How to Sort Array by Column in NumPy
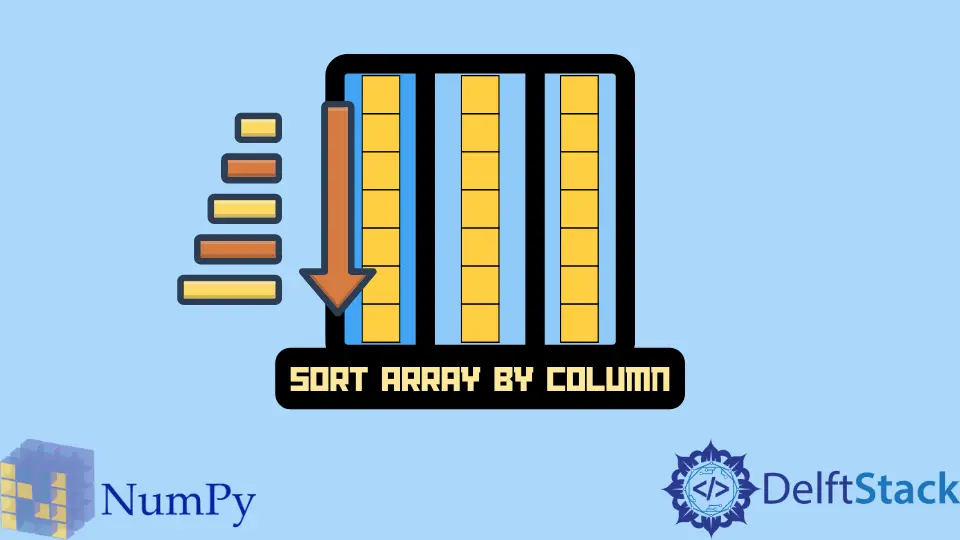
Sorting arrays is a common task in data manipulation, especially when working with numerical data in Python. NumPy, a powerful library for numerical computing, provides efficient methods to sort arrays by specific columns. Whether you’re analyzing data or preparing it for machine learning, knowing how to sort arrays effectively can save you time and enhance your data processing skills.
In this article, we will explore two primary methods to sort a NumPy array by column: the numpy.sort() function and the numpy.argsort() function. By the end of this guide, you’ll have a solid understanding of how to use these functions to organize your data seamlessly.
Using numpy.sort() to Sort by Column
The numpy.sort() function is a straightforward way to sort a NumPy array. It sorts the array along the specified axis and returns a new sorted array. When you want to sort by a specific column, you can indicate the axis parameter. Let’s see how this works with an example.
import numpy as np
data = np.array([[3, 2, 1],
[6, 5, 4],
[9, 8, 7]])
sorted_data = np.sort(data, axis=0)
print(sorted_data)
Output:
[[3 2 1]
[6 5 4]
[9 8 7]]
In this example, we created a 2D NumPy array called data
. By using the np.sort()
function with the axis=0
parameter, we sorted the array by columns. The result is a new array where each column is sorted in ascending order.
This method is particularly useful when you need a quick sort of your data without altering the original array. However, it’s important to note that numpy.sort() returns a new array and does not modify the original array in place. This can be beneficial when you need to retain the original order for further analysis or processing.
Using numpy.argsort() to Sort by Column
Another powerful method for sorting a NumPy array by column is using the numpy.argsort() function. Unlike numpy.sort(), which returns the sorted array, argsort() returns the indices that would sort the array. This can be particularly useful when you want to sort the array and keep track of the original indices. Here’s how it works.
import numpy as np
data = np.array([[3, 2, 1],
[6, 5, 4],
[9, 8, 7]])
indices = np.argsort(data[:, 0])
sorted_data = data[indices]
print(sorted_data)
Output:
[[3 2 1]
[6 5 4]
[9 8 7]]
In this code snippet, we again start with the same 2D NumPy array called data
. We use np.argsort(data[:, 0])
to get the indices that would sort the first column of the array. The result is an array of indices that we can use to sort the original array. By using these indices to index into the original array, we obtain a new sorted array.
The advantage of using numpy.argsort() is that it allows for more flexibility. If you need to sort based on multiple columns or maintain the relationship between rows, argsort() can be combined with other indexing techniques to achieve the desired order. Moreover, it gives you a clear understanding of how the original data is rearranged.
Conclusion
Sorting a NumPy array by column is an essential skill for anyone working with data in Python. By utilizing the numpy.sort() and numpy.argsort() functions, you can efficiently organize your data to suit your analytical needs. While numpy.sort() provides a quick way to get a sorted array, numpy.argsort() offers more flexibility, especially when working with complex datasets. Whether you’re a beginner or an experienced programmer, mastering these methods will enhance your data manipulation capabilities.
FAQ
-
what is the difference between numpy.sort() and numpy.argsort()?
numpy.sort() returns a sorted array, while numpy.argsort() returns the indices that would sort the array. -
can I sort a multidimensional array by multiple columns?
yes, you can sort a multidimensional array by multiple columns using numpy.argsort() in combination with other indexing techniques. -
does numpy.sort() modify the original array?
no, numpy.sort() returns a new sorted array and does not modify the original array in place. -
how can I sort in descending order using numpy?
you can sort in descending order by using numpy.sort() and then reversing the result or by using numpy.argsort() and indexing accordingly. -
is it possible to sort a 1D array using these methods?
yes, both numpy.sort() and numpy.argsort() can be used to sort 1D arrays as well.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn