C# Legge e analizza un file XML
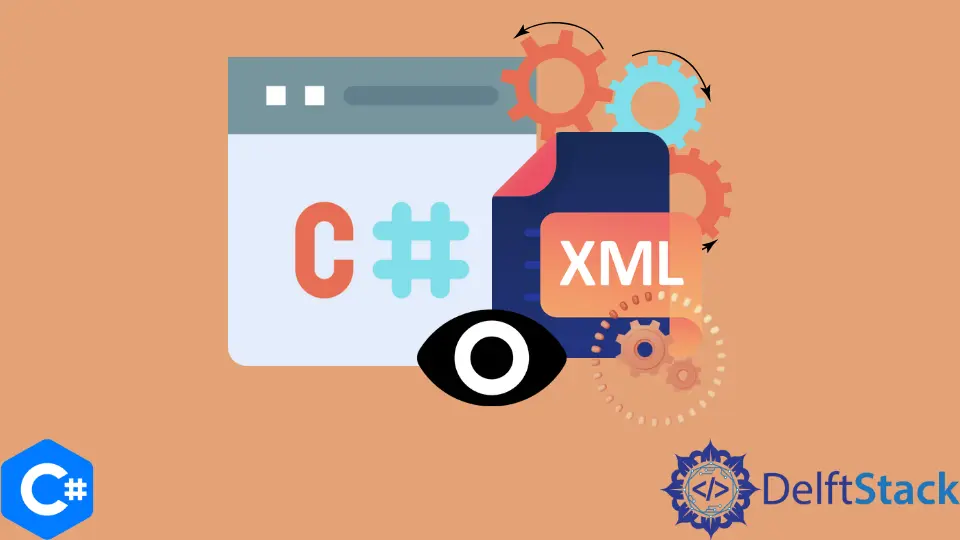
In C#, lo spazio dei nomi System.Xml
viene utilizzato per gestire i file XML
. Ha diverse classi e metodi per elaborare i file XML
. Possiamo leggere, analizzare e scrivere un file XML
utilizzando questo spazio dei nomi.
In questo articolo, discuteremo i diversi metodi usati per leggere e analizzare un file XML
.
Programma C# per leggere e analizzare un file XML
utilizzando la classe XmlReader
La classe XmlReader
in C# fornisce un modo efficiente per accedere ai dati XML
. Il metodo XmlReader.Read()
legge il primo nodo del file XML
e poi legge l’intero file usando un cicli while
.
La sintassi corretta per utilizzare questo metodo è la seguente:
XmlReader VariableName = XmlReader.Create(@"filepath");
while (reader.Read()) {
// Action to perform repeatidly
}
Il file XML
che abbiamo letto e analizzato nel nostro programma è il seguente. Copia e incolla questo codice in un nuovo file di testo e salvalo come .xml
per utilizzare questo file per eseguire il programma indicato di seguito.
<?xml version="1.0" encoding="utf-8"?>
<Students>
<Student>
</Student>
<Student>
<Name>Olivia</Name>
<Grade>A</Grade>
</Student>
<Student>
<Name>Laura</Name>
<Grade>A+</Grade>
</Student>
<Student>
<Name>Ben</Name>
<Grade>A-</Grade>
</Student>
<Student>
<Name>Henry</Name>
<Grade>B</Grade>
</Student>
<Student>
<Name>Monica</Name>
<Grade>B</Grade>
</Student>
</Students>
Codice di esempio:
using System;
using System.Xml;
namespace XMLReadAndParse {
class XMLReadandParse {
static void Main(string[] args) {
// Start with XmlReader object
// here, we try to setup Stream between the XML file nad xmlReader
using (XmlReader reader = XmlReader.Create(@"d:\Example.xml")) {
while (reader.Read()) {
if (reader.IsStartElement()) {
// return only when you have START tag
switch (reader.Name.ToString()) {
case "Name":
Console.WriteLine("The Name of the Student is " + reader.ReadString());
break;
case "Grade":
Console.WriteLine("The Grade of the Student is " + reader.ReadString());
break;
}
}
Console.WriteLine("");
}
}
Console.ReadKey();
}
}
}
Qui abbiamo creato un oggetto di XmlReader
e quindi abbiamo creato un flusso di lettura del file XML
dato usando il metodo Create()
.
Quindi, abbiamo utilizzato il metodo XmlReader.Read()
per leggere il file XML
. Questo metodo restituisce un valore bool
che indica se il nostro flusso creato contiene le istruzioni XML
o meno.
Dopodiché, abbiamo verificato se esiste un elemento di partenza utilizzando il metodo XmlReader.IsStartElement()
. Poiché abbiamo due campi elemento nei nostri dati XML
, abbiamo utilizzato l’istruzione switch
per leggere i dati da entrambi i campi utilizzando il metodo ReadString()
.
Produzione:
The Name of the Student is Olivia
The Grade of the Student is A
The Name of the Student is Laura
The Grade of the Student is A +
The Name of the Student is Ben
The Grade of the Student is A -
The Name of the Student is Henry
The Grade of the Student is B
The Name of the Student is Monica
The Grade of the Student is B