How to Generate Password in PHP
-
Use the
rand()
Function to Select a Random Array Index to Generate a Random Password in PHP -
Use the
openssl_random_pseudo_bytes()
and thebin2hex()
Functions to Generate Random Password in PHP -
Use the
substr()
and thestr_shuffle()
Functions to Generate Random Password From a Given Alphanumerical String in PHP
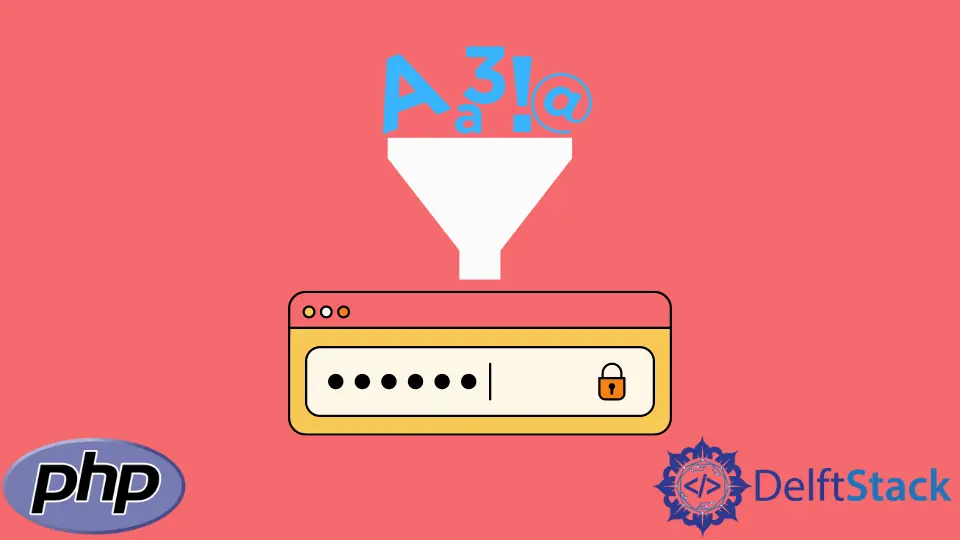
We will introduce a method to generate random passwords in PHP using the rand()
function. This method uses the combination of the lowercase and uppercase alphabets and numbers to form a password. The rand()
function returns the random integer, which is the array index, to chose the password combination.
We will also introduce another method to generate random passwords in PHP using the openssl_random_pseudo_bytes()
and the bin2hex()
functions.
This article will demonstrate another method to generate random passwords in PHP using the substr()
and the str_shuffle()
functions. This method also uses the alphanumeric values provided in the program to form a random password.
Use the rand()
Function to Select a Random Array Index to Generate a Random Password in PHP
We can use the rand()
function to generate a random password in PHP. The rand()
function returns a random integer from the range of the parameters provided. The function takes two integer values as the parameters. We can define a string of the alphabets and numbers from where we can combine the password. The random number indexes the array to pick random alphabets or numbers. We can loop the rand()
function to create the password of the desired length.
For example, create a variable $comb
and store the string comprising all lowercase and uppercase alphabets and the numbers from 0 to 10 as abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890
. Create an array $pass
with the array()
function. Store the length of the string $comb
in the $combLen
variable. Create a for
loop and iterate the loop eight times. Inside the loop, use the rand()
function with 0
and the $combLen
variable as the parameters and store the value in the $n
variable. Then, assign the $comb[$n]
to the $pass
array. Outside the loop, convert the array into the string using the implode()
function.
In the example below, we generate a random password from the combination stored in the $comb
variable. The rand()
function picks up a random integer from 0 to the string $comb
length. The random integer is used as an index to pick up the random alphanumeric value from the combination. The loop runs eight times and picks eight random alphanumeric values, and finally forms a random password. Check the PHP Manual to learn more about the rand()
function.
Example Code:
# php 7.x
<?php
$comb = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890';
$pass = array();
$combLen = strlen($comb) - 1;
for ($i = 0; $i < 8; $i++) {
$n = rand(0, $combLen);
$pass[] = $comb[$n];
}
print(implode($pass));
?>
Output:
aPsQWlzh
Use the openssl_random_pseudo_bytes()
and the bin2hex()
Functions to Generate Random Password in PHP
We can use the openssl_random_pseudo_bytes()
function to generate a pseudo-random string of bytes. We can specify an integer value as the function’s parameter to define the length of the desired number of bytes to be generated. The bin2hex()
function converts the binary data to the hexadecimal representations. We can use this function to convert the randomly generated string of bytes to the hexadecimal representation and form a password. This method generates the random password comprising of the alphanumerical values.
For example, create a variable $bytes
and assign it with the function openssl_random_pseudo_bytes()
. Specify 4
as the parameter to the function. Create another variable, $pass
, and write the function bin2hex()
to it. Specify the $bytes
variable in the function. Print the $pass
variable using the echo
statement.
In the example below, the 4
in the openssl_random_pseudo_bytes()
function denotes the number of bytes to be randomly generated. The bin2hex()
function converts the bytes into hexadecimal equivalent. The randomly generated password contains the length of 8 because the hexadecimal conversion of 4 bytes of string results in 8. Check the PHP Manual to know more about the bin2hex()
function.
Example Code:
#php 7.x
<?php
$bytes = openssl_random_pseudo_bytes(4);
$pass = bin2hex($bytes);
echo $pass;
?>
Output:
ed203eef
Use the substr()
and the str_shuffle()
Functions to Generate Random Password From a Given Alphanumerical String in PHP
We can use the str_shuffle()
method to randomly shuffle the given string. The function shuffles the string of a combination of lowercase and uppercase alphabets along with the numbers from 0 to 9. Thus, it forms one permutation of all the possible cases. We can use the substr()
function to the shuffled string to pick a portion of its characters. Thus, we can generate a random password.
For example, create a variable $comb
and store the string value abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789
in it. Use the str_shuffle()
to shuffle the $comb
variable and store it in $shfl
variable. Then, use the substr()
function and the $shfl
variable, 0
, and 8
as the parameters. Store the function in a $pwd
variable and print the variable.
In the example below, we use the same set of alphanumeric values as the first method. The randomly generated password is formed from these alphanumeric values. The str_shuffle()
function shuffles the alphanumeric value and creates a random permutation. We have used 0
and 8
in the substr()
function to select the eight characters from the first index of the shuffled permutation. Thus, it generates an eight-digit random password.
Code Example:
#php 7.x
<?php
$comb = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789";
$shfl = str_shuffle($comb);
$pwd = substr($shfl,0,8);
echo $pwd;
?>
Output:
jenZq3lY
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn