How to Count Unique Values in NumPy Array
- Using numpy.unique() to Count Unique Values
- Counting Unique Values with a Dictionary
- Using Pandas for Counting Unique Values
- Conclusion
- FAQ
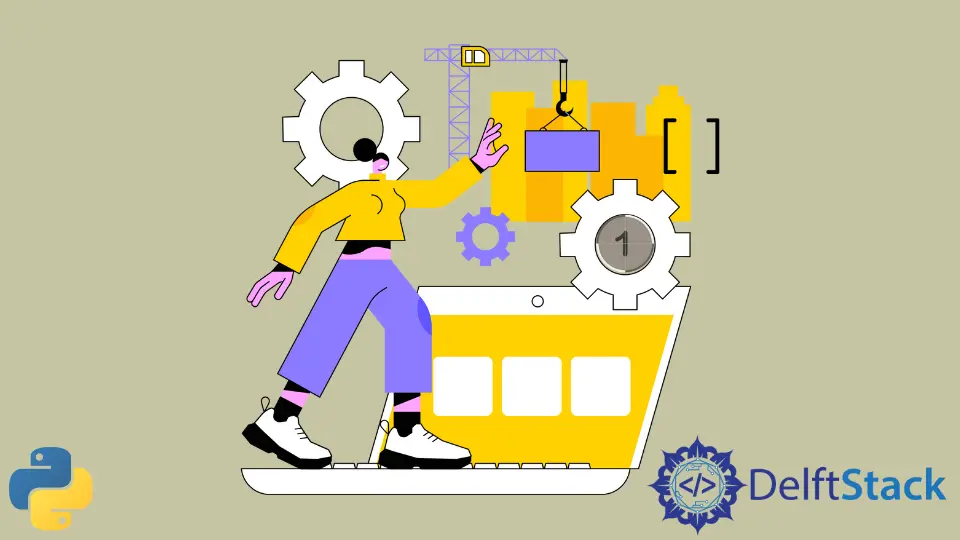
When working with data in Python, especially in scientific computing or data analysis, you often need to know how many unique values are present in an array. This is where NumPy shines, offering powerful tools to handle arrays efficiently. One of the most useful functions for this purpose is numpy.unique()
. This function not only identifies unique elements in a NumPy array but also counts the occurrences of each unique item. Whether you’re analyzing survey results, processing sensor data, or working with any dataset, being able to count unique values is essential.
In this article, we’ll explore how to use the numpy.unique()
function effectively, alongside other methods, to count unique values in a NumPy array.
Using numpy.unique() to Count Unique Values
The numpy.unique()
function is the most straightforward way to count unique values in a NumPy array. This function returns the unique values in the array and can also provide the counts of each unique value if specified. Let’s dive into how it works with a simple example.
import numpy as np
array = np.array([1, 2, 2, 3, 4, 4, 4, 5])
unique_values, counts = np.unique(array, return_counts=True)
print("Unique values:", unique_values)
print("Counts:", counts)
Output:
Unique values: [1 2 3 4 5]
Counts: [1 2 1 3 1]
In this example, we first import the NumPy library and create a NumPy array containing some values. The np.unique()
function is called with the return_counts=True
argument, which tells the function to return both the unique values and their respective counts. The output shows that the unique values in the array are 1, 2, 3, 4, and 5, with their counts being 1, 2, 1, 3, and 1, respectively. This method is efficient and concise, making it ideal for quick analyses.
Counting Unique Values with a Dictionary
Another method to count unique values in a NumPy array is by using a dictionary. This approach involves iterating through the array and counting each element manually. While it requires more code than using numpy.unique()
, it provides a clear understanding of how counting works under the hood.
import numpy as np
array = np.array([1, 2, 2, 3, 4, 4, 4, 5])
counts_dict = {}
for value in array:
if value in counts_dict:
counts_dict[value] += 1
else:
counts_dict[value] = 1
print("Counts using dictionary:", counts_dict)
Output:
Counts using dictionary: {1: 1, 2: 2, 3: 1, 4: 3, 5: 1}
In this code, we first create an empty dictionary called counts_dict
. As we iterate through each element in the NumPy array, we check if the value already exists in the dictionary. If it does, we increment its count; if not, we initialize its count to 1. This method gives us a clear dictionary mapping each unique value to its count, making it easy to understand how many times each value appears in the original array. Although this method is less concise than using numpy.unique()
, it is a valuable approach for those who prefer more control over the counting process.
Using Pandas for Counting Unique Values
For those who are familiar with the Pandas library, you can also leverage its capabilities to count unique values in a NumPy array. Pandas offers a convenient method called value_counts()
that can be applied to a Series object, which can be derived from a NumPy array.
import numpy as np
import pandas as pd
array = np.array([1, 2, 2, 3, 4, 4, 4, 5])
series = pd.Series(array)
counts = series.value_counts()
print("Counts using Pandas:", counts)
Output:
Counts using Pandas: 4 3
2 2
1 1
3 1
5 1
dtype: int64
In this example, we first convert the NumPy array into a Pandas Series. Then, by calling the value_counts()
method on the Series, we get a count of each unique value in descending order. This method not only provides the counts but also sorts them, making it easy to see which values are the most frequent. Using Pandas is particularly advantageous when dealing with larger datasets or when you need additional functionalities, such as data manipulation or visualization.
Conclusion
Counting unique values in a NumPy array is a fundamental task in data analysis. With functions like numpy.unique()
, and methods using dictionaries or Pandas, you have various options to achieve this. Each method has its advantages, whether you prefer the simplicity of NumPy, the manual control of dictionaries, or the powerful features of Pandas. By mastering these techniques, you can enhance your data analysis skills and make your Python programming more effective. So, the next time you encounter a dataset, you’ll know exactly how to count those unique values like a pro!
FAQ
-
What is numpy.unique() used for?
numpy.unique() is used to find unique values in an array and can also count their occurrences. -
Can I use numpy.unique() with multi-dimensional arrays?
Yes, numpy.unique() can handle multi-dimensional arrays, but it will flatten the array by default. -
How does the dictionary method compare to numpy.unique()?
The dictionary method provides more control and understanding of counting but is less concise than numpy.unique(). -
Is it better to use Pandas for counting unique values?
It depends on your needs. Pandas offers more functionality and is better for larger datasets, while NumPy is simpler for quick tasks. -
Can I use numpy.unique() on strings?
Yes, numpy.unique() can also be used to count unique string values in an array.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn