Python Tutorial - Decision Control
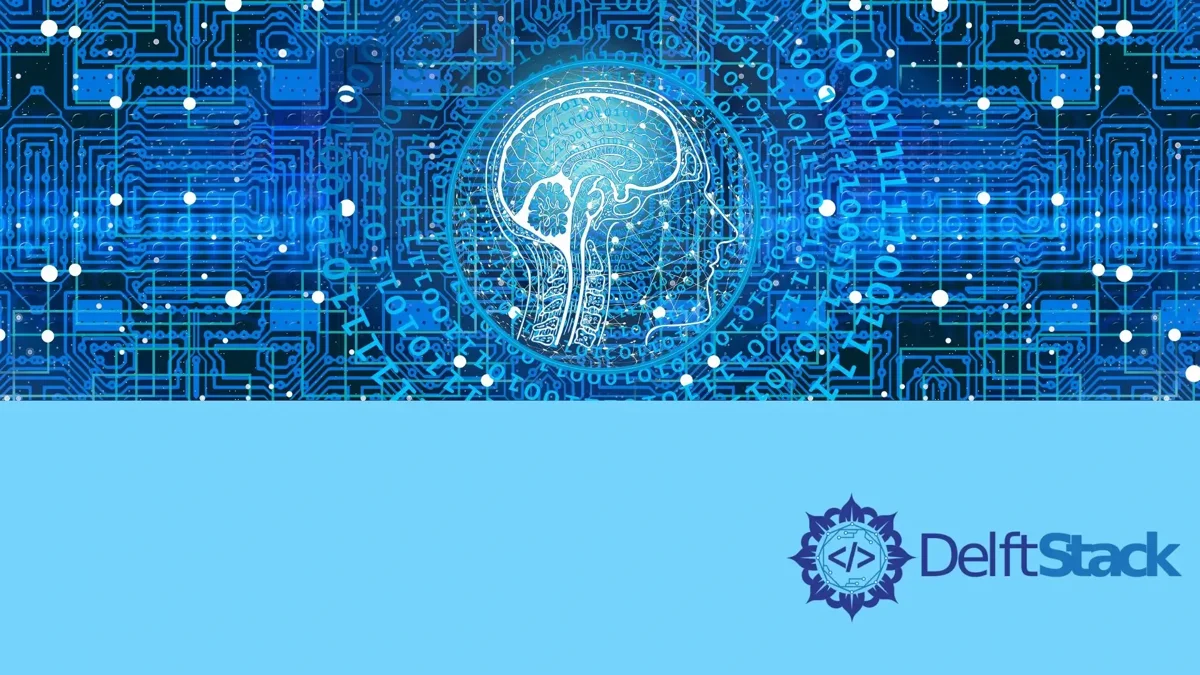
In this section, you will learn the decision-making construct in Python programming. The most used decision construct is an if…else
construct.
if…else
Statement
The if…else
statement is used when you have two blocks of statements and you want to execute only one based on some conditions. In Python programming, mostly if…elif…else
is used as a decision-making construct.
if
Statement
In if
statement you have only one block of statements and this block is executed only when the condition is True
, ignored when the condition is False
.
Below is the syntax of if
statement in Python:
if condition:
statement(s)
The body of if
statement in Python is not surrounded by curly braces, but instead, indentation is used. The end of the body is indicated by the first unintended line.
if
Statement Example
Consider the following example in which if
statement is used:
a = 24
if a % 2 == 0:
print(a, "is an even number")
b = 23
if b % 2 == 0:
print(b, "is an odd number")
24 is an even number
In this code, the variable a
is assigned a value first and then condition in if
statement is evaluated. It checks if a
is an even number or not by taking the modulus of a
with 2
and if the result of %
(mod) is 0 then control enter in the body of if
and the print
statement is executed.
Then b
is assigned an odd number 23
. The condition in if
statement is not True
, therefore print(b, "is an odd number")
will not be executed.
if...else
Statement
The following is the syntax of an if...else
statement:
if condition:
block of statements
else:
block of statements
In if...else
, if the if
the condition is True
, the corresponding block of statements is executed, otherwise the block of statements under else
part will be executed.
if...else
Statement Example
Consider the code below in which if...else
is used:
a = 44
if a % 2 == 0:
print(a, "is an even number")
else:
print(a, "is an odd number")
44 is an even number
Here if a
is even, it will print a is an even number
, otherwise it will print a is an odd number
.
if
and else
blocks couldn’t be either both executed or ignored. Only one block is executed based on whether the condition is True
or not.if...elif...else
Statement
The following is the syntax of if...elif...else
statement:
if condition:
block of statements
elif condition:
block of statements
else:
block of statements
elif
stands for else if
and it could be used multiple times in this if..elif..else
construct.
When the condition under if
becomes False
, the condition of elif
will be checked and so on. When all the conditions of if
and elif
are False
, else
part will be executed.
if...elif...else
Statement Example
Consider the code below in which we have used if...elif...else
statement which checks multiple conditions:
a = -34
if a > 0:
print("Number is Positive")
elif a < 0:
print("Number is Negative")
else:
print("Number is zero")
Number is Negative
Nested if
Statements
In Python, you can have an if
inside another if
statement. This is called nested if
statement.
You can nest any type of if
statement for any number of times. But it is not a good practice to use nested if
structures in programming languages as the readability of the program becomes worse.
Nested if
Statements Example
In the following code, nested if
structure is used to find the largest number:
T = 52
if T > 25:
if T < 50:
print("Temperature is higher than 25 but lower than 50")
else:
print("Temperature is higher than 50")
else:
if T < 0:
print("Temperature is lower than 0")
else:
print("Temperature is higher than 0 but lower than 25")
Temperature is higher than 50
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook