PyQt5 Tutorial - Radiobutton
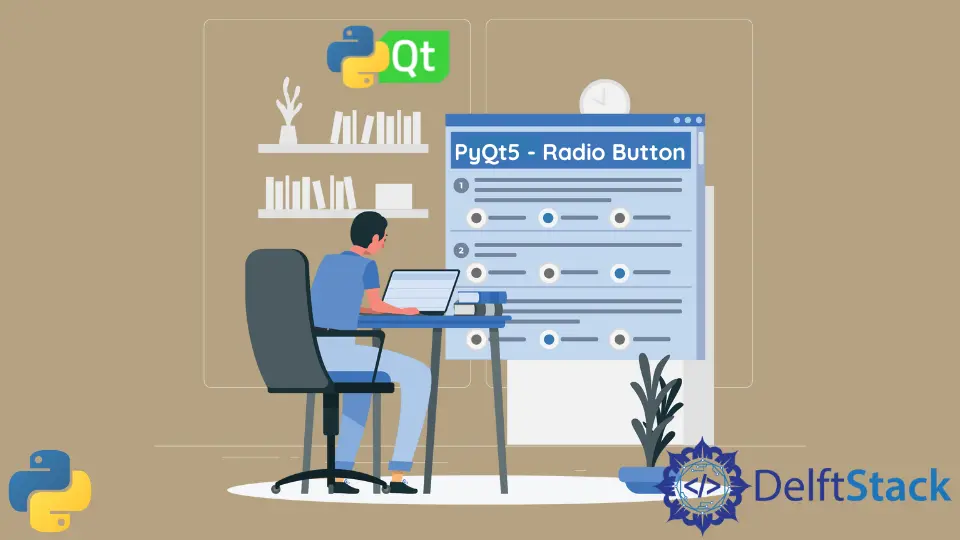
QRadiobutton
is similar to the QCheckbox
except that you’ve got more than one choice but you can only select one of them at one time. The QRadiobutton
widget has also the text label together with the round radio button.
PyQt5 QRadiobutton
Basic Example
The below code asks which city you live in and creates two radio buttons for the user to choose. It displays the in the label text after the user selects one of the radio buttons.
import sys
from PyQt5.QtWidgets import (
QLabel,
QRadioButton,
QPushButton,
QVBoxLayout,
QApplication,
QWidget,
)
class basicRadiobuttonExample(QWidget):
def __init__(self):
super().__init__()
self.init_ui()
def init_ui(self):
self.label = QLabel("Which city do you live in?")
self.rbtn1 = QRadioButton("New York")
self.rbtn2 = QRadioButton("Houston")
self.label2 = QLabel("")
self.rbtn1.toggled.connect(self.onClicked)
self.rbtn2.toggled.connect(self.onClicked)
layout = QVBoxLayout()
layout.addWidget(self.label)
layout.addWidget(self.rbtn1)
layout.addWidget(self.rbtn2)
layout.addWidget(self.label2)
self.setGeometry(200, 200, 300, 150)
self.setLayout(layout)
self.setWindowTitle("PyQt5 Radio Button Example")
self.show()
def onClicked(self):
radioBtn = self.sender()
if radioBtn.isChecked():
self.label2.setText("You live in " + radioBtn.text())
if __name__ == "__main__":
app = QApplication(sys.argv)
ex = basicRadiobuttonExample()
sys.exit(app.exec_())
self.rbtn1 = QRadioButton("New York")
self.rbtn2 = QRadioButton("Houston")
We create two radio buttons rbtn1
and rbtn2
. The string in the parenthesis is the text label shown next to the round radio button.
self.rbtn1.toggled.connect(self.onClicked)
self.rbtn2.toggled.connect(self.onClicked)
The button emits the toggled
signal every time the button is selected or deselected. The onClicked
function is connected to the toggled
signal of two radio buttons.
def onClicked(self):
radioBtn = self.sender()
if radioBtn.isChecked():
self.label2.setText("You live in " + radioBtn.text())
self.sender()
returns the widget who emits the signal and isChecked()
method in QRadiobutton
widget is True
if that specific button is selected, otherwise, it returns False
.
We could get the particular radio button that is selected from the group of radio buttons by checking the isChecked()
method.
PyQt5 QRadiobutton
setChecked
Method
The QRadiobutton
is not checked by default after it is initiated. We could change the default the state of the radio button to be Checked
during initialization by using setChecked()
method.
For example, we add the below line to the above example codes to check the radio button New York
.
self.rbtn1.setChecked(True)
PyQt5 QRadiobutton
Group
All the radio buttons in the same parent window are within the same group and it means that only one radio button could be selected at one time even the toggled
signals are connected to different functions.
QButtonGroup
from QtWidgets
groups the selected buttons together and we could create multiple QButtonGroup
instances to isolate radio buttons from different groups so that more buttons could be checked in the same parent widget.
The following shows the example to ask the user to select both city and state from two groups of radio buttons.
import sys
from PyQt5.QtWidgets import (
QLabel,
QRadioButton,
QPushButton,
QVBoxLayout,
QApplication,
QWidget,
QButtonGroup,
)
class basicRadiobuttonExample(QWidget):
def __init__(self):
super().__init__()
self.init_ui()
def init_ui(self):
self.label = QLabel("Which city do you live in?")
self.rbtn1 = QRadioButton("New York")
self.rbtn2 = QRadioButton("Houston")
self.label2 = QLabel("")
self.label3 = QLabel("Which state do you live in?")
self.rbtn3 = QRadioButton("New York")
self.rbtn4 = QRadioButton("Texas")
self.label4 = QLabel("")
self.btngroup1 = QButtonGroup()
self.btngroup2 = QButtonGroup()
self.btngroup1.addButton(self.rbtn1)
self.btngroup1.addButton(self.rbtn2)
self.btngroup2.addButton(self.rbtn3)
self.btngroup2.addButton(self.rbtn4)
self.rbtn1.toggled.connect(self.onClickedCity)
self.rbtn2.toggled.connect(self.onClickedCity)
self.rbtn3.toggled.connect(self.onClickedState)
self.rbtn4.toggled.connect(self.onClickedState)
layout = QVBoxLayout()
layout.addWidget(self.label)
layout.addWidget(self.rbtn1)
layout.addWidget(self.rbtn2)
layout.addWidget(self.label2)
layout.addWidget(self.label3)
layout.addWidget(self.rbtn3)
layout.addWidget(self.rbtn4)
layout.addWidget(self.label4)
self.setGeometry(200, 200, 300, 300)
self.setLayout(layout)
self.setWindowTitle("PyQt5 Radio Button Example")
self.show()
def onClickedCity(self):
radioBtn = self.sender()
if radioBtn.isChecked():
self.label2.setText("You live in " + radioBtn.text())
def onClickedState(self):
radioBtn = self.sender()
if radioBtn.isChecked():
self.label4.setText("You live in " + radioBtn.text())
if __name__ == "__main__":
app = QApplication(sys.argv)
ex = basicRadiobuttonExample()
sys.exit(app.exec_())
self.btngroup1 = QButtonGroup()
self.btngroup2 = QButtonGroup()
Two button groups are created to hold the radio buttons.
self.btngroup1.addButton(self.rbtn1)
self.btngroup1.addButton(self.rbtn2)
self.btngroup2.addButton(self.rbtn3)
self.btngroup2.addButton(self.rbtn4)
The radio buttons in the same groups are added to the same QButtonGroup
.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook