如何在 Java 中初始化一個陣列
Rupam Yadav
2023年10月12日
-
dataType arrayName[];
來宣告一個新的陣列 -
arrayName = new dataType[size];
來分配陣列的大小 -
arrayName[index] = value/element
用值/元素初始化陣列 -
dataType[] arrayName = new dataType[]{elements}
初始化陣列
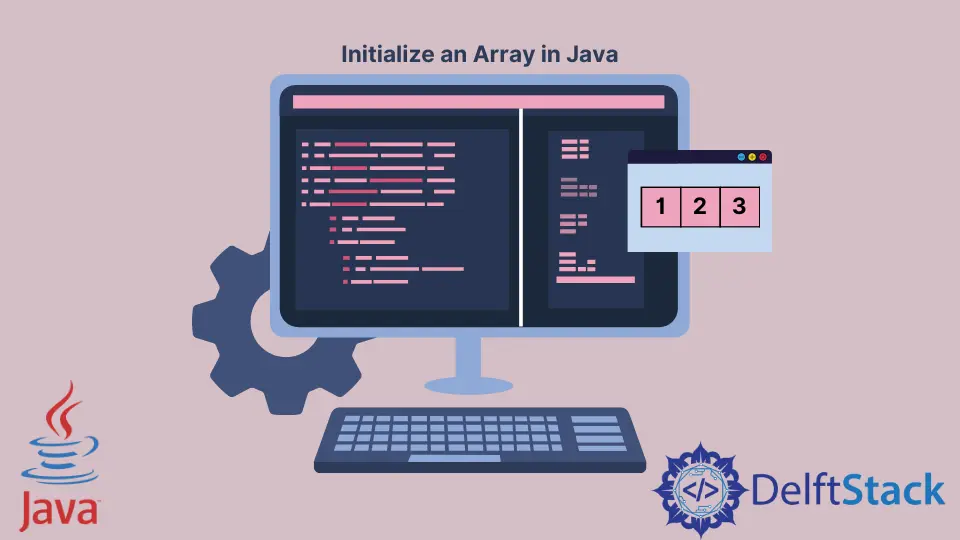
本文通過各種例項介紹了 Java 中如何宣告和初始化一個陣列。在 Java 中初始化一個陣列有兩種方法。
dataType arrayName[];
來宣告一個新的陣列
最常見的語法是 dataType arrayName[];
。
下面是一個宣告一個陣列的例子,這個陣列將在其中存放 Integer
值。
public class Main {
public static void main(String[] args) {
int[] arrayInt;
}
}
arrayName = new dataType[size];
來分配陣列的大小
Java 中的陣列可以容納固定數量的元素,這些元素的型別是相同的。這意味著在初始化時必須指定陣列的大小。當陣列被初始化時,它被儲存在一個共享記憶體中,其中的記憶體位置是根據陣列的大小給予該陣列的。
一個簡單的例子可以更好地解釋這個問題。
public class Main {
public static void main(String[] args) {
int[] arrayInt = new int[10];
System.out.println("The size of the array is: " + arrayInt.length);
}
}
在上面的例子中,
arrayInt
是一個被分配為 10 的陣列。
必須使用 new
關鍵字來例項化一個陣列。
輸出顯示了陣列的總大小,但其中沒有任何值。
輸出:
The size of the array is: 10
arrayName[index] = value/element
用值/元素初始化陣列
第一個初始化陣列的方法是通過索引號來初始化要儲存值的地方。
我們看一下這個例子就能理解清楚。
public class Main {
public static void main(String[] args) {
int[] arrayInt = new int[5];
arrayInt[0] = 10;
arrayInt[1] = 20;
arrayInt[2] = 30;
arrayInt[3] = 40;
arrayInt[4] = 50;
for (int i = 0; i < arrayInt.length; i++) {
System.out.println(arrayInt[i] + " is stored at index " + i);
}
}
}
輸出:
10 is stored at index 0
20 is stored at index 1
30 is stored at index 2
40 is stored at index 3
50 is stored at index 4
dataType[] arrayName = new dataType[]{elements}
初始化陣列
我們有另一種方法來初始化一個陣列,同時在宣告陣列時直接儲存陣列元素。當陣列的大小已經知道的時候,這種方法很有用,同時也讓程式碼更加清晰易讀。
下面是一個包含字串值的陣列的例子。
public class Main {
public static void main(String[] args) {
String[] arrayString = new String[] {"one", "two", "three", "four", "five"};
for (int i = 0; i < arrayInt.length; i++) {
System.out.println(arrayInt[i] + " is stored at index " + i);
}
}
}
輸出:
one is stored at index 0
two is stored at index 1
three is stored at index 2
four is stored at index 3
five is stored at index 4
作者: Rupam Yadav
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn