在 C# 中讀取檔案到字串
Muhammad Maisam Abbas
2024年2月16日
Csharp
Csharp File
Csharp String
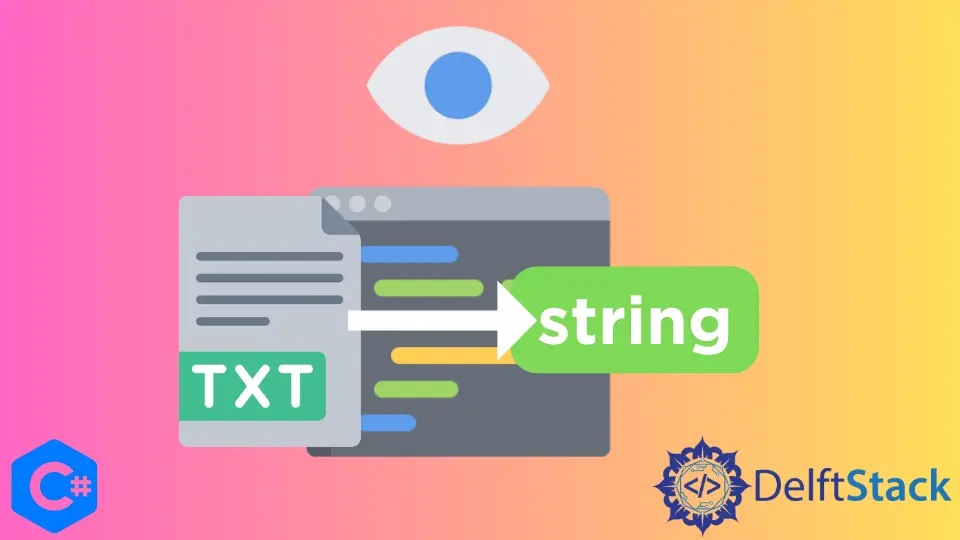
本教程將討論在 C# 中將檔案的所有內容讀取為字串變數的方法。
在 C# 中使用 File.ReadAllText()
方法將檔案讀取為字串
File
類提供了許多與 C# 中的檔案進行互動的功能。C# 中的 File.ReadAllText()
方法(File.ReadAllText 方法(System.IO)| Microsoft Docs)讀取檔案的所有內容。File.ReadAllText()
方法將檔案的路徑作為引數,並以字串變數返回指定檔案的內容。請參見以下示例程式碼。
using System;
using System.IO;
namespace read_file_to_string {
class Program {
static void Main(string[] args) {
string text = File.ReadAllText(@"C:\File\file.txt");
Console.WriteLine(text);
}
}
}
輸出:
this is all the text in this file
在上面的程式碼中,我們使用 C# 中的 File.ReadAllText()
方法將路徑 C:\File\
內檔案 file.txt
的所有內容讀取到字串變數 text
中。
在 C# 中使用 StreamReader.ReadToEnd()
方法將檔案讀取為字串
StreamReader
類從位元組流中讀取具有 C# 特定編碼的內容。StreamReader.ReadToEnd()
方法用於讀取 C# 中檔案的所有內容。StreamReader.ReadToEnd()
方法以字串變數返回指定檔案的內容。請參見以下示例程式碼。
using System;
using System.IO;
namespace read_file_to_string {
class Program {
static void Main(string[] args) {
StreamReader fileReader = new StreamReader(@"C:\File\file.txt");
string text = fileReader.ReadToEnd();
Console.WriteLine(text);
}
}
}
輸出:
this is all the text in this file
在上面的程式碼中,我們使用 C# 中的 StreamReader.ReadToEnd()
方法將路徑 C:\File\
內檔案 file.txt
的所有內容讀取到字串變數 text
中。這種方法比以前的方法快得多。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn