How to Create Full Screen Window in Tkinter
-
Windows
root.attributes('-fullscreen', True)
to Create Full Screen Mode in Tkinter -
Ubuntu
root.attributes('-zoomed', True)
to Create Full Screen Mode in Tkinter - Display Full Screen Mode With Shown Tool Bar
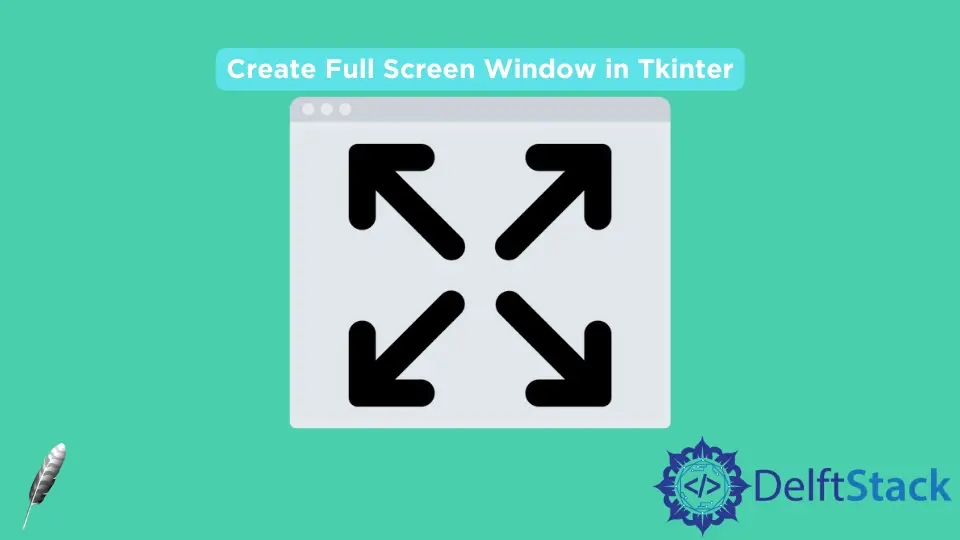
In this tutorial, we will introduce how to create full screen window in Tkinter, and how to toggle or quit full screen mode.
Windows root.attributes('-fullscreen', True)
to Create Full Screen Mode in Tkinter
tk.Tk.attributes
sets platform specific attributes. The attributes in Windows are,
-alpha
-transparentcolor
-disabled
-fullscreen
-toolwindow
-topmost
-fullscreen
specifies whether the window is full-screen mode or not.
import tkinter as tk
class Fullscreen_Example:
def __init__(self):
self.window = tk.Tk()
self.window.attributes("-fullscreen", True)
self.fullScreenState = False
self.window.bind("<F11>", self.toggleFullScreen)
self.window.bind("<Escape>", self.quitFullScreen)
self.window.mainloop()
def toggleFullScreen(self, event):
self.fullScreenState = not self.fullScreenState
self.window.attributes("-fullscreen", self.fullScreenState)
def quitFullScreen(self, event):
self.fullScreenState = False
self.window.attributes("-fullscreen", self.fullScreenState)
if __name__ == "__main__":
app = Fullscreen_Example()
self.window.bind("<F11>", self.toggleFullScreen)
F11 is bound to the function toggleFullScreen
.
def toggleFullScreen(self, event):
self.fullScreenState = not self.fullScreenState
self.window.attributes("-fullscreen", self.fullScreenState)
The fullscreen
mode is updated to the toggled state in this function.
def quitFullScreen(self, event):
self.fullScreenState = False
self.window.attributes("-fullscreen", self.fullScreenState)
quitFullScreen
function quits the full screen mode by setting -fullscreen
to be False
.
We could use lambda
function to make the solution simpler
import tkinter as tk
class Fullscreen_Example:
def __init__(self):
self.window = tk.Tk()
self.window.attributes("-fullscreen", True)
self.window.bind(
"<F11>",
lambda event: self.window.attributes(
"-fullscreen", not self.window.attributes("-fullscreen")
),
)
self.window.bind(
"<Escape>", lambda event: self.window.attributes("-fullscreen", False)
)
self.window.mainloop()
if __name__ == "__main__":
app = Fullscreen_Example()
self.window.bind(
"<F11>",
lambda event: self.window.attributes(
"-fullscreen", not self.window.attributes("-fullscreen")
),
)
It binds the lambda
function to F11, where the current full screen state could be read by self.window.attributes("-fullscreen")
that returns the state if no value is given in the method.
We don’t need the state variable fullScreenState
any more if we follow this approach.
Ubuntu root.attributes('-zoomed', True)
to Create Full Screen Mode in Tkinter
-fullscreen
attribute only exists on Windows but not on Linux or macOS. Ubuntu has a comparable attribute -zoomed
to set the window in full screen mode.
import tkinter as tk
class Ubuntu_Fullscreen_Example:
def __init__(self):
self.window = tk.Tk()
self.window.attributes("-zoomed", True)
self.fullScreenState = False
self.window.bind("<F11>", self.toggleFullScreen)
self.window.bind("<Escape>", self.quitFullScreen)
self.window.mainloop()
def toggleFullScreen(self, event):
self.fullScreenState = not self.fullScreenState
self.window.attributes("-zoomed", self.fullScreenState)
def quitFullScreen(self, event):
self.fullScreenState = False
self.window.attributes("-zoomed", self.fullScreenState)
if __name__ == "__main__":
app = Ubuntu_Fullscreen_Example()
Display Full Screen Mode With Shown Tool Bar
The full screen mode shown in above codes makes the tool bar invisible. If we need to show tool bar in the window, the geometry of the window shall be the same with the monitor size.
import tkinter as tk
class Fullscreen_Example:
def __init__(self):
self.window = tk.Tk()
self.fullScreenState = False
self.window.attributes("-fullscreen", self.fullScreenState)
self.w, self.h = (
self.window.winfo_screenwidth(),
self.window.winfo_screenheight(),
)
self.window.geometry("%dx%d" % (self.w, self.h))
self.window.bind("<F11>", self.toggleFullScreen)
self.window.bind("<Escape>", self.quitFullScreen)
self.window.mainloop()
def toggleFullScreen(self, event):
self.fullScreenState = not self.fullScreenState
self.window.attributes("-fullscreen", self.fullScreenState)
def quitFullScreen(self, event):
self.fullScreenState = False
self.window.attributes("-fullscreen", self.fullScreenState)
if __name__ == "__main__":
app = Fullscreen_Example()
self.w, self.h = self.window.winfo_screenwidth(), self.window.winfo_screenheight()
winfo_screenwidth()
and winfo_screenheight()
get the width and height of the monitor.
self.window.geometry("%dx%d" % (self.w, self.h))
It sets the GUI window size the same as the monitor width and height, by using geometry
method.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook