C# XML 파일 읽기 및 구문 분석
Minahil Noor
2024년2월16일
Csharp
Csharp Xml
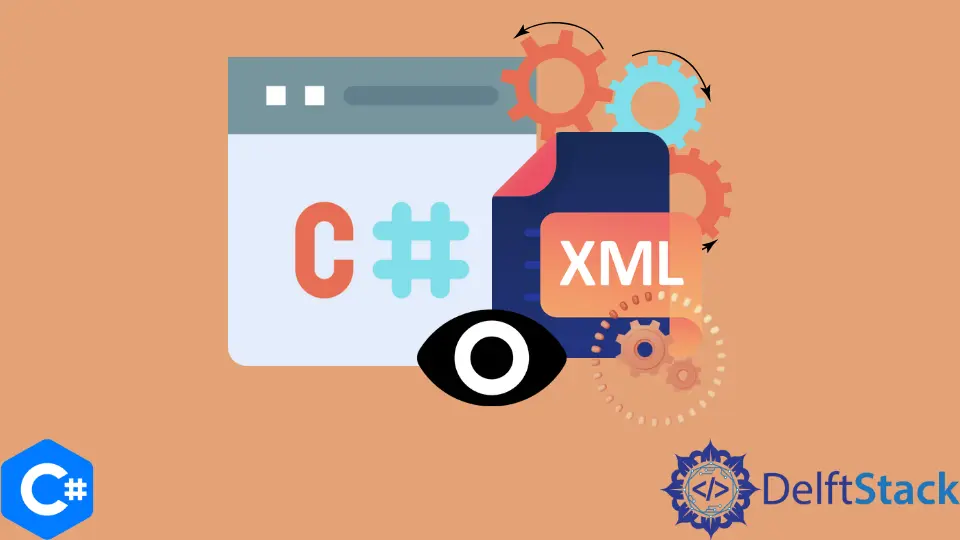
C#에서System.Xml
네임 스페이스는XML
파일을 처리하는 데 사용됩니다. XML
파일을 처리하는 클래스와 메소드가 다릅니다. 이 네임 스페이스를 사용하여 XML 파일을 읽고 구문 분석하고 작성할 수 있습니다.
이 기사에서는 XML 파일을 읽고 파싱하는 데 사용되는 여러 가지 방법에 대해 설명합니다.
XmlReader 클래스를 사용하여 XML 파일을 읽고 구문 분석하는 C# 프로그램
C#의 XmlReader
클래스는XML
데이터에 액세스하는 효율적인 방법을 제공합니다. XmlReader.Read()
메소드는 XML
파일의 첫 번째 노드를 읽은 다음while
루프를 사용하여 전체 파일을 읽습니다.
이 방법을 사용하는 올바른 구문은 다음과 같습니다.
XmlReader VariableName = XmlReader.Create(@"filepath");
while (reader.Read()) {
// Action to perform repeatidly
}
프로그램에서 읽고 파싱 한 XML 파일은 다음과 같습니다. 이 코드를 복사하여 새 텍스트 파일에 붙여넣고.xml
로 저장하여이 파일을 사용하여 아래 제공된 프로그램을 실행하십시오.
<?xml version="1.0" encoding="utf-8"?>
<Students>
<Student>
</Student>
<Student>
<Name>Olivia</Name>
<Grade>A</Grade>
</Student>
<Student>
<Name>Laura</Name>
<Grade>A+</Grade>
</Student>
<Student>
<Name>Ben</Name>
<Grade>A-</Grade>
</Student>
<Student>
<Name>Henry</Name>
<Grade>B</Grade>
</Student>
<Student>
<Name>Monica</Name>
<Grade>B</Grade>
</Student>
</Students>
예제 코드:
using System;
using System.Xml;
namespace XMLReadAndParse {
class XMLReadandParse {
static void Main(string[] args) {
// Start with XmlReader object
// here, we try to setup Stream between the XML file nad xmlReader
using (XmlReader reader = XmlReader.Create(@"d:\Example.xml")) {
while (reader.Read()) {
if (reader.IsStartElement()) {
// return only when you have START tag
switch (reader.Name.ToString()) {
case "Name":
Console.WriteLine("The Name of the Student is " + reader.ReadString());
break;
case "Grade":
Console.WriteLine("The Grade of the Student is " + reader.ReadString());
break;
}
}
Console.WriteLine("");
}
}
Console.ReadKey();
}
}
}
여기서 우리는XmlReader
의 객체를 만들고Create()
메소드를 사용하여 주어진XML
파일의 리더 스트림을 만들었습니다.
그런 다음 XmlReader.Read()
메서드를 사용하여 XML 파일을 읽었습니다. 이 메소드는 생성 된 스트림에 XML
문이 포함되어 있는지 여부를 나타내는 bool 값을 반환합니다.
그런 다음 XmlReader.IsStartElement()
메서드를 사용하여 시작 요소가 있는지 확인했습니다. XML 데이터에 두 개의 요소 필드가 있기 때문에switch
문을 사용하여ReadString()
메소드를 사용하여 두 필드에서 데이터를 읽습니다.
출력:
The Name of the Student is Olivia
The Grade of the Student is A
The Name of the Student is Laura
The Grade of the Student is A+
The Name of the Student is Ben
The Grade of the Student is A-
The Name of the Student is Henry
The Grade of the Student is B
The Name of the Student is Monica
The Grade of the Student is B
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다