Python Tutorial - Function Arguments
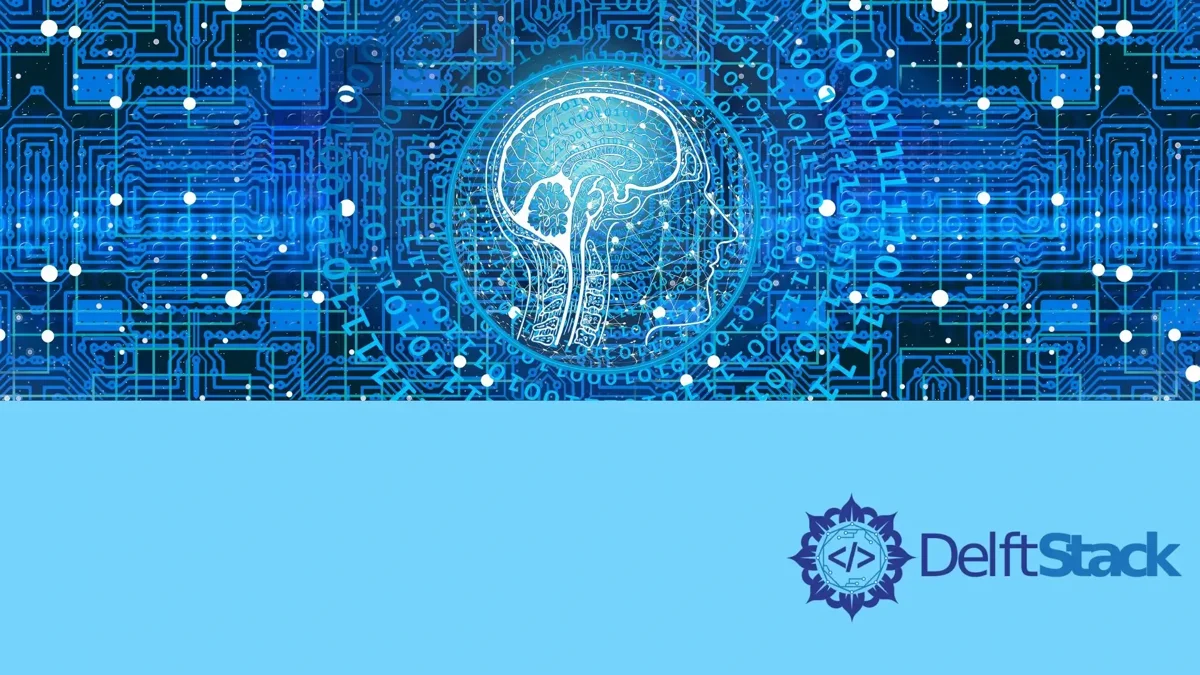
In this section, you will learn Python function arguments and its different types such as default arguments, keyword arguments, and arbitrary arguments.
Python Function Arguments
Arguments of function are the values on which the operations are performed inside the function.
When you define a function with a specified number of arguments, you have to call this particular function by passing that specified number of arguments.
If you pass more or fewer arguments than specified in the function definition, you will get a TypeError
.
Consider the code below:
def language(p, n):
"""Function to print a message"""
print("Programming language", n, "is:", p)
language("Python", 1)
language("Java", 2)
Programming language 1 is: Python
Programming language 2 is: Java
The function language
has two arguments. And if you call the function with a different number of arguments, you will have an error as below:
>>> language('Python')
Traceback (most recent call last):
File "<pyshell#7>", line 1, in <module>
language('Python')
TypeError: language() takes exactly 2 arguments (1 given)
>>> language(2, 'Python', 'Java')
Traceback (most recent call last):
File "<pyshell#8>", line 1, in <module>
language(2, 'Python', 'Java')
TypeError: language() takes exactly 2 arguments (3 given)
Python Function Arguments Types
The previous function has a fixed number of arguments. And function could also have variable arguments.
There are three different ways to pass variable arguments to a function. Now let’s go through them all.
Python Function Default Arguments
In a function, arguments can have default values which are assigned in the function definition. If the arguments have a default value, then it is optional to pass values for those arguments:
This is demonstrated in the example below:
def language(p="C++", n=3):
"""Function to print a message"""
print("Programming language", n, "is:", p)
language()
Programming language 3 is: C++
You could see here that no argument is passed but the program still works fine; because default values for the arguments are provided. If you pass values for any of the arguments, the default value will be overwritten.
Python Function Keyword Arguments
Normally, you pass multiple arguments in the same sequence as defined in the function definition. But if you use keyword arguments you can change the position of the arguments.
def language(p, n):
"""Function to print a message"""
print("Programming language", n, "is:", p)
language(p="C++", n=3)
Programming language 3 is: C++
You can also change the position of arguments if you use keyword arguments to assign values explicitly to the specific arguments.
def language(p, n):
"""Function to print a message"""
print("Programming language", n, "is:", p)
language(n=3, p="C++")
Programming language 3 is: C++
Python Function Arbitrary Arguments
Arbitrary arguments are used when it is not known in advance that how many arguments should be passed.
An Arbitrary function is denoted by an asterisk *
before the name of the argument. It passes non-keyworded arguments as a tuple to the function.
def language(*args):
"""Demo function of arbitray arguments"""
for i in args:
print("Programming language:", i)
language("Java", "C++", "R", "Kotlin")
Programming language: Java
Programming language: C++
Programming language: R
Programming language: Kotlin
The function is called by passing multiple arguments. These arguments are converted into a tuple before the function is actually called.
Double asterisk **kwargs
is the idiom to pass the arbitrary keyword arguments to the function. For example,
def language(**kwargs):
"""Demo funciton of arbitrary keyword arguments"""
for a in kwargs:
print(a, kwargs[a])
language(country="NL", code="+31")
country NL
code +31
Arguments in this keyword arbitrary arguments are passed as a dictionary whose keys
are keywords and values
are corresponding values. Like in the above example, the passed arguments are converted to a dictionary {'country':"NL", 'code':"+31"}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook