How to Convert Int to Bytes in Python 2 and Python 3
-
Python 2.7 and 3 Compatible
int
tobytes
Conversion Method -
Python 3 Only
int
tobytes
Conversion Methods - Performance Comparison
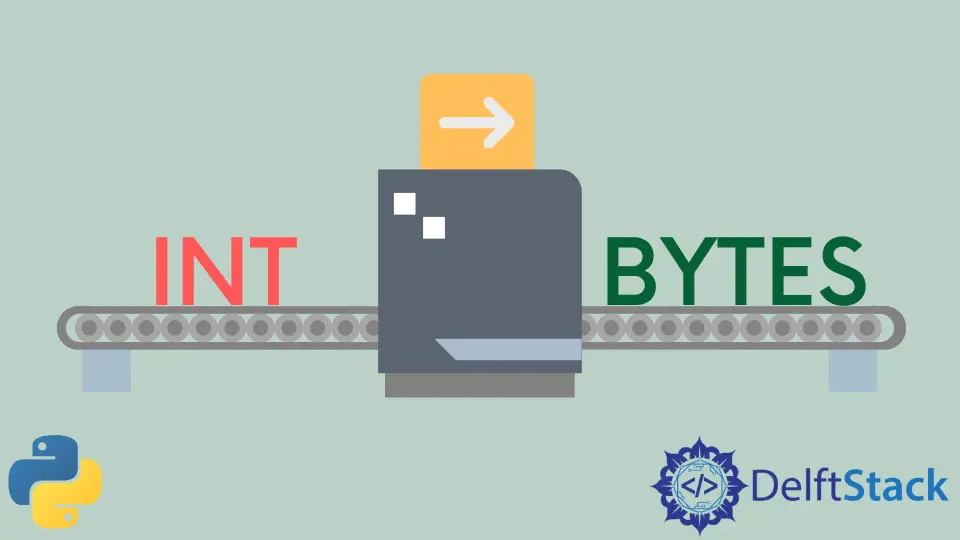
Conversion from int
to bytes
is the reverse operation of conversion from bytes
to int
that is introduced in the last HowTo tutorial. Most of the int-to-bytes methods introduced in this article are the reverse methods of bytes-to-int methods.
Python 2.7 and 3 Compatible int
to bytes
Conversion Method
You could use pack
function in the Python struct module to convert the integer to bytes in the specific format.
>>> import struct
>>> struct.pack("B", 2)
'\x02'
>>> struct.pack(">H", 2)
'\x00\x02'
>>> struct.pack("<H", 2)
'\x02\x00'
The first argument in the struct.pack
function is the format string that specifies the bytes format like byte length, sign, byte order (little or big endian), etc.
Python 3 Only int
to bytes
Conversion Methods
Use bytes
to Convert int
to bytes
As indicated in the last article, bytes
is a built-in data type from Python 3. You could easily use bytes
to convert the integer 0~255 to bytes data type.
>>> bytes([2])
b'\x02'
The integer must be surrounded by the parenthesis, otherwise, you will get the bytes object of size given by the parameter initialized with null bytes
but not the corresponding bytes.
>>> bytes(3)
b'\x00\x00\x00'
Use int.to_bytes()
Method to Convert int
to bytes
From Python3.1, a new integer class method int.to_bytes()
is introduced. It is the reverse conversion method of int.from_bytes()
as discussed in the last article.
>>> (258).to_bytes(2, byteorder="little")
b'\x02\x01'
>>> (258).to_bytes(2, byteorder="big")
b'\x01\x02'
>>> (258).to_bytes(4, byteorder="little", signed=True)
b'\x02\x01\x00\x00'
>>> (-258).to_bytes(4, byteorder="little", signed=True)
b'\xfe\xfe\xff\xff'
The first argument is the converted bytes data length, the second argument byteorder
defines the byte order to be little or big-endian, and the optional argument signed
determines whether two’s complement is used to represent the integer.
Performance Comparison
In Python 3, you have 3 ways to convert int
to bytes
,
bytes()
methodstruct.pack()
methodint.to_bytes()
method
We will check the execution time of each method to compare their performance, and finally give you the recommendation if you want to increase your code execution speed.
>>> import timeint
>>> timeit.timeit('bytes([255])', number=1000000)
0.31296982169325455
>>> timeit.timeit('struct.pack("B", 255)', setup='import struct', number=1000000)
0.2640925447800839
>>> timeit.timeit('(255).to_bytes(1, byteorder="little")', number=1000000)
0.5622947660224895
Conversion Method | -Execution Time (1 million times) |
---|---|
bytes() |
0.31296982169325455 s |
struct.pack() |
0.2640925447800839 s |
int.to_bytes() |
0.5622947660224895 s |
Therefore, please use struct.pack()
function to perform the int-to-bytes conversion to get the best execution performance, although it is already introduced in Python 2 branch.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook