C# 讀取和解析 XML 檔案
Minahil Noor
2023年12月11日
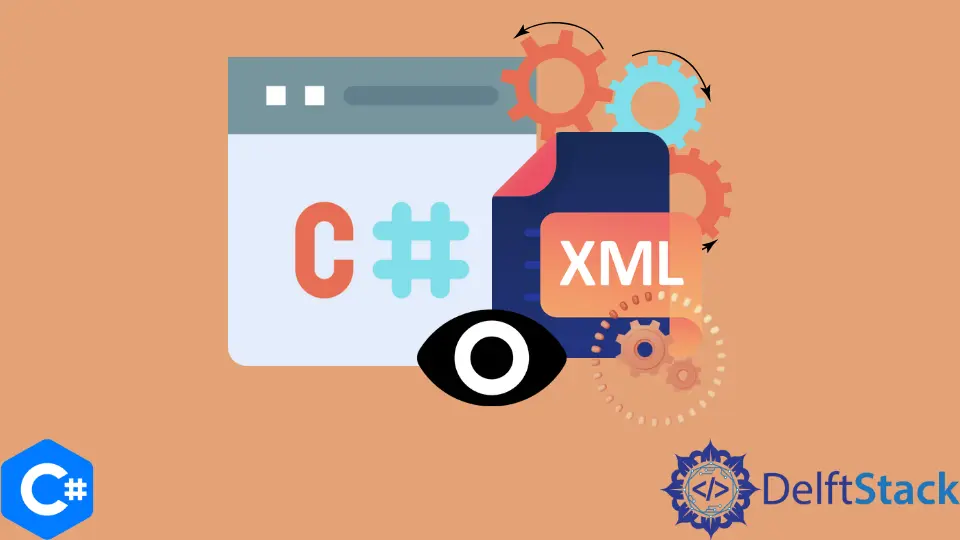
在 C# 中,System.Xml
名稱空間用於處理 XML
檔案。它具有不同的類和方法來處理 XML 檔案。我們可以使用該名稱空間讀取,解析和寫入 XML 檔案。
在本文中,我們將討論用於讀取和解析 XML 檔案的不同方法。
使用 XmlReader 類讀取和解析 XML 檔案的 C# 程式
C# 中的 XmlReader
類提供了一種訪問 XML 資料的有效方法。XmlReader.Read()
方法讀取 XML 檔案的第一個節點,然後使用 while
迴圈讀取整個檔案。
此方法的正確語法如下:
XmlReader VariableName = XmlReader.Create(@"filepath");
while (reader.Read()) {
// Action to perform repeatidly
}
我們已經在程式中讀取並解析的 XML 檔案如下。複製此程式碼並將其貼上到新的文字檔案中,並將其另存為 .xml
,以使用該檔案執行以下給出的程式。
<?xml version="1.0" encoding="utf-8"?>
<Students>
<Student>
</Student>
<Student>
<Name>Olivia</Name>
<Grade>A</Grade>
</Student>
<Student>
<Name>Laura</Name>
<Grade>A+</Grade>
</Student>
<Student>
<Name>Ben</Name>
<Grade>A-</Grade>
</Student>
<Student>
<Name>Henry</Name>
<Grade>B</Grade>
</Student>
<Student>
<Name>Monica</Name>
<Grade>B</Grade>
</Student>
</Students>
示例程式碼:
using System;
using System.Xml;
namespace XMLReadAndParse {
class XMLReadandParse {
static void Main(string[] args) {
// Start with XmlReader object
// here, we try to setup Stream between the XML file nad xmlReader
using (XmlReader reader = XmlReader.Create(@"d:\Example.xml")) {
while (reader.Read()) {
if (reader.IsStartElement()) {
// return only when you have START tag
switch (reader.Name.ToString()) {
case "Name":
Console.WriteLine("The Name of the Student is " + reader.ReadString());
break;
case "Grade":
Console.WriteLine("The Grade of the Student is " + reader.ReadString());
break;
}
}
Console.WriteLine("");
}
}
Console.ReadKey();
}
}
}
在這裡,我們建立了一個 XmlReader
物件,然後使用 Create()
方法建立了給定 XML 檔案的閱讀器流。
然後,我們使用了 XmlReader.Read()
方法來讀取 XML 檔案。該方法返回一個 bool
值,該值指示我們建立的流是否包含 XML
語句。
之後,我們使用 XmlReader.IsStartElement()
方法檢查是否有任何起始元素。因為我們在 XML 資料中有兩個元素欄位,所以我們使用了 switch
語句通過 ReadString()
方法從兩個欄位中讀取資料。
輸出:
The Name of the Student is Olivia
The Grade of the Student is A
The Name of the Student is Laura
The Grade of the Student is A+
The Name of the Student is Ben
The Grade of the Student is A-
The Name of the Student is Henry
The Grade of the Student is B
The Name of the Student is Monica
The Grade of the Student is B