检查 Pandas 中是否存在列
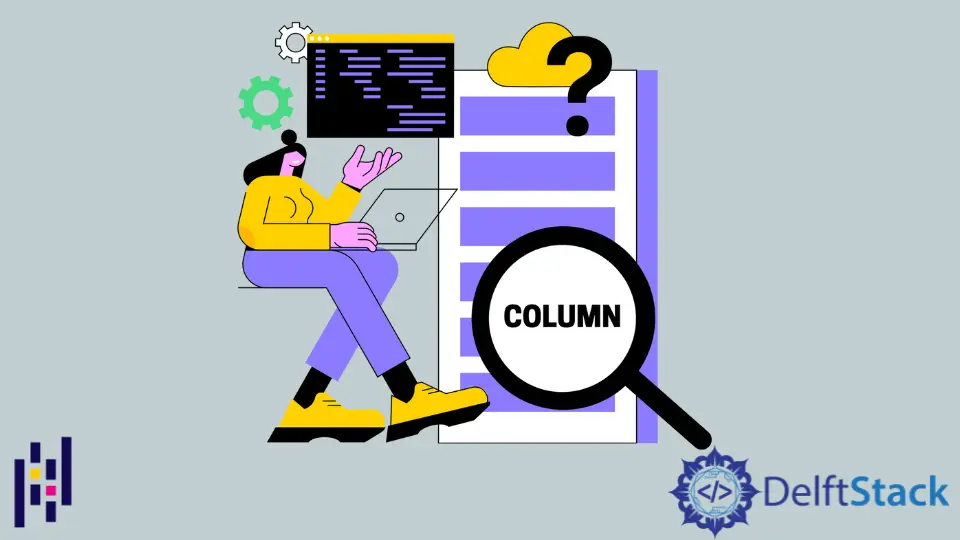
本教程演示了检查 Python 中是否存在 Pandas Dataframe 中的列的方法。我们将在 Python 中使用可用于执行此操作的 IN
和 NOT IN
运算符。
使用 IN
运算符检查 Pandas 中是否存在列
Dataframe 是一种保存二维数据及其相应标签的排列。我们可以使用 dataframe.column
属性找到列标签。
为了确保列是否存在,我们使用 IN
表达式。但是,在开始之前,我们需要在 Pandas 中形成一个虚拟 DataFrame 以使用上述技术。
在这里,我们创建了一个学生表现的 DataFrame,列名称为 Name
、Promoted
和 Marks
。
import pandas as pd
import numpy as np
# Creating dataframe
df = pd.DataFrame()
# Adding columns to the dataframe
df["Name"] = ["John", "Doe", "Bill"]
df["Promoted"] = [True, False, True]
df["Marks"] = [82, 38, 63]
# Getting the dataframe as an output
print(df)
代码给出以下输出。
Name Promoted Marks
0 John True 82
1 Doe False 38
2 Bill True 63
DataFrame 准备好后,我们可以通过编写下面给出的代码来检查 DataFrame 是否包含项目或为空。为此,我们可以使用两种方法。
我们可以使用 Pandas 中存在的 df.empty
函数,或者我们可以使用 len(df.index)
检查 DataFrame 的长度。
我们在下面的示例中使用了 Pandas 属性 df.empty
。
if df.empty:
print("DataFrame is empty!")
else:
print("Not empty!")
由于我们已将数据插入列中,因此输出必须为 Not empty!
。
Not empty!
现在,让我们继续使用 IN
方法检查 Pandas DataFrame 中的列是否存在。请参阅下面的代码以查看此功能的运行情况。
if "Promoted" in df:
print("Yes, it does exist.")
else:
print("No, it does not exist.")
代码给出以下输出。
Yes, it does exist.
为了更清楚起见,也可以将其写成 if 'Promoted' in df.columns:
,而不是仅仅写成 df
。
使用 NOT IN
运算符检查 Pandas 中是否存在列
让我们看看如何使用 NOT IN
属性来执行相同的操作。它以相反的方式运行,并且由于属性中添加了否定,输出被反转。
这是下面给出的 NOT IN
属性的示例工作。
if "Promoted" not in df.columns:
print("Yes, it does not exist.")
else:
print("No, it does exist.")
代码给出以下输出。
No, it does exist.
我们已经看到如何对 DataFrame 中的单个列执行此操作。Pandas 还使用户能够检查 DataFrame 中的多个列。
这有助于快速完成任务并有助于同时对多个列进行分类。
下面是检查 Pandas DataFrame 中多列的代码片段。
if set(["Name", "Promoted"]).issubset(df.columns):
print("Yes, all of them exist.")
else:
print("No")
代码给出以下输出。
Yes, all of them exist.
set([])
也可以使用花括号来构造。
if not {"Name", "Promoted"}.issubset(df.columns):
print("Yes")
else:
print("No")
输出将是:
No
这些是检查数据中的一列或多列的可能方法。同样,我们也可以对现成的数据而不是虚拟数据执行这些功能。
我们只需要通过 read_csv
方法使用 Python Pandas 模块导入 CSV 文件。如果使用 Google Colab,请从 google.colab
导入 files
模块,以便在运行时从个人系统上传数据文件。