How to Zip NumPy Arrays
-
NumPy Zip With the
list(zip())
Function -
NumPy Zip With the
numpy.stack()
Function -
NumPy Zip With the
numpy.column_stack()
Function -
NumPy Zip With the
np.vstack()
Function -
NumPy Zip With
np.concatenate
,np.newaxis
, andreshape
- Conclusion
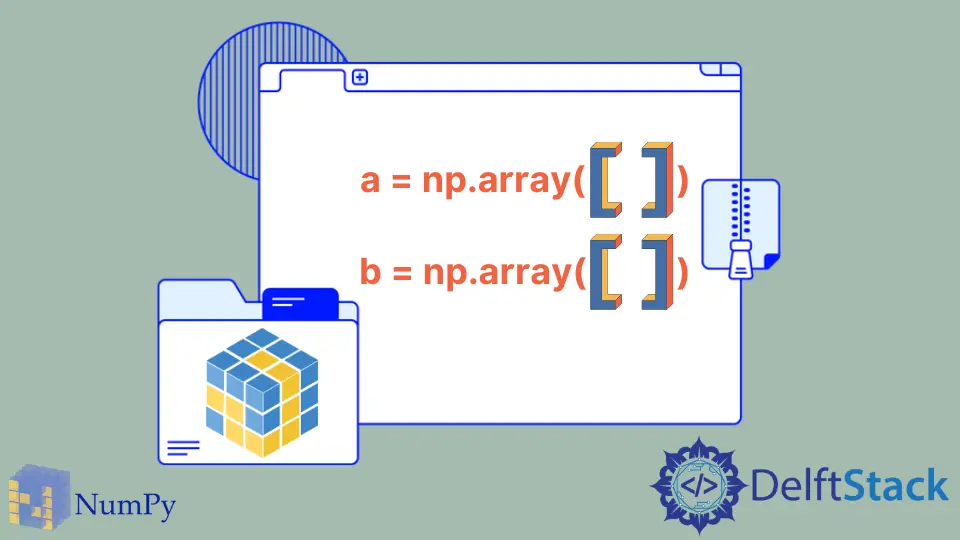
NumPy, the fundamental package for scientific computing in Python, offers various techniques to merge two 1D NumPy arrays into a single 2D NumPy array.
This article explores several methods to achieve this, each with its advantages and use cases.
NumPy Zip With the list(zip())
Function
The zip
function in Python allows you to combine multiple iterables element-wise, creating tuples that contain corresponding elements from the input iterables.
When you use list(zip())
with two NumPy arrays, you can merge them into a list of tuples. The real magic happens when you convert this list of tuples into a NumPy array.
Let’s illustrate this with a simple example. Consider two 1D NumPy arrays, a
and b
:
import numpy as np
a = np.array([1, 3, 5, 7])
b = np.array([2, 4, 6, 8])
You can employ the list(zip())
function to pair the elements of a
and b
together, creating a list of tuples:
c = np.array(list(zip(a, b)))
Here’s what each parameter in the syntax means:
-
arrays
: A sequence of arrays that you want to stack together. It can be a tuple, list, or any iterable containing the arrays you want to stack. -
axis
(optional): Specifies the axis along which the arrays will be stacked. By default,axis
is set to0
, which means that the arrays are stacked along a new axis, creating a new dimension in the result.If you specify
axis=1
, the arrays will be stacked horizontally. You can adjust theaxis
value to control the orientation of the result. -
out
(optional): Allows you to specify an output array where the result will be stored. Ifout
is not provided or isNone
, a new array will be created to store the result. -
where
(optional): A condition that defines where the result is taken from. By default, it is set toTrue
, meaning that the result will be taken from all locations.
You can use this parameter to conditionally select elements from the input arrays.
As mentioned, by specifying the axis
parameter, you can control the orientation of the resulting array. In the context of merging 1D NumPy arrays into a 2D NumPy array, you can set the axis=1
to obtain a similar result as the previous example.
Let’s dive into a practical example. Consider two 1D NumPy arrays, a
and b
:
import numpy as np
a = np.array([1, 2, 3, 4, 5])
b = np.array([6, 7, 8, 9, 10])
You can use the numpy.stack()
function to seamlessly merge the elements of a
and b
into a 2D NumPy array:
c = np.stack((a, b), axis=1)
In this step, c
is a 2D NumPy array:
array([[1, 6],
[2, 7],
[3, 8],
[4, 9],
[5, 10]])
Unlike the previous approach involving list(zip())
, the use of numpy.stack()
is more efficient as it eliminates the need for type conversion between lists and arrays. This efficiency can be especially advantageous when working with larger datasets and more complex tasks.
Here’s a breakdown of the key features of this approach:
-
Efficiency:
numpy.stack()
operates directly on NumPy arrays, reducing unnecessary conversions and enhancing overall performance. -
Axis Control: You can specify the
axis
parameter to control how the arrays are stacked. In this case,axis=1
results in a horizontal stacking.
NumPy Zip With the numpy.column_stack()
Function
The numpy.column_stack()
function is also an efficient and straightforward approach to merging two 1D NumPy arrays into a single 2D NumPy array. This method streamlines the process, eliminating the need for explicit axis specification and type conversion.
The numpy.column_stack()
function is a specialized tool within the NumPy arsenal designed for the precise task of joining two or more 1D arrays as columns into a single 2D array.
Unlike some other methods, you don’t need to specify an axis parameter with this approach. It’s a streamlined way to merge arrays without the need for explicit orientation control.
Syntax:
numpy.column_stack(tup)
Here’s what the parameter in the syntax means:
tup
: This is a sequence of arrays that you want to stack as columns. It can be a tuple, list, or any iterable containing the arrays you want to merge.
Let’s illustrate this with a practical example. Consider two 1D NumPy arrays, a
and b
:
import numpy as np
a = np.array([1, 3, 5, 7])
b = np.array([2, 4, 6, 8])
You can utilize the numpy.column_stack()
function to seamlessly merge the elements of a
and b
into a 2D NumPy array:
d = np.column_stack((a, b))
Here’s what the parameter in the syntax means:
tup
: This is a tuple of arrays that you want to stack vertically. You can provide multiple arrays in the tuple to stack them in a vertically concatenated form.
Let’s illustrate the process with a practical example. Consider two 1D NumPy arrays, array1
and array2
:
import numpy as np
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])
You can employ the np.vstack
function to stack the elements of array1
and array2
vertically, creating a single 2D NumPy array:
result = np.vstack((array1, array2))
In this step, the result
is a 2D NumPy array:
array([[1, 2, 3],
[4, 5, 6]])
The np.vstack
function is not only straightforward to use but also highly versatile. It can handle arrays of different shapes as long as they are compatible along the vertical axis.
This means you can combine arrays of varying lengths as long as the dimensions match along the axis you’re stacking.
NumPy Zip With np.concatenate
, np.newaxis
, and reshape
To combine two 1D NumPy arrays into a single 2D NumPy array, we can also use np.concatenate
to stack the arrays along a specified axis. We will also explore two different techniques:
-
Using
np.concatenate
withnp.newaxis
: This method involves introducing a new axis to the 1D arrays, effectively converting them into 2D arrays, and then concatenating them. -
Using
np.concatenate
withreshape
: Here, we will reshape the 1D arrays to give them the desired dimensions for concatenation.
Syntax of np.concatenate
:
np.concatenate((arrays), axis=0)
arrays
: A sequence of arrays that you want to concatenate.axis
: Specifies the axis along which the arrays will be concatenated. The default value is0
, indicating concatenation along the first axis.
Syntax of np.newaxis
:
array[:, np.newaxis]
array
: A NumPy array to which you want to add a new axis.
Syntax of reshape
:
array.reshape(newshape)
array
: The NumPy array that you want to reshape.newshape
: Defines the new shape of the array. It could be a tuple or an integer.
Let’s illustrate both of these techniques with a practical example. Consider two 1D NumPy arrays, array1
and array2
:
import numpy as np
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])
To merge the arrays using np.concatenate
with np.newaxis
, you first introduce a new axis to each of the 1D arrays and then concatenate them along this new axis:
result = np.concatenate((array1[:, np.newaxis], array2[:, np.newaxis]), axis=1)
In this code block, we achieve the same result using a slightly different approach.
Here, we use the reshape
method to reshape array1
into a 2D column vector. The -1
is used to indicate that the size of that dimension should be inferred from the length of the original array, ensuring it’s reshaped properly.
Similarly, we reshape array2
into a 2D column vector. As with the previous approach, we use np.concatenate
to concatenate the two 2D column vectors along axis=1
, resulting in a 2D NumPy array that combines the elements of array1
and array2
in a structured format.
The final result is, once again, the same 2D NumPy array:
array([[1, 4],
[2, 5],
[3, 6]])
The use of np.concatenate
along with np.newaxis
or reshape
provides you with the flexibility to adjust the shape and dimension of your arrays as needed, allowing you to tackle a wide range of data manipulation tasks.
Conclusion
In this article, we’ve covered multiple techniques for merging two 1D NumPy arrays into a single 2D NumPy array. Whether you prefer the simplicity of list(zip())
, the efficiency of numpy.stack()
, the ease of numpy.column_stack()
, or the versatility of np.vstack()
, NumPy provides solutions for various use cases.
Additionally, we’ve demonstrated how to use np.concatenate
in combination with np.newaxis
or reshape
for ultimate flexibility in shaping and combining arrays. With these methods at your disposal, you can efficiently manipulate and merge NumPy arrays to meet the requirements of your data processing tasks, whether working with small or large datasets.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn