Python Tutorial - Function
- What Is Python Function
- How to Define a Python Function
- Python Function Example
- Call a Function in Python
-
the
return
Statement - Scope and Lifetime of Python Variables
- Types of Functions
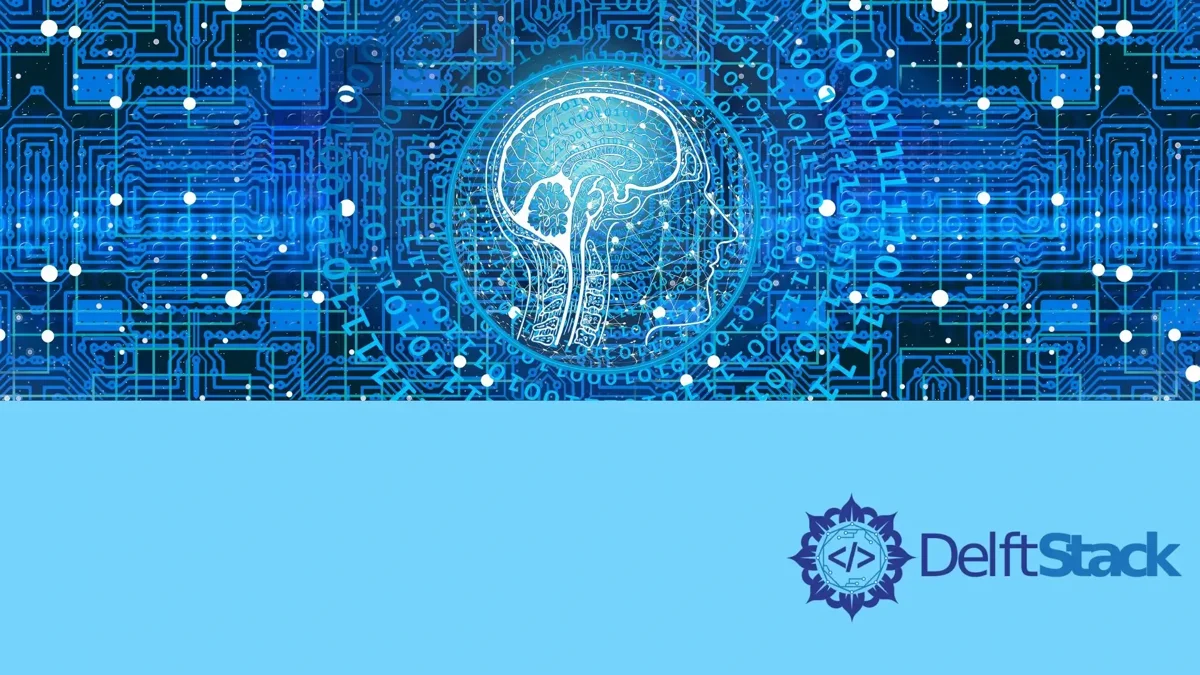
In this section, you will learn
- What is a function
- How to create a function
- Types of functions
What Is Python Function
A function is a small block of a program that contains a number of statements to perform a specific task. When you have a program of thousands of lines performing different tasks then you should divide the program into small modules (blocks) which increases the readability and lowers the complexity.
How to Define a Python Function
The following is the syntax to define a function:
def functionName(arguments):
"""This is the definition of this function"""
statements
return returnParam
- The keyword
def
is used to define a function. functionName
is the name of the function.arguments
are optional. Arguments provide values to function to perform operations on.- Colon (
:
) ends the function header. """This is the definition of this function"""
is adocstring
and is optional which describes what the function does.statements
refers to the body of the function.- The statement
return
optionally returns the result to the caller.
Python Function Example
def language(p):
"""Function to print a message"""
print("Programming language:", p)
Here a function language
is defined which has one argument p
passing from the caller. Inside the function, there is a docstring and a print
statement to print the message containing the argument p
.
Call a Function in Python
A function can be called from anywhere in the program. A function can be called by its name with the required parameters.
language("Python")
Programming language: Python
the return
Statement
The return
statement transfers the control back to the codes where the function is called.
It indicates the ending of the function definition.
The syntax of return
is as follows:
return [values_to_be_returned]
If there is no return
statement in a function, a None
object will be returned.
Example of Using return
Statement
def square(n):
return n * n
print("Square of 4=", square(4))
Square of 4=16
In this code, the function is called in a print
function and 4 is passed as an argument. Inside the function, you have an expression n*n
which is evaluated and the result is returned to where the function is called (print
statement).
Scope and Lifetime of Python Variables
The scope of a variable is where a variable can be accessed. When a variable is declared inside a function, it is not accessible to the outside of that function. This type of variables is called local variable and is accessed only to the function where it is declared.
The lifetime of a variable is the time during which a variable exists in memory. When a variable is declared inside a function, the memory of it will be released when the control jumps out of the function.
See the example below:
def fun():
a = 12
print("Value of a inside function:", a)
a = 24
fun()
print("Value of a outside function:", a)
Value of a inside function: 12
Value of a outside function: 24
In this code, variable a
inside the function and variable a
outside the function are different variables.
If you try to access variables declared inside functions from outside, you will come across an error - NameError name 'x' is not defined
. But the variables declared outside of functions have a global scope and can be accessed from inside.
Types of Functions
Functions in Python can be categorized into two types:
Built-in functions
: have a predefined meaning and perform specific tasks.User-defined functions
: are defined by the user containing any number of statements to perform user-defined tasks.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook