Tkinter Tutorial - Entry
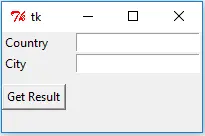
Tkinter Entry
widget lets the user enter a single line of text that has only one font type. If more lines are required, you should use Tkinter Text
widget. Entry
widget could also be used to display the single-line text.
Tkinter Entry Example
import tkinter as tk
from tkinter import ttk
def callbackFunc():
resultString.set("{} - {}".format(landString.get(), cityString.get()))
app = tk.Tk()
app.geometry("200x100")
labelLand = tk.Label(app, text="Country")
labelLand.grid(column=0, row=0, sticky=tk.W)
labelCity = tk.Label(app, text="City")
labelCity.grid(column=0, row=1, sticky=tk.W)
landString = tk.StringVar()
cityString = tk.StringVar()
entryLand = tk.Entry(app, width=20, textvariable=landString)
entryCity = tk.Entry(app, width=20, textvariable=cityString)
entryLand.grid(column=1, row=0, padx=10)
entryCity.grid(column=1, row=1, padx=10)
resultButton = tk.Button(app, text="Get Result", command=callbackFunc)
resultButton.grid(column=0, row=2, pady=10, sticky=tk.W)
resultString = tk.StringVar()
resultLabel = tk.Label(app, textvariable=resultString)
resultLabel.grid(column=1, row=2, padx=10, sticky=tk.W)
app.mainloop()
This example code creates a GUI that the user could enter the country and city names, then it displays the entered information after Get Result
button is clicked.
entryLand = tk.Entry(app, width=20, textvariable=landString)
It creates one Tkinter Entry
widget instance whose width is 20 character units. It could only display 20 characters in the entry box, therefore, you need to use arrow keys to display the rest of the line if the text line has more than 20 characters.
The text of the entry widget is assigned to a Tkinter string variable landString
. You could get the text with landString.get()
method, and set the text with landString.set()
method. The text in the entry box updates automatically if set()
method is used.
get()
method of StringVar
, you could also use get()
method of Entry
widget object to retrieve the string in Entry
box.Tkinter Entry Default Text
We have two ways to set the Entry
default text,
Set()
Method to Set the Default Text of Tkinter Entry
As indicated in the above example, you could use set()
method of StringVar
to set the default text of Tkinter Entry
.
For example,
landString.set("Netherlands")
It will set the default text as Netherlands
.
Insert()
Method to Set Tkinter Entry
Default Text
insert(index, string)
method inserts the text string
at the given index
position. And if index
is END
, it appends the text to the Entry
widget.
entryLand.insert(tk.END, "Netherlands")
It sets the default text to be Netherlands
.
index
is larger than the length of existing string in the widget, it is equal to insert(END, string)
.Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook