Java で配列を初期化する方法
Rupam Yadav
2023年10月12日
Java
Java Array
-
新しい配列を宣言するには
dataType arrayName[];
を使用する -
配列のサイズを割り当てるための
arrayName = new dataType[size];
-
値/要素で配列を初期化するための
arrayName[index] = value/element
-
サイズなしで配列を初期化する
dataType[] arrayName = new dataType[]{elements}
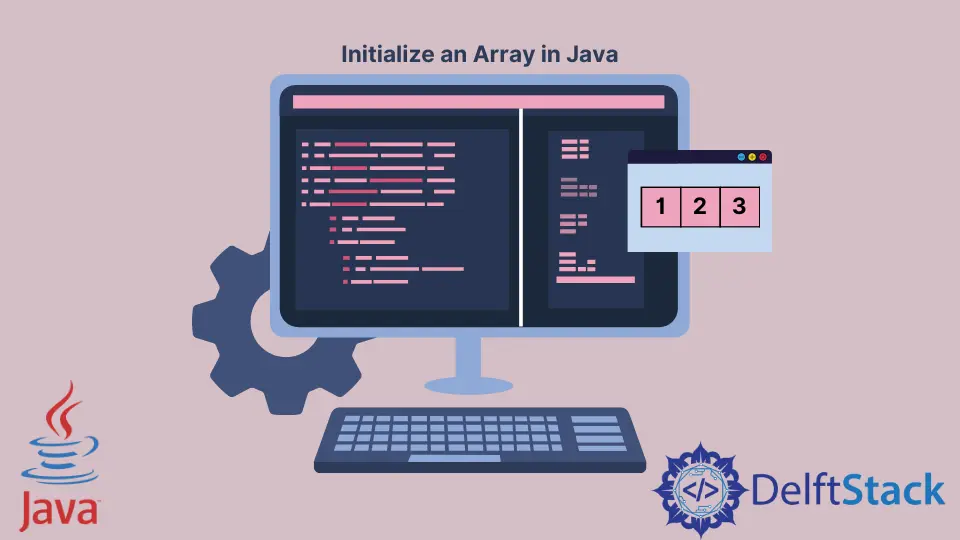
この記事では、配列を宣言して初期化する方法を様々な例を交えて紹介します。Java で配列を初期化する方法は 2つあります。
新しい配列を宣言するには dataType arrayName[];
を使用する
最も一般的な構文は dataType arrayName[];
です。
以下は、整数値を保持する配列を宣言した例です。
public class Main {
public static void main(String[] args) {
int[] arrayInt;
}
}
配列のサイズを割り当てるための arrayName = new dataType[size];
Java の配列は、同じ型の要素を一定数保持します。つまり、初期化時に配列のサイズを指定する必要があります。配列が初期化されると、その配列は共有メモリに格納され、そのサイズに応じたメモリの位置がその配列に与えられます。
簡単な例でこれをよりよく説明できます。
public class Main {
public static void main(String[] args) {
int[] arrayInt = new int[10];
System.out.println("The size of the array is: " + arrayInt.length);
}
}
上の例では
arrayInt
は 10 のサイズが割り当てられた配列です。
配列のインスタンスを作成するには、new
キーワードを使用しなければなりません。
出力は配列の合計サイズを示しますが、内部には値がありません。
出力:
The size of the array is: 10
値/要素で配列を初期化するための arrayName[index] = value/element
配列を初期化する最初の方法は、値を格納するインデックス番号によるものです。
分かりやすいように例を見てみましょう。
public class Main {
public static void main(String[] args) {
int[] arrayInt = new int[5];
arrayInt[0] = 10;
arrayInt[1] = 20;
arrayInt[2] = 30;
arrayInt[3] = 40;
arrayInt[4] = 50;
for (int i = 0; i < arrayInt.length; i++) {
System.out.println(arrayInt[i] + " is stored at index " + i);
}
}
}
出力:
10 is stored at index 0
20 is stored at index 1
30 is stored at index 2
40 is stored at index 3
50 is stored at index 4
サイズなしで配列を初期化する dataType[] arrayName = new dataType[]{elements}
配列を初期化する別の方法がありますが、その一方で配列の要素は配列の宣言時に直接格納されます。これは、配列のサイズがすでにわかっている場合や、コードを読みやすくするために便利です。
以下は、文字列の値を含む配列の例です。
public class Main {
public static void main(String[] args) {
String[] arrayString = new String[] {"one", "two", "three", "four", "five"};
for (int i = 0; i < arrayInt.length; i++) {
System.out.println(arrayInt[i] + " is stored at index " + i);
}
}
}
出力:
one is stored at index 0
two is stored at index 1
three is stored at index 2
four is stored at index 3
five is stored at index 4
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: Rupam Yadav
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn