Python Tutorial - Class Inheritance
- Python Class Inheritance Method Overriding
- Python Class Multiple Inheritance
- Python Class Multilevel Inheritance
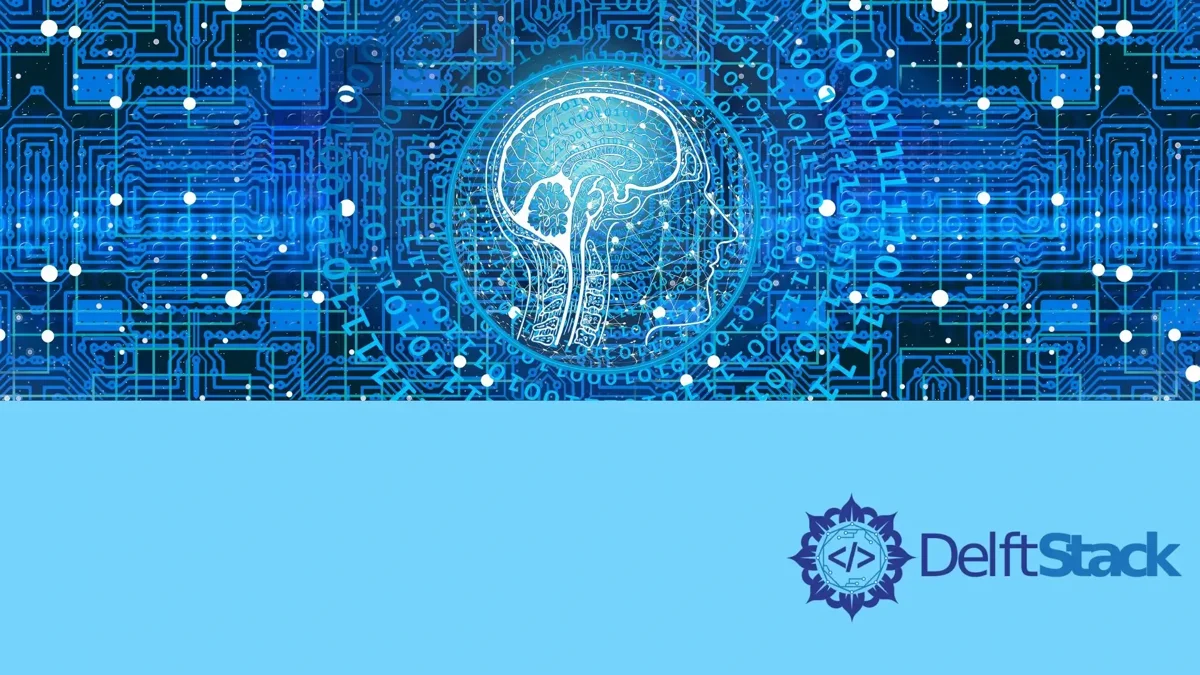
In this section, you will learn inheritance of Python object-oriented programming.
In Inheritance, a child class is created from a parent class. The child class inherits everything (data members and member functions) from the parent class.
The following is the syntax of inheritance in Python:
class ParentClass:
# members
class ChildClass(ParentClass):
# members
In the child class, you have all the features of the parent class and new functionalities can also be added.
Like in the example below, it has a parent class named Auto
and a child class car
is inherited from it.
>>> class Auto:
def __init__(self, e, n):
self.engine = e
self.name = n
def display(self):
print("Name of Auto: ", self.name)
print("Engine of auto: ", self.engine)
>>> class Car(Auto):
def __init__(self):
self.x= input("Enter name of car: ")
self.y= input("Enter engine of car: ")
Auto.__init__(self,self.y,self.x)
>>> c = Car()
Enter name of car: Prius
Enter engine of car: 1.5l
>>> c.display()
Name of Auto: Prius
Engine of auto: 1.5l
You can see here that the methods of Auto
class can be used in the Car
class. It is because Car
is inherited from Auto
.
Inside the constructor of Car
, the constructor of Auto
is called. The object of Car
can be used to call all methods of Auto
.
Python Class Inheritance Method Overriding
Method overriding is when you have the same methods for both child and parent classes. Method overriding is basically a concept in which a child class changes the implementation of methods of its parent class.
Consider the example where the child class Car
has the same display
method as in Auto
class but the implementation is changed:
>>> class Auto:
def __init__(self, e, n):
self.engine = e
self.name = n
def display(self):
print("Name of Auto: ", self.name)
print("Engine of auto: ", self.engine)
>>> class Car(Auto):
def __init__(self):
self.x= input("Enter name of car: ")
self.y= input("Enter engine of car: ")
Auto.__init__(self,self.y,self.x)
def display(self):
print("You are in child class")
>>> c = Car()
Enter name of car: Prius
Enter engine of car: 1.5l
>>> c.display()
You are in child class
So now when the display method is called in object c
, the method of the child class is invoked.
Python Class Multiple Inheritance
Multiple inheritance happens when a child class has more than one parent classes. The functionalities of each parent class are inherited by the child class.
The following is the syntax of multiple inheritance:
class A:
# members
class B:
# members
class child(A, B):
# members
Example:
>>> class A:
def dispA(self):
print('You are in class A')
>>> class B:
def dispB(self):
print('You are in class B')
>>> class C(A, B):
def dispC(self):
print('You are in class C')
>>> Cobj = C()
>>> Cobj.dispA()
You are in class A
>>> Cobj.dispB()
You are in class B
>>> Cobj.dispC()
You are in class C
It should be noticed that the object of C
(child class) can call the methods of its parent classes A
and B
. Therefore, you can say C inherits everything of A
and B
.
Python Class Multilevel Inheritance
Multilevel inheritance is when you inherit a child class from another child class.
The syntax of multilevel inheritance is as follows:
class A:
# members
class B(A):
# members
class C(B):
# members
Example:
>>> class A:
def dispA(self):
print('You are in class A')
>>> class B(A):
def dispB(self):
print('You are in class B')
>>> class C(B):
def dispC(self):
print('You are in class C')
>>> Cobj = C()
>>> Cobj.dispA()
You are in class A
>>> Cobj.dispB()
You are in class B
>>> Cobj.dispC()
You are in class C
You could see here that the C
class can invoke the methods of its parent and grandparent classes.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook