How to Upload Multiple Files in PHP
-
Use the
multiple
Tag, an Array in thename
Tag of theinput
Attribute in the Form and themove_uploaded_file()
Function to Upload Multiple File in PHP - Use the PDO to Upload the Multiple Files in the Database in PHP
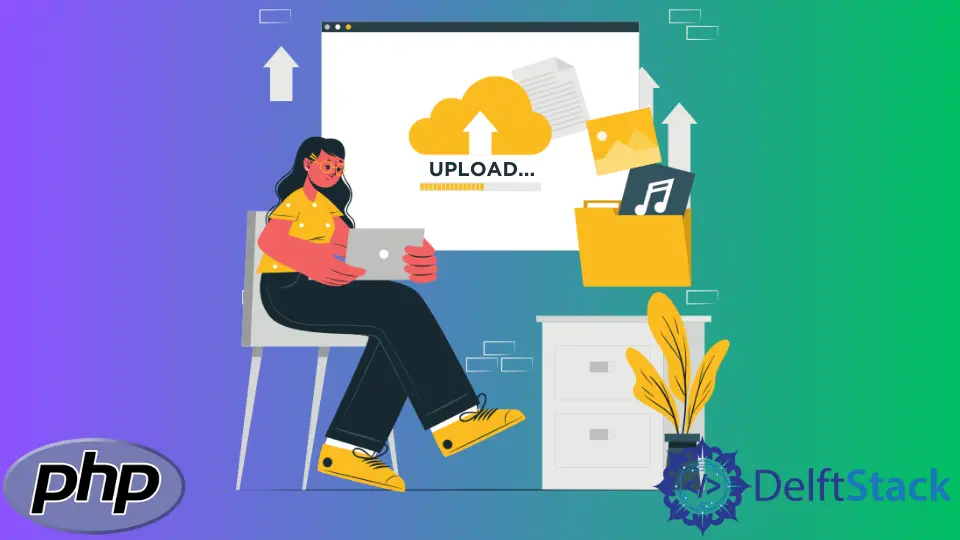
We will introduce a method to upload multiple files in PHP by specifying the name
attribute of the input
tag as an array and using the multiple
attribute. The value of the enctype
attribute of the form
tag is multipart/form-data
. This method uses mysqli
for the database connection.
We will also introduce another method to upload multiple files in PHP using the PDO. We will upload the files in a folder and then upload them to a database. This method is similar to the first method in terms of implementation.
Use the multiple
Tag, an Array in the name
Tag of the input
Attribute in the Form and the move_uploaded_file()
Function to Upload Multiple File in PHP
We can specify the name
attribute of the input
tag as an array to upload multiple files. The input
tag uses the multiple
keyword that lets us select the multiple file while uploading. We can write the encoding multipart/form-data
for the enctype
attribute to specify how the form-data should be encoded while submitting the form. We can use the mysqli
to connect to the database. The move_uploaded_file()
function moves the uploaded file from a temporary location of the server to the desired location. We can use the INSERT
SQL statements to upload the selected file to the database.
For example, set a database connection with the mysqli
object and assign the value to the $db
variable. Create an HTML form with POST
method and encoding type as multipart/form-data
to upload the files. Specify the type
attribute of the input
tag as file
and the name
attribute as file[]
. Do not forget to write the attribute multiple
before closing the input
tag. Write the input
tag to submit the form.
Check whether the form has been submited with the isset()
function. Use the count
function to count the number of uploaded files. Take the $_FILES['file']['name']
as the parameter for the count
function and assign it to a variable $countfiles
. Use the for loop to loop over the uploaded file. Inside the loop, create a variable $filename
and assign it as $_FILES['file']['name'][$i]
. Create a folder named upload
in the root directory. Use the move_upload_file()
function to move the file. Use $_FILES['file']['tmp_name'][$i]
as the first parameter which is the file with temporary name. Use 'upload/'.$filename
as the second parameter which is the filename and the location to store the uploaded file.
Run a SQL INSERT
query to insert the files into the database. Insert the variable $filename
as both id
and name
into the fileup
table in the database. Use the$db
variable to call the query()
function with the $sql
variable as the parameter to execute the query.
Example Code:
# php 7.*
<?php
if(isset($_POST['submit'])){
$countfiles = count($_FILES['file']['name']);
for($i=0;$i<$countfiles;$i++){
$filename = $_FILES['file']['name'][$i];
$sql = "INSERT INTO fileup(id,name) VALUES ('$filename','$filename')";
$db->query($sql);
move_uploaded_file($_FILES['file']['tmp_name'][$i],'upload/'.$filename);
}
}
?>
<form method='post' action='' enctype='multipart/form-data'>
<input type="file" name="file[]" id="file" multiple>
<input type='submit' name='submit' value='Upload'>
</form>
?>
Output:
SELECT * FROM `fileup`
id name
1 cfc.jpg
2 hills.jpg
Use the PDO to Upload the Multiple Files in the Database in PHP
We can use the PDO to upload multiple files in PHP. We can use the PDO object to create a connection to the database. We use the prepared statements to insert the file into the database. This method is only different from the first method in terms of the database connection. We can use the exact HTML form for this method too. We demonstrate this method by uploading two jpg files in the folder and the database.
For example, establish a PDO database connection using the new
keyword and assign it to $conn
variable. Check whether the form has been submitted with the isset()
function. Count the files using the count
function as above. Then, write a query on the $query
variable to insert the id
and name
. Write the placeholders ?
for the values in the query. Prepare the query using the prepare()
function and assign the value in $statement
variable. Move the each file to the uplaod
folder using the move_uploaded_file()
function. Execute the query using the execute()
function. Supply an array with the $filename
and $target_file
as the parameters in the function.
In the example below, the user uploads two jpg files. Firstly, the file gets uploaded to the upload
folder, and then it gets uploaded to the database. The database contains the table fileup
, and it has the columns id
and name
. To learn more about the move_uploaded_files()
function, please refer to the PHP Manual
Example Code:
#php 7.x
<?php
$conn = new PDO("mysql:host=$server;dbname=$dbname","$username","$password");
$conn->setAttribute(PDO::ATTR_ERRMODE,PDO::ERRMODE_EXCEPTION);
if(isset($_POST['submit'])){
$countfiles = count($_FILES['file']['name']);
$query = "INSERT INTO fileup (id, name) VALUES(?,?)";
$statement = $conn->prepare($query);
for($i=0;$i<$countfiles;$i++){
$filename = $_FILES['file']['name'][$i];
$target_file = 'upload/'.$filename;
move_uploaded_file($_FILES['file']['tmp_name'][$i],$target_file);
$statement->execute(array($filename,$target_file));
}
}
?>
Output:
SELECT * FROM `fileup`
id name
1 upload/cfc.jpg
2+ upload/count.jpg
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn