Tkinter Tutorial - Combobox
- Python Tkinter TTK Combobox Example
- Python Tkinter TTK Combobox Fonts
- Python Tkinter TTK Combobox Event Binding
- Python Tkinter TTK Combobox Dynamically Update Values
- Python Tkinter TTK Combobox Read Only
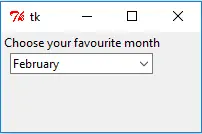
Tkinter Combobox is the drop-down list for the user to choose from. It is a combination of Entry
and drop-down
widgets as you can see. When you click the arrow on the left side, you will see a drop-down menu showing all the choices, and if you click on one, it will replace the current Entry
contents.
Python Tkinter TTK Combobox Example
import tkinter as tk
from tkinter import ttk
app = tk.Tk()
app.geometry("200x100")
labelTop = tk.Label(app, text="Choose your favourite month")
labelTop.grid(column=0, row=0)
comboExample = ttk.Combobox(app, values=["January", "February", "March", "April"])
pprint(dict(comboExample))
comboExample.grid(column=0, row=1)
comboExample.current(1)
print(comboExample.current(), comboExample.get())
app.mainloop()
from tkinter import ttk
Tkinter Combobox
widget is in the ttk
module of Tkinter, therefore, you need to import the ttk
module to use this widget. You don’t need to import the ttk
module to use the Tkinter widgets like Button
.
comboExample = ttk.Combobox(app, values=["January", "February", "March", "April"])
It creates the Tkinter combobox widget instance and also assigns the values that will appear in the drop-down list.
comboExample.current(1)
Normally, the default item showing in the combobox is the first element in the values list. You could also change it to any element by using current(index)
method.
print(comboExample.current(), comboExample.get())
You could also use current()
to get the index of the currently selected element, and use get()
method to get the element itself.
Python Tkinter TTK Combobox Fonts
It needs to set fonts for both Combobox and also its list box to achieve the goal that fonts of Combobox is correctly configured.
import tkinter as tk
from tkinter import ttk
app = tk.Tk()
app.geometry("300x100")
labelTop = tk.Label(app, text="Choose your favourite month")
labelTop.grid(column=0, row=0)
fontExample = ("Courier", 16, "bold")
comboExample = ttk.Combobox(
app, values=["January", "February", "March", "April"], font=fontExample
)
app.option_add("*TCombobox*Listbox.font", fontExample)
comboExample.grid(column=0, row=1)
app.mainloop()
fontExample = ("Courier", 16, "bold")
comboExample = ttk.Combobox(
app, values=["January", "February", "March", "April"], font=fontExample
)
Tkinter font could be specified with a tuple with options of font-family
, size, weight (bold
or normal
), slant (italic
or roman
), etc. The font tuple is assigned to the Combobox by font = fontExample
.
app.option_add("*TCombobox*Listbox.font", fontExample)
We also need to specify the font of the list box of Combobox otherwise the text in the list of Combobox still uses the system default font but not the fault specified to the Combobox.
Python Tkinter TTK Combobox Event Binding
The combobox callback function binding is different from the widgets introduced in the last chapters. bind()
method is the way to bind the callback function with the combobox virtual event when the user selects an element in the drop-down list.
import tkinter as tk
from tkinter import ttk
def callbackFunc(event):
print("New Element Selected")
app = tk.Tk()
app.geometry("200x100")
labelTop = tk.Label(app, text="Choose your favourite month")
labelTop.grid(column=0, row=0)
comboExample = ttk.Combobox(app, values=["January", "February", "March", "April"])
comboExample.grid(column=0, row=1)
comboExample.current(1)
comboExample.bind("<<ComboboxSelected>>", callbackFunc)
app.mainloop()
def callbackFunc(event):
print("New Element Selected")
This is the defined callback function whenever you select an element from the list.
event
shall not be skipped as the passing argument, it is passed from the combobox virtual eventcomboExample.bind("<<ComboboxSelected>>", callbackFunc)
It binds the virtual event <<ComboboxSelected>>
with the callback function.
Every time you select a new element from the list, it prints New Element Selected
.
Python Tkinter TTK Combobox Dynamically Update Values
The list of choices in Tkinter combobox could be dynamically updated with the option postcommand
that executes the given function before displaying the pop-down list of choices.
import tkinter as tk
from tkinter import ttk
def callbackFunc(event):
print("New Element Selected")
app = tk.Tk()
app.geometry("200x100")
def changeMonth():
comboExample["values"] = ["July", "August", "September", "October"]
labelTop = tk.Label(app, text="Choose your favourite month")
labelTop.grid(column=0, row=0)
comboExample = ttk.Combobox(
app, values=["January", "February", "March", "April"], postcommand=changeMonth
)
comboExample.grid(column=0, row=1)
app.mainloop()
The changeMonth
function changes the combobox list of choices to be
["July", "August", "September", "October"]
The combobox shows empty after GUI starts and shows the given months as above after the user clicks the arrow in the combobox.
Python Tkinter TTK Combobox Read Only
The content of the elements in the Combobox examples shown above is editable because the default state
is normal
that makes the text field directly editable.
Tkinter Combobox has the other two states besides normal
,
readonly
- the text field is not editable, and the user could only select the values from the drop-down list.disabled
- the Combobox is grayed out and interaction is impossible.
import tkinter as tk
from tkinter import ttk
app = tk.Tk()
app.geometry("200x100")
labelTop = tk.Label(app, text="Choose your favourite month")
labelTop.grid(column=0, row=0)
comboExample = ttk.Combobox(
app, values=["January", "February", "March", "April"], state="readonly"
)
comboExample.grid(column=0, row=1)
comboExample.current(0)
print(comboExample.current(), comboExample.get())
app.mainloop()
The above example sets the Tkinter Combobox read-only.
If you change the state from readonly
to disabled
, the Combobox is grayed out as shown below.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook