯入 Python Pandas 庫,然後建立一個簡單的 DataFrame。DataFrame 儲存在 data_bus
變數中。
import pandas as pd
data_bus = pd.DataFrame(
{
"bus": ["2x", "3Tr", "4x", "5x"],
"bus_sale_q1": [21, 23, 25, 27],
"bus_sale_q2": [12, 14, 16, 18],
},
columns=["bus", "bus_sale_q1", "bus_sale_q2"],
)
print(data_bus)
輸出:
bus bus_sale_q1 bus_sale_q2
0 2x 21 12
1 3Tr 23 14
2 4x 25 16
3 5x 27 18
上面的輸出顯示了建立的簡單 DataFrame。之後,使用 groupby()
函式根據銷售額 q1 和 q2 的總和對匯流排列進行分組。
grouped_data = data_bus.groupby(by="bus").agg("sum")
grouped_data
輸出:
bus bus_sale_q1 bus_sale_q2
2x 21 12
3Tr 23 14
4x 25 16
5x 27 18
我們將使用 reset_index()
函式來展平分層索引列。
flat_data = grouped_data.reset_index()
flat_data
輸出:
bus bus_sale_q1 bus_sale_q2
0 2x 21 12
1 3Tr 23 14
2 4x 25 16
3 5x 27 18
使用 as_index
在 Pandas 的列中展平層次索引
pandas groupby()
函式將用於按季度對巴士銷售資料進行分組,而 as_index
將展平分組 DataFrame 的分層索引列。
語法:
pandas.DataFrame.groupby(by, level, axis, as_index)
其中,
level
:必須對其執行 groupby 操作的列。by
:必須對其執行 groupby 操作的列。axis
:是否沿行 (0) 或列 (1) 拆分。as_index
:對於聚合輸出,返回帶有索引組標籤的物件。
我們將使用 Pandas groupby()
函式按季度對巴士銷售資料進行分組,並將 as_index
引數設定為 False。這確保了分組 DataFrame 的分層索引是扁平的。
將使用與上一個示例相同的 DataFrame。
例子:
import pandas as pd
data_bus = pd.DataFrame(
{
"bus": ["2x", "3Tr", "4x", "5x"],
"bus_sale_q1": [21, 23, 25, 27],
"bus_sale_q2": [12, 14, 16, 18],
},
columns=["bus", "bus_sale_q1", "bus_sale_q2"],
)
data_bus
grouped_data = data_bus.groupby(by="bus", as_index=False).agg("sum")
print(grouped_data)
輸出:
bus bus_sale_q1 bus_sale_q2
0 2x 21 12
1 3Tr 23 14
2 4x 25 16
3 5x 27 18
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe