NumPy Tutorial - NumPy Array Creation
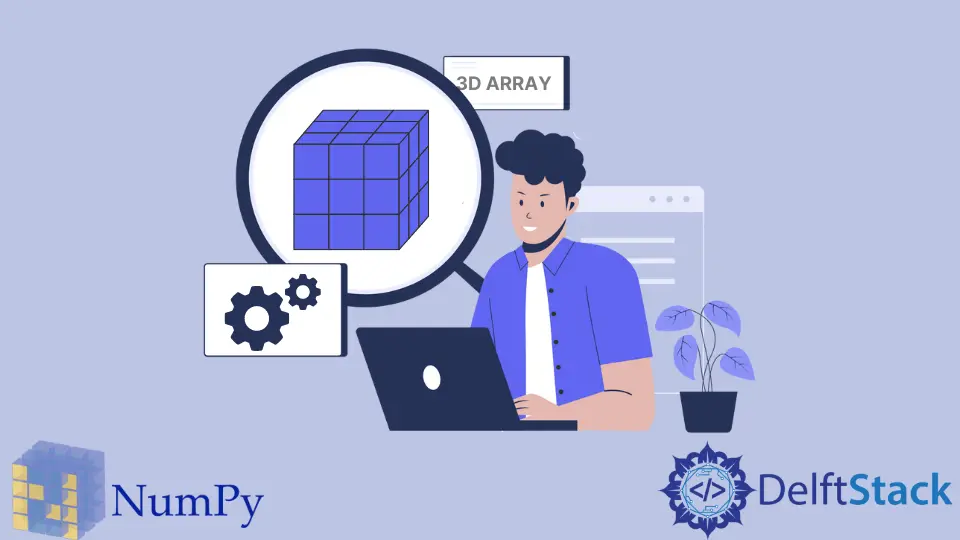
Zeros Array
np.zeros
np.zeros
is used to create the array that all the elements is 0
. Its syntax is,
np.zeros(shape, dtype=float, order="C")
Where,
shape
is the size of the matrix, and it could be 1-D, 2-D or multiple dimensions.
dtype
is float64
by default, but could be assigned with any data type in NumPy
.
Let’s create some zeros arrays
>>> import numpy as np
>>> np.zeros(5) # it creates a 1D array with 5 zeros
array([0., 0., 0., 0., 0.])
>>> np.zeros(5, dtype='int8') # it creates a 1D array with 5 zeros with the data type of int8
array([0, 0, 0, 0, 0], dtype=int8)
>>> np.zeros((4, 3)) # it creates a 4x3 zeros array
array([[0., 0., 0.],
[0., 0., 0.],
[0., 0., 0.],
[0., 0., 0.]])
np.empty
In some cases, you only want to initialize an array with the specified shape and u don’t care about the initialization data inside. You can use np.empty
to achieve faster initialization, but keep in mind that it does not guarantee the value of created array is 0.
We could use %timeit
to compare the execution time of np.empty()
and np.zeros()
,
In[1]: % timeit np.empty((100, 100))
715 ns ± 11.6 ns per loop(mean ± std. dev. of 7 runs, 1000000 loops each)
In[2]: % timeit np.zeros((100, 100))
4.03 µs ± 104 ns per loop(mean ± std. dev. of 7 runs, 100000 loops each)
Obviously, np.empty()
is much faster than np.zeros()
in initialization. It takes only about 17.5%
of time needed for np.zeros()
to initialize an array with the shape of (100, 100)
.
np.zeros_like
Suppose we already have an array and want to create a zeros array that has the same shape. We could use the traditional way to create this new array.
test = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
np.zeros(test.shape)
Or we could use the dedicated method - np.zeros_like()
to create the new array more efficiently.
x = np.zeros_like(test)
np.zeros_like()
has not only the same shape with the given array but also the same data type as it.Ones Array
np.ones
Similar to zeros
, we could also create the array filled with ones. The syntax and parameters of np.ones()
are identical to np.zeros()
.
>>> import numpy as np
>>> np.ones(5) # it creates a 1-D array with 5 ones
array([1., 1., 1., 1., 1.])
>>> np.ones(5, dtype='int8') # it creates a 1-D array with 5 ones, and its data type is int8
array([1, 1, 1, 1, 1], dtype=int8)
>>> np.ones((4, 3)) # it creates an array of (4, 3) shape
array([[1., 1., 1.],
[1., 1., 1.],
[1., 1., 1.],
[1., 1., 1.]])
np.ones_like
Similar to np.zeros_like
, np.ones_like
could be used to create a new array of ones with the same shape as given array.
>>> test = np.array([[1.0,2.0,3.0],[4.0,5.0,6.0],[7.0,8.0,9.0]])
>>> np.ones_like(test)
array([[1., 1., 1.],
[1., 1., 1.],
[1., 1., 1.]])
Diagonal Array
Diagonal array is the 2-D array with ones on the diagonal and zeros elsewhere. It could be created with np.eye()
method whose syntax is,
np.eye(N, M=None, k=0, dtype= < class 'float' >, order='C')
Where,
parameter | type of data | Summary |
---|---|---|
N |
INT | Specifies the number of rows in the generated matrix. |
M |
INT | (Optional) The default is None . Specifies the number of columns in the matrix to be generated. When it is None , it is equal to N . |
k |
INT | (Optional) The default is 0. 0 is the main diagonal position. If k is positive, it creates upper diagonal with the displacement of k ; if k is negative, it creates lower diagonal with the displacement of -k . |
dtype |
type of data | (Optional) The default value is float . It specifies the data type of the element of the created array. |
np.eye()
Example
>>> import numpy as np
>>> np.eye(3)
array([[1., 0., 0.],
[0., 1., 0.],
[0., 0., 1.]])
>>> np.eye(4, 3)
array([[1., 0., 0.],
[0., 1., 0.],
[0., 0., 1.],
[0., 0., 0.]])
>>> np.eye(3, 4)
array([[1., 0., 0., 0.],
[0., 1., 0., 0.],
[0., 0., 1., 0.]])
>>> np.eye(4, k=1)
array([[0., 1., 0., 0.],
[0., 0., 1., 0.],
[0., 0., 0., 1.],
[0., 0., 0., 0.]])
np.identity()
np.identity
could be treated as special np.eye
because it creates a square diagonal array with ones on the main diagonal.
numpy.identity(n, dtype = float)
parameter | type of data | Description |
---|---|---|
n |
INT | Specifies the size of the matrix to be generated. It creates an N×N square array. |
dtype |
type of data | (Optional) The default is float. It specifies the data type of the element. |
np.identity()
Examples
>>> import numpy as np
>>> np.identity(3)
array([[1., 0., 0.],
[0., 1., 0.],
[0., 0., 1.]])
Triangular Array
You could use np.tri()
to create a triangular array. Its syntax is,
numpy.tri(N, M=None, k=0, dtype= < class 'float' >)
Its input parameters are similar to np.eye()
.
np.tri()
Examples
>>> import numpy as np
>>> np.tri(3)
array([[1., 0., 0.],
[1., 1., 0.],
[1., 1., 1.]])
>>> np.tri(4, k=1)
array([[1., 1., 0., 0.],
[1., 1., 1., 0.],
[1., 1., 1., 1.],
[1., 1., 1., 1.]])
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook