C# Leer y analizar un archivo XML
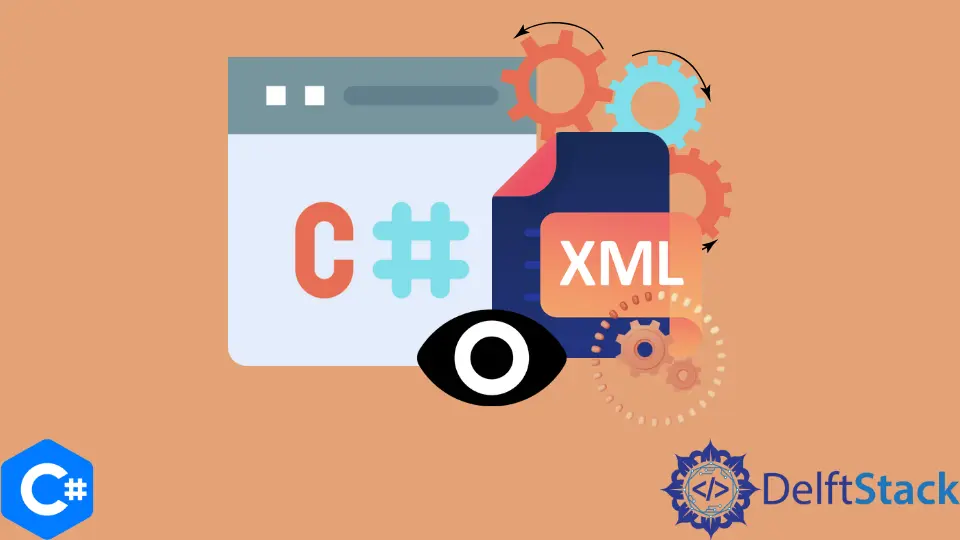
En C#, el espacio de nombres System.Xml
se usa para tratar los archivos XML
. Tiene diferentes clases y métodos para procesar los archivos XML
. Podemos leer, analizar y escribir un archivo XML
usando este espacio de nombres.
En este artículo, vamos a discutir los diferentes métodos que se usan para leer y analizar un archivo XML
.
Programa C# para leer y analizar un archivo XML
usando la clase XmlReader
La clase XmlReader en C# proporciona una manera eficiente de acceder a los datos XML
. El método XmlReader.Read()
lee el primer nodo del archivo XML
y luego lee todo el archivo usando un bucle while
.
La sintaxis correcta para usar este método es la siguiente:
XmlReader VariableName = XmlReader.Create(@"filepath");
while (reader.Read()) {
// Action to perform repeatidly
}
El archivo XML
que hemos leído y analizado en nuestro programa es el siguiente. Copia y pega este código en un nuevo archivo de texto y guárdalo como .xml
para usar este archivo para ejecutar el programa que se indica a continuación.
<?xml version="1.0" encoding="utf-8"?>
<Students>
<Student>
</Student>
<Student>
<Name>Olivia</Name>
<Grade>A</Grade>
</Student>
<Student>
<Name>Laura</Name>
<Grade>A+</Grade>
</Student>
<Student>
<Name>Ben</Name>
<Grade>A-</Grade>
</Student>
<Student>
<Name>Henry</Name>
<Grade>B</Grade>
</Student>
<Student>
<Name>Monica</Name>
<Grade>B</Grade>
</Student>
</Students>
Código de ejemplo:
using System;
using System.Xml;
namespace XMLReadAndParse {
class XMLReadandParse {
static void Main(string[] args) {
// Start with XmlReader object
// here, we try to setup Stream between the XML file nad xmlReader
using (XmlReader reader = XmlReader.Create(@"d:\Example.xml")) {
while (reader.Read()) {
if (reader.IsStartElement()) {
// return only when you have START tag
switch (reader.Name.ToString()) {
case "Name":
Console.WriteLine("The Name of the Student is " + reader.ReadString());
break;
case "Grade":
Console.WriteLine("The Grade of the Student is " + reader.ReadString());
break;
}
}
Console.WriteLine("");
}
}
Console.ReadKey();
}
}
}
Aquí hemos creado un objeto de XmlReader
y luego hemos creado un flujo lector del archivo XML
dado usando el método Create()
.
Luego, hemos usado el método XmlReader.Read()
para leer el archivo XML
. Este método devuelve un valor bool
que indica si nuestro flujo creado contiene las declaraciones XML
o no.
Después de eso, hemos comprobado si hay algún elemento de inicio usando el método XmlReader.IsStartElement()
. Como tenemos dos campos de elementos en nuestros datos XML
, hemos usado la sentencia switch
para leer los datos de ambos campos usando el método ReadString()
.
Producción :
The Name of the Student is Olivia
The Grade of the Student is A
The Name of the Student is Laura
The Grade of the Student is A+
The Name of the Student is Ben
The Grade of the Student is A-
The Name of the Student is Henry
The Grade of the Student is B
The Name of the Student is Monica
The Grade of the Student is B